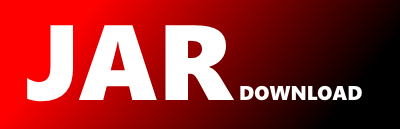
org.jwall.web.audit.io.MessageParser Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.audit.io;
import java.io.BufferedReader;
import java.io.StringReader;
import java.util.LinkedList;
import java.util.List;
import org.jwall.log.io.MParser;
import org.jwall.web.audit.AuditEvent;
import org.jwall.web.audit.AuditEventMessage;
import org.jwall.web.audit.ModSecurity;
import org.jwall.web.audit.ModSecurityEventMessage;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.thoughtworks.xstream.XStream;
/**
*
* This class implements a simple message parser, which reads the audit trailer
* section from a ModSecurity event and creates Message
instances
* from that section.
*
*
* @author Christian Bockermann <[email protected]>
*
*/
public class MessageParser extends MParser {
static Logger log = LoggerFactory.getLogger(MessageParser.class);
static XStream xstream = new XStream();
static {
xstream.processAnnotations(AuditEventMessage.class);
}
/**
*
* @param evt
* @return
*/
public static List parseMessages(AuditEvent evt) {
List msgs = new LinkedList();
try {
if (evt.getSection(ModSecurity.SECTION_AUDIT_TRAILER) == null)
return msgs;
BufferedReader r = new BufferedReader(new StringReader(
evt.getSection(ModSecurity.SECTION_AUDIT_TRAILER)));
String line = r.readLine();
while (line != null) {
if (line.startsWith("Message: ")) {
log.debug("Parsing: {}", line);
ModSecurityEventMessage m = new ModSecurityEventMessage();
String theMesg = line.substring("Message: ".length());
//
// TODO: The following should be enhanced to get the
// extractions done in a 1-pass manner
//
String file = extract("file", theMesg);
m.setFile(file);
if (file != null) {
theMesg = remove("file", theMesg);
}
String lineNr = extract("line", theMesg);
if (lineNr != null) {
try {
m.setLine(Integer.parseInt(lineNr));
} catch (Exception e) {
log.error("Not a line-number: {}", lineNr);
e.printStackTrace();
}
theMesg = remove("line", theMesg);
}
String id = extract("id", theMesg);
if (id != null) {
m.setRuleId(id);
theMesg = remove("id", theMesg);
}
String data = extract("data", theMesg);
if (data != null) {
m.setRuleData(data);
theMesg = remove("data", theMesg);
}
String severity = extract("severity", theMesg);
if (severity != null) {
m.setSeverity(ModSecurity.getSeverity(severity));
theMesg = remove("severity", theMesg);
}
String ruleMsg = extract("msg", theMesg);
if (ruleMsg != null) {
theMesg = remove("msg", theMesg);
m.setRuleMsg(ruleMsg);
}
String tag = extract("tag", theMesg);
while (tag != null) {
theMesg = remove("tag", theMesg);
m.setTag(tag);
tag = extract("tag", theMesg);
}
m.setText(theMesg.trim());
msgs.add(m);
} else
log.debug("Skipping: {}", line);
line = r.readLine();
}
r.close();
} catch (Exception e) {
log.error("Failed to parse messages from event {}: {}",
evt.getEventId(), e.getMessage());
e.printStackTrace();
}
return msgs;
}
public static AuditEventMessage parseMessage(String line) {
if (line.startsWith("Message: ")) {
ModSecurityEventMessage m = new ModSecurityEventMessage();
String theMesg = line.substring("Message: ".length());
//
// TODO: The following should be enhanced to get the extractions
// done in a 1-pass manner
//
String file = extract("file", theMesg);
m.setFile(file);
if (file != null) {
theMesg = remove("file", theMesg);
}
String lineNr = extract("line", theMesg);
if (lineNr != null) {
try {
m.setLine(Integer.parseInt(lineNr));
} catch (Exception e) {
log.error("Not a line-number: {}", lineNr);
e.printStackTrace();
}
theMesg = remove("line", theMesg);
}
String id = extract("id", theMesg);
if (id != null) {
m.setRuleId(id);
theMesg = remove("id", theMesg);
}
String data = extract("data", theMesg);
if (data != null) {
m.setRuleData(data);
theMesg = remove("data", theMesg);
}
String severity = extract("severity", theMesg);
if (severity != null) {
m.setSeverity(ModSecurity.getSeverity(severity));
theMesg = remove("severity", theMesg);
}
String ruleMsg = extract("msg", theMesg);
if (ruleMsg != null) {
theMesg = remove("msg", theMesg);
m.setRuleMsg(ruleMsg);
}
String tag = extract("tag", theMesg);
while (tag != null) {
theMesg = remove("tag", theMesg);
m.setTag(tag);
tag = extract("tag", theMesg);
}
m.setText(theMesg.trim());
return m;
} else
return null;
}
public static String extract(String tag, String line) {
log.debug("trying to extract {} from line {}", tag, line);
try {
String start = "[" + tag + " \"";
String end = "\"]";
int fileIndex = line.indexOf(start);
if (fileIndex > 0) {
int fileEnd = line.indexOf(end, fileIndex);
String data = "";
//
// this is a fix to handle a ModSecurity limitation of the
// maximum
// length
// of Message lines. In case we hit the end of the line without
// having found
// a proper end-tag, we treat the rest of the line as value
//
if (fileEnd < 0)
data = line.substring(fileIndex + start.length());
else
data = line.substring(fileIndex + start.length(), fileEnd);
return data;
}
} catch (Exception e) {
log.error("Failed to extract {}: {}", tag, e.getMessage());
}
return null;
}
public static String remove(String tag, String line) {
log.debug("trying to remove {} from line {}", tag, line);
String start = "[" + tag + " \"";
String end = "\"]";
try {
int fileIndex = line.indexOf(start);
if (fileIndex > 0) {
int fileEnd = line.indexOf(end, fileIndex);
//
// this is a fix to handle a ModSecurity limitation of the
// maximum
// length
// of Message lines. In case we hit the end of the line without
// having found
// a proper end-tag, we treat the rest of the line as value
//
if (fileEnd < 0)
return "";
line = line.substring(0, fileIndex)
+ line.substring(fileEnd + end.length());
return line;
}
} catch (Exception e) {
log.error("Failed to extract {}: {}", tag, e.getMessage());
}
return line;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy