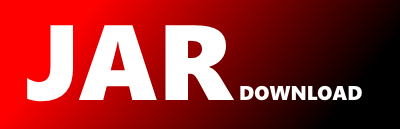
stream.parser.ParseString Maven / Gradle / Ivy
/**
*
*/
package stream.parser;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import stream.AbstractProcessor;
import stream.Data;
import stream.ProcessContext;
import stream.annotations.Parameter;
import stream.util.parser.Parser;
import stream.util.parser.ParserGenerator;
/**
* @author Christian Bockermann <[email protected]>
*
*/
public class ParseString extends AbstractProcessor {
static Logger log = LoggerFactory.getLogger(ParseString.class);
String key = null;
String format = null;
Parser
© 2015 - 2025 Weber Informatics LLC | Privacy Policy