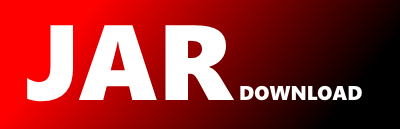
org.jwall.web.audit.net.SyslogReceiverThread Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.audit.net;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.ArrayList;
import java.util.List;
import org.jwall.web.audit.AuditEventListener;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
*
* This class is the central syslog-server thread. It waits for incoming connections
* and creates a new connection handler for each incoming call.
*
*
* @author Christian Bockermann <[email protected]>
*
*/
public class SyslogReceiverThread
extends Thread
{
/* The logger for this class */
static Logger log = LoggerFactory.getLogger( SyslogReceiverThread.class );
/* The server socket for incoming TCP connections */
ServerSocket socket;
/* The list of handlers, currently processing connections */
List handlers = new ArrayList();
AuditEventListener listener = null;
SocketAuthenticator authenticator;
public SyslogReceiverThread( AuditEventListener l, Integer port ) throws Exception {
listener = l;
socket = new ServerSocket( port, 100 );
log.info( "Listening for connections on tcp-port {}", port );
}
public SyslogReceiverThread( AuditEventListener l, InetAddress addr, Integer port ) throws Exception {
listener = l;
socket = new ServerSocket( port, 100, addr );
log.info( "Listening for connections on " + addr.getHostAddress() + ":" + port );
}
public void setSocketAuthenticator( SocketAuthenticator auth ){
this.authenticator = auth;
}
public SocketAuthenticator getSocketAuthenticator(){
return this.authenticator;
}
protected String authenticate( Socket socket ){
log.debug( "Authenticating connection..." );
if( authenticator != null )
return authenticator.authenticate( socket );
return null;
}
public void run(){
while( ! socket.isClosed() ){
try {
String sensor = null;
Socket connection = socket.accept();
log.info( "incoming connection from {}:{}", connection.getInetAddress().getHostAddress(), connection.getPort() );
sensor = authenticate( connection );
if( sensor != null ){
log.info( "Accepting connection from address {} for sensor '{}'", connection.getInetAddress(), sensor );
AuditEventStreamHandler handler = createHandler( connection );
handler.start();
} else {
log.warn( "Denying TCP connection from {} - no sensor found for that remote address!", connection.getInetAddress() );
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
private AuditEventStreamHandler createHandler( Socket connection ) throws Exception {
AuditEventStreamHandler handler = new AuditEventStreamHandler( this, connection, false, true );
if( listener != null )
handler.addListener( listener );
synchronized( handlers ){
handlers.add( handler );
}
return handler;
}
protected void handlerFinished( AuditEventStreamHandler handler ){
synchronized( handlers ){
handlers.add( handler );
}
}
public void shutdown(){
try {
socket.close();
} catch (Exception e) {
e.printStackTrace();
}
log.info( "Sending shutdown signal to connection handlers..." );
for( AuditEventStreamHandler handler : handlers )
handler.close();
for( AuditEventStreamHandler handler : handlers ){
log.info( "Waiting for handler {} to stop", handler );
try {
handler.join();
} catch (Exception e) {
log.error( "Failed to wait for handler: {}", e );
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy