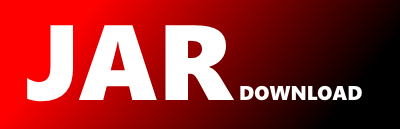
org.jwall.web.audit.AuditEventView Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.audit;
import java.io.InputStream;
import java.nio.ByteBuffer;
import java.rmi.RemoteException;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import org.jwall.audit.EventView;
import org.jwall.web.audit.filter.AuditEventFilter;
/**
*
* This interface defines all method that are accessible by a user for
* investigating events contained in an event store. Typically implementations
* of this interface are acquired by looking up the remote store and querying a
* view instance using a users credentials.
*
*
* The view returned by the store then contains all events that are viewable by
* that specific user.
*
*
* @author Christian Bockermann <[email protected]>
*
*/
public interface AuditEventView extends EventView {
public boolean contains(AuditEvent e);
/**
* This method will return the number of events which match the given filter
* filter
AND the user-specific implicit filter expressions.
*
* @param filter
* The filter to select the events being counted.
* @return The number of events in this view, matching the given filter.
* @throws RemoteException
*/
public Long count(AuditEventFilter filter) throws Exception;
/**
* This method will return the complete audit-event from the storage. The ID
* provided is expected to be the event ID provided by ModSecurity and used
* by ModSecurity within the section separators.
*
* @param id
* @return
* @throws Exception
*/
public AuditEvent getEvent(String id) throws Exception;
public Long delete(String id) throws Exception;
/**
* This method will retrieve the entry with the given id from the index or
* null
if no entry exists with that id.
*
* @param id
* @return
* @throws Exception
*/
public AuditEvent getEventEntry(String id) throws Exception;
public ByteBuffer getEventRaw(String id);
public InputStream getEventAsStream(String id);
/**
* Creates an iterator over all event entries matching the given filter. The
* iterator supports the remove() method, but this does not have an effect
* on the database.
*
* @param filter
* @return
* @throws Exception
*/
public Iterator iterator(AuditEventFilter filter) throws Exception;
public List getLastAlertMessages(int max)
throws Exception;
public Set getVariables();
/**
*
* @return
* @throws Exception
* @deprecated
*/
public Set getTags() throws Exception;
/**
*
* @param id
* @return
* @deprecated
*/
public Set getTagsByEventId(String id);
/**
*
* @param user
* @return
* @deprecated
*/
public Set getTagsByUser(String user);
/**
*
* @param filter
* @param offset
* @param num
* @deprecated
* @return
*/
public List list(AuditEventFilter filter, int offset, int num);
/**
*
* @param filter
* @param order
* @param offset
* @param num
* @return
* @deprecated
*/
public List list(AuditEventFilter filter, List order,
int offset, int num);
/**
*
* @param eventId
* @param user
* @param tags
* @deprecated
*/
public void tag(String eventId, String user, List tags);
/**
*
* @param filter
* @param user
* @param tags
* @deprecated
*/
public void tag(AuditEventFilter filter, String user, List tags);
/**
* @param eventId
* @param user
* @param tags
* @deprecated
*/
public void untag(String eventId, String user, List tags);
/**
*
* @param filter
* @param user
* @param tags
* @deprecated use untag(FilterExpression,..) instead
*/
public void untag(AuditEventFilter filter, String user, List tags);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy