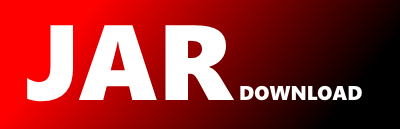
org.kafkacrypto.msgs.msgpack Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kafkacrypto-java Show documentation
Show all versions of kafkacrypto-java Show documentation
Java port of kafkacrypto, a lightweight and cross-platform/cross-language encryption library for Apache Kafka.
package org.kafkacrypto.msgs;
import org.kafkacrypto.msgs.Msgpacker;
import org.msgpack.core.MessagePack;
import org.msgpack.core.MessageBufferPacker;
import org.msgpack.core.MessagePacker;
import org.msgpack.core.MessageUnpacker;
import org.msgpack.value.Value;
import org.msgpack.value.IntegerValue;
import org.msgpack.value.FloatValue;
import org.msgpack.value.ValueType;
import java.util.List;
import java.util.Collection;
import java.util.Map;
import java.util.ArrayList;
import java.util.Arrays;
import java.math.BigInteger;
import java.io.IOException;
import java.lang.reflect.Array;
// msgpack
public class msgpack
{
public static List unpackb(byte[] input) throws IOException
{
MessagePack.UnpackerConfig cfg = new MessagePack.UnpackerConfig();
cfg.withAllowReadingBinaryAsString(false);
MessageUnpacker unpacker = cfg.newUnpacker(input);
List rv = new ArrayList();
while (unpacker.hasNext())
rv.add(unpacker.unpackValue());
unpacker.close();
if (rv.size() == 1 && rv.get(0).isArrayValue())
rv = rv.get(0).asArrayValue().list();
return rv;
}
protected static void packb_recurse(MessagePacker packer, boolean input) throws IOException
{
packer.packBoolean(input);
}
protected static void packb_recurse(MessagePacker packer, byte input) throws IOException
{
packer.packByte(input);
}
protected static void packb_recurse(MessagePacker packer, short input) throws IOException
{
packer.packShort(input);
}
protected static void packb_recurse(MessagePacker packer, int input) throws IOException
{
packer.packInt(input);
}
protected static void packb_recurse(MessagePacker packer, long input) throws IOException
{
packer.packLong(input);
}
protected static void packb_recurse(MessagePacker packer, float input) throws IOException
{
packer.packFloat(input);
}
protected static void packb_recurse(MessagePacker packer, double input) throws IOException
{
packer.packDouble(input);
}
protected static void packb_recurse(MessagePacker packer, byte[] input) throws IOException
{
if (input == null) {
packer.packNil();
} else {
packer.packBinaryHeader(input.length);
packer.writePayload(input);
}
}
protected static void packb_recurse(MessagePacker packer, Msgpacker input) throws IOException
{
if (input == null) {
packer.packNil();
} else {
input.packb(packer);
}
}
protected static void packb_recurse(MessagePacker packer, Collection input) throws IOException
{
if (input == null) {
packer.packNil();
} else {
packer.packArrayHeader(input.size());
for (E o : input)
msgpack.packb_recurse(packer, o);
}
}
protected static void packb_recurse(MessagePacker packer, Map input) throws IOException
{
if (input == null) {
packer.packNil();
} else {
packer.packMapHeader(input.size());
for (K ok : input.keySet()) {
msgpack.packb_recurse(packer, ok);
msgpack.packb_recurse(packer, input.get(ok));
}
}
}
protected static void packb_recurse(MessagePacker packer, BigInteger input) throws IOException
{
if (input == null) {
packer.packNil();
} else {
packer.packBigInteger(input);
}
}
protected static void packb_recurse(MessagePacker packer, String input) throws IOException
{
if (input == null) {
packer.packNil();
} else {
packer.packString(input);
}
}
protected static void packb_recurse(MessagePacker packer, T input) throws IOException
{
if (input == null) {
packer.packNil();
} else if (input instanceof byte[]) {
msgpack.packb_recurse(packer, (byte[])input);
} else if (input instanceof Byte) {
msgpack.packb_recurse(packer, (byte)input);
} else if (input instanceof Short) {
msgpack.packb_recurse(packer, (short)input);
} else if (input instanceof Integer) {
msgpack.packb_recurse(packer, (int)input);
} else if (input instanceof Long) {
msgpack.packb_recurse(packer, (long)input);
} else if (input instanceof BigInteger) {
msgpack.packb_recurse(packer, (BigInteger)input);
} else if (input instanceof Float) {
msgpack.packb_recurse(packer, (float)input);
} else if (input instanceof Double) {
msgpack.packb_recurse(packer, (double)input);
} else if (input instanceof Msgpacker>) {
((Msgpacker>)input).packb(packer);
} else if (input instanceof Collection>) {
msgpack.packb_recurse(packer, (Collection>)input);
} else if (input instanceof Map,?>) {
msgpack.packb_recurse(packer, (Map,?>)input);
} else if (input.getClass().isArray()) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy