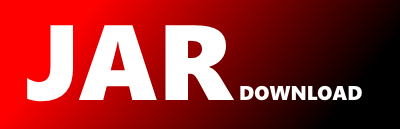
stream.io.multi.SequentialMultiStream Maven / Gradle / Ivy
package stream.io.multi;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import stream.Data;
import stream.io.Stream;
public class SequentialMultiStream extends AbstractMultiDataStream {
static Logger log = LoggerFactory.getLogger(SequentialMultiStream.class);
String sourceKey = "@source";
int index = 0;
public String getSourceKey() {
return sourceKey;
}
public void setSourceKey(String sourceKey) {
this.sourceKey = sourceKey;
}
/**
* @see stream.io.multi.AbstractMultiDataStream#readNext(stream.Data,
* java.util.Map)
*/
@Override
protected Data readNext(Data item, Map streams)
throws Exception {
Data data = null;
while ((data == null && index < additionOrder.size())) {
try {
String current = additionOrder.get(index);
log.debug("Current stream is: {}", current);
Stream currentStream = streams.get(current);
data = currentStream.read();
if (data != null) {
data.put(sourceKey, current);
log.debug(" returning data {}", data);
return data;
}
log.debug("Stream {} ended, switching to next stream", current);
index++;
if (index >= additionOrder.size()) {
log.debug("No more streams to read from!");
return null;
}
} catch (Exception e) {
log.error("Error: {}", e.getMessage());
if (log.isTraceEnabled())
e.printStackTrace();
}
}
log.debug("No more streams to read from - all seem to have reached their end.");
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy