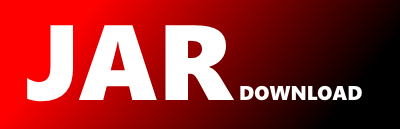
org.jwall.web.audit.DefaultAuditEventFactory Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.audit;
import java.io.BufferedReader;
import java.io.File;
import java.io.StringReader;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.jwall.web.audit.io.ParseException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
*
* This is the default implementation of the event factory. It will not provide any recycling
* or memory optimization, but simply create events in the way they have been created before.
*
*
* @author Christian Bockermann <[email protected]>
*
*/
public class DefaultAuditEventFactory
implements AuditEventFactory
{
static Logger log = LoggerFactory.getLogger( "DefaultAuditEventFactory" );
/** a count of all events created by this factory */
protected static Long evtCount = Long.valueOf( 0L );
private static AuditEventFactory sharedInstance = null;
private final static String RULE_FILE_PREFIX = "[file ";
private final static String RULE_LINE_PREFIX = "[line ";
private final static String RULE_ID_PREFIX = "[id ";
private final static String RULE_MSG_PREFIX = "[msg ";
private final static String RULE_SEVERITY_PREFIX = "[severity ";
private final static String RULE_TAG_PREFIX = "[tag ";
private final static String extract[] = new String[]{
RULE_FILE_PREFIX, ModSecurity.RULE_FILE,
RULE_LINE_PREFIX, ModSecurity.RULE_LINE,
RULE_ID_PREFIX, ModSecurity.RULE_ID,
RULE_MSG_PREFIX, ModSecurity.RULE_MSG,
RULE_TAG_PREFIX, ModSecurity.RULE_TAG,
RULE_SEVERITY_PREFIX, ModSecurity.RULE_SEV
};
/**
* The private constructor for this class. There should only be one
* instance of this class available.
*/
public DefaultAuditEventFactory(){
evtCount = 0L;
}
/**
* This method will return the shared instance of this factory.
*
* @return The shared factory instance.
*/
public static AuditEventFactory getInstance(){
if( sharedInstance == null ){
sharedInstance = new DefaultAuditEventFactory();
}
return sharedInstance;
}
public AuditEvent createAuditEvent(String[] sectionData, AuditEventType type)
throws ParseException
{
AuditEvent evt = new ModSecurityAuditEvent( sectionData, type );
if( evt != null ){
synchronized( evtCount) {
evtCount++;
}
}
return evt;
}
/* (non-Javadoc)
* @see org.jwall.web.audit.AuditEventFactory#createAuditEvent(java.lang.String, java.lang.String[], java.io.File, long, long)
*/
public AuditEvent createAuditEvent(String id, String[] data, File inputFile,
long offset, long size, AuditEventType type ) throws ParseException
{
ModSecurityAuditEvent evt = new ModSecurityAuditEvent(id, data, inputFile, offset, size, type );
if( evt != null ){
synchronized( evtCount) {
evtCount++;
}
}
if( inputFile != null )
evt.set( AuditEvent.FILE, inputFile.getAbsolutePath() );
evt.set( AuditEvent.FILE_OFFSET, Long.toString( offset ) );
evt.set( AuditEvent.SIZE, Long.toString( size ) );
return evt;
}
public static Map> parseAuditTrailer( Map> cols, String trailer ) throws ParseException {
//Map> cols = new HashMap>();
if( trailer == null || "".equals( trailer.trim() ) )
return cols;
try {
// now we scan the audit-trailer, extracting rule-ids, msgs, tags and the like
//
BufferedReader r = new BufferedReader( new StringReader( trailer ) );
String line = r.readLine();
while( line != null ){
System.out.println( "Processing tailer-line: " + line );
int start = line.indexOf( "[" ); // anything we extract starts with a bracket
// now we can limit the search on everything after the first bracket
if( start >= 0 ){
String val = "";
for( int i = 0; start >= 0 && i + 2 < extract.length; i += 2 ){
line = line.substring( start );
if( line.startsWith( extract[i] ) ){
int end = line.indexOf( "]" );
val = line.substring( extract[i].length(), end ).trim();
if( val.startsWith("\"") )
val = val.substring(1);
if( val.endsWith( "\"" ) )
val = val.substring( 0, val.length() - 1 );
addValue( cols, extract[i+1], val );
start = line.indexOf( "[", end );
} else {
start++;
}
}
}
line = r.readLine();
}
} catch ( Exception e ) {
e.printStackTrace();
throw new ParseException("Error while parsing the audit-trailer: " + e.getMessage() );
}
return cols;
}
public static void addValue( Map> cols, String col, String value ){
log.info( "Adding value \"" + value + "\" to variable \"" + col + "\"" );
List collection = cols.get( col );
if( collection == null ){
collection = new LinkedList();
cols.put( col, collection );
}
collection.add( value );
}
public static Long getEventCount(){
return evtCount;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy