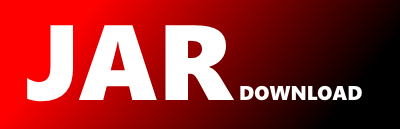
org.kathra.api.KathraCatalogUpdater Maven / Gradle / Ivy
/*
* Copyright 2019 The Kathra Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Contributors:
*
* IRT SystemX (https://www.kathra.org/)
*
*/
package org.kathra.api;
import io.fabric8.kubernetes.api.model.ConfigMap;
import io.fabric8.kubernetes.api.model.ConfigMapList;
import io.fabric8.kubernetes.client.DefaultKubernetesClient;
import io.fabric8.kubernetes.client.KubernetesClient;
import io.fabric8.kubernetes.client.Watch;
import io.fabric8.kubernetes.client.Watcher;
import io.fabric8.kubernetes.client.dsl.FilterWatchListDeletable;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.IOCase;
import org.apache.commons.io.IOUtils;
import org.apache.commons.io.filefilter.FileFilterUtils;
import org.yaml.snakeyaml.Yaml;
import org.kathra.api.Config;
import java.io.File;
import java.util.Collection;
import java.util.Map;
public class KathraCatalogUpdater {
private Config config = null;
protected static KubernetesClient client = new DefaultKubernetesClient();
static Yaml yaml = new Yaml();
public static void main(String[] args) throws Exception {
Config config = new Config();
config.initConfig();
String domain = config.getDomain();
if(domain==null) domain = "irtsystemx.org";
String protocol = config.getProtocol();
if(protocol == null || protocol.equals("")) protocol = "http";
String namespace = client.getNamespace();
if(namespace==null || namespace.equals("")) namespace = "kathra-catalog";
File[] directories = new File("/data/kathra-catalog/stable").listFiles(File::isDirectory);
FilterWatchListDeletable> cmDel = client.configMaps().inNamespace(namespace).withLabel("kind", "catalog");
if(cmDel.list().getItems()!=null) cmDel.delete();
for(File dir : directories) {
System.out.print("Parsing : "+dir.getName()+" -> ");
File configMapFile = FileUtils.getFile(dir, "configmap.yml");
if(!configMapFile.exists()) {
configMapFile = FileUtils.getFile(dir, "configmap.yaml");
}
String configMapContent = FileUtils.readFileToString(configMapFile);
Map configMap = (Map) yaml.load(configMapContent);
if(configMap==null) {
System.out.println("ERROR");
continue;
}
ConfigMap cm = client.configMaps().load(IOUtils.toInputStream(yaml.dump(configMap))).get();
Collection icons = FileUtils.listFiles(dir, FileFilterUtils.prefixFileFilter("icon.", IOCase.INSENSITIVE), null);
String iconName = null;
for(File icon : icons) {
iconName = icon.getName();
}
cm.getMetadata().getAnnotations().put("kathra.icon", protocol + "://icons." + namespace + "." + domain +"/kathra-catalog/stable/"+dir.getName()+"/"+iconName);
client.resource(cm).inNamespace(namespace).createOrReplace();
System.out.println("SUCCESS");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy