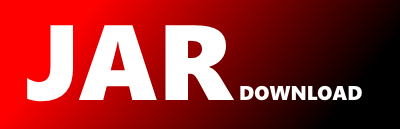
org.jwall.web.http.HttpRequest Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2014 Christian Bockermann
*
* This file is part of the web-audit library.
*
* web-audit library is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* The web-audit library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.jwall.web.http;
import java.net.URL;
public class HttpRequest extends HttpMessage {
public final static String ACCEPT = "Accept";
public final static String ACCEPT_CHARSET = "Accept-Charset";
public final static String ACCEPT_ENCODING = "Accept-Encoding";
public final static String ACCEPT_LANGUAGE = "Accept-Language";
public final static String AUTHORIZATION = "Authorization";
public final static String EXPECT = "Expect";
public final static String FROM = "From";
public final static String HOST = "Host";
public final static String IF_MATCH = "If-Match";
public final static String IF_MODIFIED_SINCE = "If-Modified-Since";
public final static String IF_NONE_MATCH = "If-None-Match";
public final static String IF_RANGE = "If-Range";
public final static String IF_UNMODIFIED_SINCE = "If-Unmodified-Since";
public final static String MAX_FORWARDS = "Max-Forwards";
public final static String PROXY_AUTHORIZATION = "Proxy-Authorization";
public final static String RANGE = "Range";
public final static String REFERER = "Referer";
public final static String TE = "TE";
public final static String USER_AGENT = "User-Agent";
String method = "";
String uri = "";
URL url = null;
/**
*
* This will create a new http-request from the given String-array. The array
* is assumed to contain the request-header in reqData[0]
and
* optionally the request-body in reqData[1]
.
*
*
* @param reqHeader
* @param reqBody
* @throws ProtocolException
*/
public HttpRequest( String reqHeader, byte[] reqBody )
throws ProtocolException
{
super( new HttpHeader(reqHeader), reqBody );
parseStartLine( header.startLine );
}
public HttpRequest( HttpHeader h, byte[] reqBody)
throws ProtocolException
{
super( h, reqBody);
parseStartLine( header.startLine );
}
public HttpRequest( String reqHeader )
throws Exception
{
this( reqHeader, new byte[0] );
}
protected void parseStartLine( String line )
throws MessageFormatException
{
try {
String[] tok = line.split( HttpHeader.SP );
method = tok[0];
uri = tok[1];
version = tok[2];
} catch ( Exception e ){
throw new MessageFormatException("Invalid request-line: " + line );
}
try {
String host = getHeader( HttpRequest.HOST );
String proto = "http";
String sep = "/";
if( uri.startsWith("/") )
sep = "";
url = new URL( proto + "://" + host + sep + uri );
} catch ( Exception e ){
throw new MessageFormatException("Error while constructing the URL: "+e.getLocalizedMessage());
}
}
public String getMethod(){
return method;
}
public String getURI(){
return uri;
}
public URL getURL(){
return url;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy