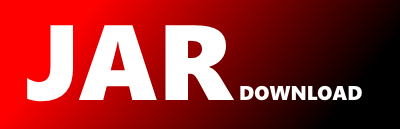
org.jwall.web.audit.IronBeeEventMessage Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.audit;
import java.io.Serializable;
import java.lang.reflect.Array;
import java.util.ArrayList;
import java.util.Date;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
public class IronBeeEventMessage implements AuditEventMessage {
public final static String EVENT_ID = AuditEvent.EVENT_ID;
public final static String RULE_ID = ModSecurity.RULE_ID;
public final static String TAGS = AuditEvent.TAGS;
public final static String FIELDS = "FIELDS";
public final static String CONFIDENCE = "CONFIDENCE";
public final static String REC_ACTION = "REC_ACTION";
final Map map = new LinkedHashMap();
@Override
public String getTxId() {
return get(ModSecurity.TX_ID) + "";
}
@Override
public Date getDate() {
return null;
}
@Override
public String getFile() {
return "";
}
@Override
public Integer getLine() {
return -1;
}
@Override
public Integer getSeverity() {
try {
return new Integer(get("SEVERITY") + "");
} catch (Exception e) {
return -1;
}
}
@Override
public String getText() {
return get("MSG") + "";
}
@Override
public List getRuleTags() {
ArrayList list = new ArrayList();
try {
String[] tags = (String[]) get("TAGS");
if (tags == null)
return list;
for (String tag : tags) {
list.add(tag);
}
} catch (Exception e) {
e.printStackTrace();
}
return list;
}
@Override
public String getRuleMsg() {
return getText();
}
@Override
public String getRuleId() {
return get(ModSecurity.RULE_ID) + "";
}
@Override
public String getRuleData() {
return get(ModSecurity.RULE_DATA) + "";
}
public void set(String key, Serializable value) {
if (value == null) {
map.remove(map(key));
return;
}
map.put(map(key), value);
}
public Serializable get(String key) {
return map.get(map(key));
}
private String map(String key) {
return key.toUpperCase().replaceAll("-", "_");
}
public String toString() {
StringBuffer s = new StringBuffer();
Iterator it = map.keySet().iterator();
while (it.hasNext()) {
String key = it.next();
s.append(key);
s.append("=");
Serializable val = map.get(key);
if (val.getClass().isArray()) {
s.append("[");
int len = Array.getLength(val);
for (int i = 0; i < len; i++) {
Object obj = Array.get(val, i);
s.append(obj);
if (i + 1 < len)
s.append(",");
}
s.append("]");
} else {
s.append(val.toString());
}
if (it.hasNext())
s.append(", ");
}
return s.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy