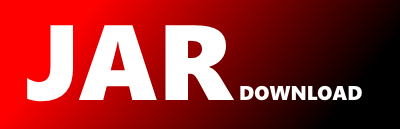
stream.data.NormalizeVector Maven / Gradle / Ivy
/**
*
*/
package stream.data;
import java.io.Serializable;
import java.util.Set;
import stream.Data;
import stream.Processor;
import stream.learner.LearnerUtils;
import stream.util.KeyFilter;
/**
* @author chris
*
*/
public class NormalizeVector implements Processor {
String[] keys;
/**
* @see stream.Processor#process(stream.data.Data)
*/
@Override
public Data process(Data input) {
Double sum = 0.0;
Set ks = KeyFilter.select(input, keys);
for (String key : ks) {
if (LearnerUtils.isAnnotation(key))
continue;
Serializable value = input.get(key);
if (value instanceof Number) {
double d = ((Number) value).doubleValue();
sum += (d * d);
}
}
if (sum > 0.0) {
sum = Math.sqrt(sum);
for (String key : ks) {
if (LearnerUtils.isAnnotation(key))
continue;
Serializable value = input.get(key);
if (value instanceof Number) {
input.put(key, ((Number) value).doubleValue() / sum);
}
}
}
return input;
}
/**
* @return the keys
*/
public String[] getKeys() {
return keys;
}
/**
* @param keys
* the keys to set
*/
public void setKeys(String[] keys) {
this.keys = keys;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy