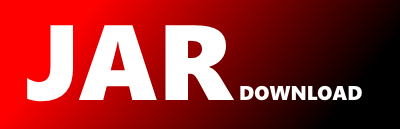
org.kasource.spring.integration.SpringBeanResolver Maven / Gradle / Ivy
package org.kasource.spring.integration;
import java.lang.annotation.Annotation;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.kasource.di.bean.BeanNotFoundException;
import org.kasource.di.bean.BeanResolver;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.stereotype.Repository;
@Repository
public class SpringBeanResolver implements BeanResolver, ApplicationContextAware {
private ApplicationContext applicationContext;
@Override
public T getBean(String beanName, Class ofType) throws BeanNotFoundException {
try {
return applicationContext.getBean(beanName, ofType);
} catch (BeansException e) {
throw new BeanNotFoundException("Could not find any bean named: " + beanName + " of class: " + ofType, e);
}
}
@Override
public Set getBeans(Class ofType) {
Set beansFound = new HashSet();
beansFound.addAll(applicationContext.getBeansOfType(ofType).values());
return beansFound;
}
@SuppressWarnings("unchecked")
@Override
public Set getBeansByQualifier(Class ofType, Class extends Annotation>... qualifiers) {
Set beansFound = new HashSet();
if (qualifiers.length == 0) {
for ( Class extends Annotation> qualifier : qualifiers) {
Map beans = applicationContext.getBeansWithAnnotation(qualifier);
for (Object bean : beans.values()) {
if (ofType.isAssignableFrom(bean.getClass())) {
beansFound.add((T) bean);
}
}
}
} else {
Map beans = applicationContext.getBeansOfType(ofType);
beansFound.addAll(beans.values());
}
return beansFound;
}
@Override
public Set getBeansByQualifier(Class ofType, Annotation... qualifiers) {
Set beansFound = new HashSet();
Map beans = applicationContext.getBeansOfType(ofType);
if (qualifiers.length == 0) {
beansFound.addAll(beans.values());
}
for (T bean : beans.values()) {
for (Annotation annotation : qualifiers) {
Annotation found = bean.getClass().getAnnotation(annotation.getClass());
if (annotation.equals(found)) {
beansFound.add(bean);
}
}
}
return beansFound;
}
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
this.applicationContext = applicationContext;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy