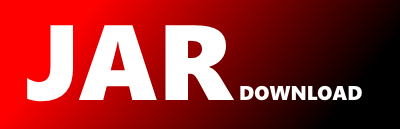
org.jwall.web.audit.session.HeuristicSessionTracker Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.audit.session;
import java.util.HashMap;
import java.util.LinkedList;
import org.jwall.web.audit.AuditEvent;
import org.jwall.web.audit.ModSecurity;
/**
*
* This heuristic session-tracker implements the session-algorithm used by
* most popular web-statistic tools, e.g. WUM.
*
* @author Christian Bockermann <[email protected]>
*
*/
public class HeuristicSessionTracker
extends AbstractSessionTracker
{
/**
* This constructor creates a session-tracker based on heuristics.
* Key to the session is simply the ip-address of the requests.
*
* @param sessionTime
*/
public HeuristicSessionTracker(long sessionTime){
super( sessionTime );
sessionTimeOut = sessionTime;
//
// this hash stores lists of sessions by ip-address, user-agent and start-date
// when one session ends (times out), another is created an enqueued to the
// list identified by (ip-address,user-agent)
//
activeSessions = new HashMap();
//
// we store a list of all timed-out sessions
//
timedOutSessions = new LinkedList();
}
/**
*
* Since this tracker simply relies on the remote address as session key, this
* method will just return the event's sender-address.
*
* @param evt The AuditEvent to extract a key-id from.
* @return A string with the ip-address of the event to be used as a session-id.
*
*/
public String extractKey( AuditEvent evt ){
try {
StringBuffer msg = new StringBuffer();
String addr = evt.get( ModSecurity.REMOTE_ADDR );
msg.append( addr );
String ua = evt.get( ModSecurity.REQUEST_HEADERS + ":" + HttpProtocol.REQUEST_HEADER_USER_AGENT );
if( ua != null )
msg.append( ua );
return msg.toString();
} catch (Exception e ){
return evt.get( ModSecurity.REMOTE_ADDR );
}
}
public String toString(){
return "HeuristicSessionTracker["+this.sessionTimeOut+"]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy