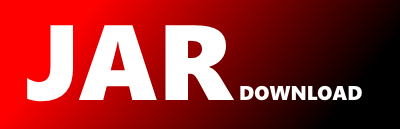
org.jwall.web.audit.session.Session Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.audit.session;
import java.net.InetAddress;
import java.util.Date;
import java.util.Hashtable;
import java.util.Set;
import org.jwall.web.audit.AuditEvent;
import org.jwall.web.audit.ModSecurity;
/**
*
* This class implements a data storage of some key-value-pairs, all associated
* with a unique session-id.
*
* @author Christian Bockermann <[email protected]>
*
*/
public class Session
implements Comparable, SessionContext
{
public final static int COOKIE_BASED = 1;
public final static int PARAMETER_BASED = 2;
public final static int HEURISTIC_BASED = 3;
/* This sessions type, e.g. parameter-based, cookie-based or heuristic */
private int type;
/* The unique session-id */
private String id;
/* The date, this session has been established */
private Date firstAccessed;
/* The date of the last request of this session */
private Date lastAccessed;
/* The source-ip, which is associated with this session */
private InetAddress address;
/** the session-score which shows how dangerous this session is... */
private double score = 0.0d;
Hashtable sessionVariables = new Hashtable();
/**
* Creates a new session with the given session-identifier and the first
* event of the session being evt
. Start time of the session
* is the date for evt
.
*
* @param type The type which denotes the kind of session-tracker by which
* the session is created.
*
*/
public Session(int type, String id, AuditEvent evt){
this.id = id;
this.type = type;
if( evt != null ){
try {
address = InetAddress.getByName(evt.get( ModSecurity.REMOTE_ADDR ));
} catch (Exception e) {
e.printStackTrace();
}
firstAccessed = evt.getDate();
lastAccessed = firstAccessed;
//evt.setRelativeSessionTime(0);
}
}
/**
* Returns wether this session context is tracked based on a Session-Id in
* Cookies, Parameters or just heuristically using ip, time and user-agent.
*
* @return The type of session-tracker that created this ession.
*/
public int getType(){
return this.type;
}
/**
*
* @param addr
*/
public void setInetAddress(InetAddress addr){
address = addr;
}
/**
*
* @return The ip-address that is associated with this session.
*/
public InetAddress getInetAddress(){
return address;
}
public Date lastAccessed(){
return new Date( lastAccessed.getTime() );
}
public Date firstAccessed(){
return new Date( firstAccessed.getTime() );
}
public String getId(){
return this.id;
}
/**
*
*/
public boolean equals(Object o){
if(! (o instanceof Session))
return false;
Session other = (Session) o;
return id.equals(other.id);
}
/**
*
*
* @param e
*/
public void addEvent(AuditEvent e){
if(e.getDate().after( this.lastAccessed() ))
this.lastAccessed = e.getDate();
}
/**
* This compares the session to s
. Basically this is just a comparison
* of the session-identifiers and results in a lexikographical order of sessions based
* on ther session-ids.
*
* @param s
* @return 0, if this session equals s
, or +1/-1 if it is ordered before/after s
.
*/
public int compareTo(Session s){
return id.compareTo(s.id);
}
public String toString(){
StringBuffer s = new StringBuffer();
s.append( this.getId() +" ~> ");
/*
Iterator it = events.iterator();
while(it.hasNext()){
AuditEvent evt = it.next();
long rel = evt.getDate().getTime() - firstAccessed().getTime();
s.append( evt.get( ModSecurity.REQUEST_METHOD )+":"+evt.get( ModSecurity.REQUEST_FILENAME )+" ("+rel+")");
if( it.hasNext() )
s.append(", ");
}
*/
return s.toString();
}
public double getScore(){
return score;
}
public void setScore(double d){
score = d;
}
//
//
//
/* (non-Javadoc)
* @see org.jwall.core.http.SessionContext#getVariable(java.lang.String)
*/
public String getVariable(String name)
{
if( name != null && sessionVariables.containsKey( name ) )
return sessionVariables.get( name );
return null;
}
/* (non-Javadoc)
* @see org.jwall.core.http.SessionContext#getVariableNames()
*/
public Set getVariableNames()
{
return sessionVariables.keySet();
}
/* (non-Javadoc)
* @see org.jwall.core.http.SessionContext#increment(java.lang.String, int)
*/
public void increment(String var, int count)
{
String val = getVariable( var );
if( val == null || ! val.matches("\\d+") )
return;
Integer d = new Integer(val);
d++;
sessionVariables.put( var, d.toString() );
}
/* (non-Javadoc)
* @see org.jwall.core.http.SessionContext#setVariable(java.lang.String, java.lang.String, java.util.Date)
*/
public void setVariable(String name, String value, Date expires)
{
sessionVariables.put( name, value);
}
/*
* @param name
*/
public void removeVariable( String name ){
if( sessionVariables.containsKey( name ) )
sessionVariables.remove( name );
}
public int hashCode(){
return id.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy