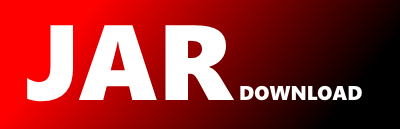
stream.Keys Maven / Gradle / Ivy
The newest version!
/**
*
*/
package stream;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import stream.data.DataFactory;
import stream.util.WildcardPattern;
/**
*
* The Keys
class represents a set of key strings, which may
* include wildcard patterns using the wildcard ?
and
* *
. This allows for quickly specifying a list of keys from a data
* item.
*
*
* @author Christian Bockermann
*
*/
public final class Keys implements Serializable {
/** The unique class ID */
private static final long serialVersionUID = 1301122628686584473L;
static Logger log = LoggerFactory.getLogger(Keys.class);
final String[] keyValues;
/**
* This constructor will split the argument string by occurences of the
* '
character and create a Keys
instance of the
* resulting substrings.
*
* @param kString
*/
public Keys(String kString) {
this(kString.split(","));
}
/**
* This constructor creates an instance of Keys
with the given
* list of key names. Each key name may contain wildcards.
*
* @param ks
*/
protected Keys(String... ks) {
final ArrayList keyValues = new ArrayList();
for (String k : ks) {
if (k.trim().isEmpty()) {
continue;
} else {
keyValues.add(k.trim());
}
}
this.keyValues = keyValues.toArray(new String[keyValues.size()]);
}
public Keys(Collection ks) {
final ArrayList keyValues = new ArrayList();
for (String k : ks) {
if (k.trim().isEmpty()) {
continue;
} else {
keyValues.add(k.trim());
}
}
this.keyValues = keyValues.toArray(new String[keyValues.size()]);
}
public Set select(Data item) {
if (item == null) {
return new HashSet();
}
return select(item.keySet());
}
public Set select(Collection names) {
return select(names, keyValues);
}
public final String toString() {
StringBuffer s = new StringBuffer();
for (int i = 0; i < keyValues.length; i++) {
if (keyValues[i] != null && !keyValues[i].trim().isEmpty()) {
if (s.length() > 0) {
s.append(",");
}
s.append(keyValues[i].trim());
}
}
return s.toString();
}
public Data refine(Data item) {
Data out = DataFactory.create();
for (String key : this.select(item.keySet())) {
out.put(key, item.get(key));
}
return out;
}
public static String joinValues(Data item, String[] keys, String glue) {
StringBuffer s = new StringBuffer();
for (String key : select(item, keys)) {
Serializable value = item.get(key);
if (value != null) {
if (s.length() > 0) {
s.append(glue);
}
s.append(value.toString());
}
}
return s.toString();
}
public static Set select(Data item, String filter) {
if (filter == null || item == null)
return new LinkedHashSet();
return select(item, filter.split(","));
}
public static Set select(Data item, String[] keys) {
Set selected = new LinkedHashSet();
if (item == null)
return selected;
return select(item.keySet(), keys);
}
public static Set select(Collection ks, String[] keys) {
Set selected = new LinkedHashSet();
if (ks == null)
return selected;
if (keys == null) {
selected.addAll(ks);
return selected;
}
for (String key : ks) {
if (isSelected(key, keys))
selected.add(key);
}
return selected;
}
public static boolean isSelected(String value, String[] keys) {
if (keys == null || keys.length == 0)
return false;
boolean included = false;
for (String k : keys) {
if (k.startsWith("!")) {
k = k.substring(1);
if (included && WildcardPattern.matches(k, value)) {
included = false;
log.debug(
"Removing '{}' from selection due to pattern '!{}'",
value, k);
}
} else {
if (!included && WildcardPattern.matches(k, value)) {
included = true;
log.debug("Adding '{}' to selection due to pattern '{}'",
value, k);
}
}
}
return included;
}
/**
* Get the strings which were used to build this object.
* @return the keys
*/
public String[] getKeyValues() {
return keyValues;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy