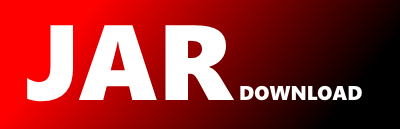
org.jwall.web.audit.ModSecurityEventMessage Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.audit;
import java.util.ArrayList;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
public class ModSecurityEventMessage implements AuditEventMessage {
/** The unique class ID */
private static final long serialVersionUID = -2274796492823296560L;
private static Long instanceCount = 0L;
String txId = "";
String text = null;
String file = null;
Integer line = null;
String ruleMsg = null;
String ruleId = null;
String ruleData = null;
List ruleTags = null;
Integer severity = ModSecurity.SEVERITY_NOT_SET;
Date date = new Date();
public String toString() {
StringBuilder b = new StringBuilder("Message:");
if (text != null) {
b.append(" ");
b.append(text);
}
if (file != null) {
b.append(" [file \"" + file + "\"]");
}
if (line != null) {
b.append(" [line \"" + line + "\"]");
}
if (ruleId != null) {
b.append(" [id \"" + ruleId + "\"]");
}
if (ruleMsg != null) {
b.append(" [msg \"" + ruleMsg + "\"]");
}
if (this.severity != null && severity >= 0
&& severity < ModSecurity.SEVERITIES.length) {
b.append(" [severity \"" + ModSecurity.SEVERITIES[severity] + "\"]");
}
if (ruleData != null) {
b.append(" [data \"" + ruleData + "\"]");
}
if (ruleTags != null && !ruleTags.isEmpty()) {
Iterator it = ruleTags.iterator();
while (it.hasNext()) {
b.append(" [tag \"" + it.next() + "\"]");
}
}
return b.toString();
}
public ModSecurityEventMessage() {
synchronized (instanceCount) {
instanceCount++;
}
}
/**
* @return the eventId
*/
public String getTxId() {
return txId;
}
/**
* @param eventId
* the eventId to set
*/
public void setTxId(String eventId) {
this.txId = eventId;
}
/**
* @return the date
*/
public Date getDate() {
return date;
}
/**
* @param date
* the date to set
*/
public void setDate(Date date) {
this.date = date;
}
/**
* @return the text
*/
public String getText() {
return text;
}
/**
* @param text
* the text to set
*/
public void setText(String text) {
this.text = text;
}
/**
* @return the file
*/
public String getFile() {
return file;
}
/**
* @param file
* the file to set
*/
public void setFile(String file) {
this.file = file;
}
/**
* @return the line
*/
public Integer getLine() {
return line;
}
/**
* @param line
* the line to set
*/
public void setLine(Integer line) {
this.line = line;
}
/**
* @return the ruleMsg
*/
public String getRuleMsg() {
return ruleMsg;
}
/**
* @param ruleMsg
* the ruleMsg to set
*/
public void setRuleMsg(String ruleMsg) {
this.ruleMsg = ruleMsg;
}
/**
* @return the ruleId
*/
public String getRuleId() {
return ruleId;
}
/**
* @param ruleId
* the ruleId to set
*/
public void setRuleId(String ruleId) {
this.ruleId = ruleId;
}
/**
* @return the ruleData
*/
public String getRuleData() {
return ruleData;
}
/**
* @param ruleData
* the ruleData to set
*/
public void setRuleData(String ruleData) {
this.ruleData = ruleData;
}
/**
* @return the ruleTags
*/
public List getRuleTags() {
return ruleTags;
}
/**
* @param ruleTags
* the ruleTags to set
*/
public void setRuleTags(List ruleTags) {
this.ruleTags = ruleTags;
}
public Integer getSeverity() {
return severity;
}
public void setSeverity(Integer sev) {
severity = sev;
}
public String getTag() {
if (this.ruleTags == null || ruleTags.isEmpty())
return null;
return ruleTags.get(0);
}
public void setTag(String tag) {
if (this.ruleTags == null)
this.ruleTags = new ArrayList();
this.ruleTags.add(tag);
}
public void finalize() {
synchronized (instanceCount) {
instanceCount--;
}
}
public static Long getInstanceCount() {
return instanceCount;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy