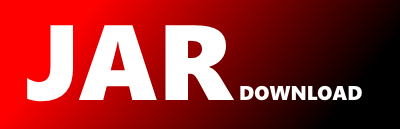
org.kathra.codegen.languages.KathraPythonCodegen Maven / Gradle / Ivy
/*
* Copyright 2019 The Kathra Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Contributors:
*
* IRT SystemX (https://www.kathra.org/)
*
*/
package org.kathra.codegen.languages;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.Lists;
import com.google.common.collect.Multimap;
import org.kathra.codegen.*;
import io.swagger.models.*;
import io.swagger.models.parameters.*;
import io.swagger.models.properties.*;
import io.swagger.util.Yaml;
import org.apache.commons.lang3.StringUtils;
import java.io.File;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class KathraPythonCodegen extends DefaultCodegen implements CodegenConfig {
public static final String MODEL_MUSTACHE = "model.mustache";
public static final String REPOSITORY_URI = "repositoryUrl";
public static final String REPOSITORY_PYTHON_NAME = "repositoryPythonName";
public static final String GROUP_ID = "groupId";
public static final String ARTIFACT_ID = "artifactId";
public static final String ARTIFACT_VERSION = "artifactVersion";
public static final String OBJECTS = "objects";
public static final String X_GROUP_ID = "x-groupId";
public static final String X_MODEL_PACKAGE = "x-modelPackage";
public static final String SERVICE_MUSTACHE = "service.mustache";
public static final String LAUNCHER_MUSTACHE = "launcher.mustache";
public static final String INIT_MUSTACHE = "__init__.mustache";
public static final String SOURCE_FOLDER_ATTRIBUTE_NAME = "sourceFolder";
public static final String SUPPORT_PYTHON2 = "supportPython2";
public static final String SWAGGER_MUSTACHE = "swagger.mustache";
public static final String SWAGGER_YAML = "swagger.yaml";
protected int serverPort = 8080;
protected String packageName;
protected String packageVersion;
protected String packagePrefix = "";
protected Map regexModifiers;
protected String apiName;
protected String apiSuffix;
protected String invokerPackage = "org.kathra";
protected String projectFolder;
protected String sourceFolder;
protected String repositoryUrl = "undefined";
protected String repositoryPythonName = "undefined";
protected String groupId = "org.kathra";
protected String artifactId = "kathra-autogenerated-artifact";
protected String artifactVersion = "1.0.0-SNAPSHOT";
protected String artifactVersionApi = "1.0.0-SNAPSHOT";
protected String interfaceModule = "";
protected String modelModule = "";
protected String implModule = "";
protected String moduleName = "";
protected String modulePrefix = "";
HashMap kathraImports = new HashMap();
public KathraPythonCodegen() {
super();
modelPackage = "models";
testPackage = "test";
languageSpecificPrimitives.clear();
languageSpecificPrimitives.add("int");
languageSpecificPrimitives.add("float");
languageSpecificPrimitives.add("List");
languageSpecificPrimitives.add("Dict");
languageSpecificPrimitives.add("bool");
languageSpecificPrimitives.add("str");
languageSpecificPrimitives.add("datetime");
languageSpecificPrimitives.add("date");
languageSpecificPrimitives.add("file");
languageSpecificPrimitives.add("object");
typeMapping.clear();
typeMapping.put("integer", "int");
typeMapping.put("float", "float");
typeMapping.put("number", "float");
typeMapping.put("long", "int");
typeMapping.put("double", "float");
typeMapping.put("array", "List");
typeMapping.put("map", "Dict");
typeMapping.put("boolean", "bool");
typeMapping.put("string", "str");
typeMapping.put("date", "date");
typeMapping.put("DateTime", "datetime");
typeMapping.put("object", "object");
typeMapping.put("file", "file");
typeMapping.put("UUID", "str");
// from https://docs.python.org/3/reference/lexical_analysis.html#keywords
setReservedWordsLowerCase(
Arrays.asList(
// @property
// python reserved words
"and", "del", "from", "not", "while", "as", "elif", "global", "or", "with",
"assert", "else", "if", "pass", "yield", "break", "except", "import",
"print", "class", "exec", "in", "raise", "continue", "finally", "is",
"return", "def", "for", "lambda", "try", "self", "None", "True", "False", "nonlocal"));
// set the output folder here
outputFolder = "generated-code" + File.separator + "python";
//apiTestTemplateFiles().put(API_TEST_MUSTACHE, ".py");
/*
* Additional Properties. These values can be passed to the templates and
* are available in models, apis, and supporting files
*/
additionalProperties.put("serverPort", serverPort);
/*
* Supporting Files. You can write single files for the generator with the
* entire object tree available. If the input file has a suffix of `.mustache
* it will be processed by the template engine. Otherwise, it will be copied
*/
//supportingFiles.add(new SupportingFile("README.mustache", "", "README.md"));
supportingFiles.add(new SupportingFile("setup.mustache", "", "setup.py"));
supportingFiles.add(new SupportingFile("gitignore.mustache", "", ".gitignore"));
//supportingFiles.add(new SupportingFile("MANIFEST.in.mustache", "", "MANIFEST.in"));
regexModifiers = new HashMap();
regexModifiers.put('i', "IGNORECASE");
regexModifiers.put('l', "LOCALE");
regexModifiers.put('m', "MULTILINE");
regexModifiers.put('s', "DOTALL");
regexModifiers.put('u', "UNICODE");
regexModifiers.put('x', "VERBOSE");
supportedLibraries.put(KathraLibraryType.MODEL.getName(), "Generate Model");
supportedLibraries.put(KathraLibraryType.INTERFACE.getName(), "Generate Interface + Api");
supportedLibraries.put(KathraLibraryType.IMPLEM.getName(), "Generate Implementation");
supportedLibraries.put(KathraLibraryType.CLIENT.getName(), "Generate Client");
CliOption libraryOption = new CliOption(CodegenConstants.LIBRARY, "library template (sub-template) to use");
libraryOption.setEnum(supportedLibraries);
// set model as the default
libraryOption.setDefault(KathraLibraryType.MODEL.getName());
cliOptions.add(libraryOption);
setLibrary(KathraLibraryType.MODEL.getName());
}
@Override
public void processOpts() {
if (additionalProperties.containsKey(CodegenConstants.TEMPLATE_DIR)) {
this.setTemplateDir((String) additionalProperties.get(CodegenConstants.TEMPLATE_DIR));
}
if (additionalProperties.containsKey(CodegenConstants.MODEL_PACKAGE)) {
this.setModelPackage((String) additionalProperties.get(CodegenConstants.MODEL_PACKAGE));
}
if (additionalProperties.containsKey(CodegenConstants.API_PACKAGE)) {
this.setApiPackage((String) additionalProperties.get(CodegenConstants.API_PACKAGE));
}
if (additionalProperties.containsKey(CodegenConstants.SORT_PARAMS_BY_REQUIRED_FLAG)) {
this.setSortParamsByRequiredFlag(Boolean.valueOf(additionalProperties
.get(CodegenConstants.SORT_PARAMS_BY_REQUIRED_FLAG).toString()));
}
if (additionalProperties.containsKey(CodegenConstants.ENSURE_UNIQUE_PARAMS)) {
this.setEnsureUniqueParams(Boolean.valueOf(additionalProperties
.get(CodegenConstants.ENSURE_UNIQUE_PARAMS).toString()));
}
if (additionalProperties.containsKey(CodegenConstants.ALLOW_UNICODE_IDENTIFIERS)) {
this.setAllowUnicodeIdentifiers(Boolean.valueOf(additionalProperties
.get(CodegenConstants.ALLOW_UNICODE_IDENTIFIERS).toString()));
}
if (additionalProperties.containsKey(CodegenConstants.MODEL_NAME_PREFIX)) {
this.setModelNamePrefix((String) additionalProperties.get(CodegenConstants.MODEL_NAME_PREFIX));
}
if (additionalProperties.containsKey(CodegenConstants.MODEL_NAME_SUFFIX)) {
this.setModelNameSuffix((String) additionalProperties.get(CodegenConstants.MODEL_NAME_SUFFIX));
}
if (additionalProperties.containsKey(CodegenConstants.REMOVE_OPERATION_ID_PREFIX)) {
this.setSortParamsByRequiredFlag(Boolean.valueOf(additionalProperties
.get(CodegenConstants.REMOVE_OPERATION_ID_PREFIX).toString()));
}
if ((additionalProperties.get(CodegenConstants.ARTIFACT_ID) != null) && (!additionalProperties.get(CodegenConstants.ARTIFACT_ID).toString().trim().isEmpty()))
artifactId = additionalProperties.get(CodegenConstants.ARTIFACT_ID).toString().trim();
else
additionalProperties.put(CodegenConstants.ARTIFACT_ID, artifactId);
if ((additionalProperties.get(CodegenConstants.MODEL_PACKAGE) != null) && (!additionalProperties.get(CodegenConstants.MODEL_PACKAGE).toString().trim().isEmpty()))
modelPackage = additionalProperties.get(CodegenConstants.MODEL_PACKAGE).toString().trim();
else
additionalProperties.put(CodegenConstants.MODEL_PACKAGE, modelPackage);
if ((additionalProperties.get(CodegenConstants.API_PACKAGE) != null) && (!additionalProperties.get(CodegenConstants.API_PACKAGE).toString().trim().isEmpty()))
apiPackage = additionalProperties.get(CodegenConstants.API_PACKAGE).toString().trim();
else
additionalProperties.put(CodegenConstants.API_PACKAGE, apiPackage);
if ((additionalProperties.get(CodegenConstants.INVOKER_PACKAGE) != null) && (!additionalProperties.get(CodegenConstants.INVOKER_PACKAGE).toString().trim().isEmpty()))
invokerPackage = additionalProperties.get(CodegenConstants.INVOKER_PACKAGE).toString().trim();
else
additionalProperties.put(CodegenConstants.INVOKER_PACKAGE, invokerPackage);
invokerPackage = invokerPackage.toLowerCase();
if ((additionalProperties.get(CodegenConstants.REPOSITORY_URL) != null) && (!additionalProperties.get(CodegenConstants.REPOSITORY_URL).toString().trim().isEmpty()))
repositoryUrl = additionalProperties.get(CodegenConstants.REPOSITORY_URL).toString().trim();
else
additionalProperties.put(CodegenConstants.REPOSITORY_URL, repositoryUrl);
if ((additionalProperties.get(CodegenConstants.REPOSITORY_PYTHON_NAME) != null) && (!additionalProperties.get(CodegenConstants.REPOSITORY_PYTHON_NAME).toString().trim().isEmpty()))
repositoryPythonName = additionalProperties.get(CodegenConstants.REPOSITORY_PYTHON_NAME).toString().trim();
else
additionalProperties.put(CodegenConstants.REPOSITORY_PYTHON_NAME, repositoryPythonName);
if ((additionalProperties.get(CodegenConstants.GROUP_ID) != null) && (!additionalProperties.get(CodegenConstants.GROUP_ID).toString().trim().isEmpty()))
groupId = additionalProperties.get(CodegenConstants.GROUP_ID).toString().trim();
else
additionalProperties.put(CodegenConstants.GROUP_ID, groupId);
groupId = groupId.toLowerCase();
if ((additionalProperties.get(CodegenConstants.ARTIFACT_VERSION) != null) && (!additionalProperties.get(CodegenConstants.ARTIFACT_VERSION).toString().trim().isEmpty()))
artifactVersion = additionalProperties.get(CodegenConstants.ARTIFACT_VERSION).toString().trim();
else
additionalProperties.put(CodegenConstants.ARTIFACT_VERSION, artifactVersion);
if (additionalProperties.containsKey(CodegenConstants.ARTIFACT_VERSION_API)) {
artifactVersionApi = additionalProperties.get(CodegenConstants.ARTIFACT_VERSION_API).toString();
}
if ((additionalProperties.get(CodegenConstants.MODEL_NAME_PREFIX) != null) && (!additionalProperties.get(CodegenConstants.MODEL_NAME_PREFIX).toString().trim().isEmpty()))
modelNamePrefix = additionalProperties.get(CodegenConstants.MODEL_NAME_PREFIX).toString().trim();
else
additionalProperties.put(CodegenConstants.MODEL_NAME_PREFIX, modelNamePrefix);
if ((additionalProperties.get(CodegenConstants.MODEL_NAME_SUFFIX) != null) && (!additionalProperties.get(CodegenConstants.MODEL_NAME_SUFFIX).toString().trim().isEmpty()))
modelNameSuffix = additionalProperties.get(CodegenConstants.MODEL_NAME_SUFFIX).toString().trim();
else
additionalProperties.put(CodegenConstants.MODEL_NAME_SUFFIX, modelNameSuffix);
if ((additionalProperties.get(CodegenConstants.GIT_USER_ID) != null) && (!additionalProperties.get(CodegenConstants.GIT_USER_ID).toString().trim().isEmpty()))
gitUserId = additionalProperties.get(CodegenConstants.GIT_USER_ID).toString().trim();
else
additionalProperties.put(CodegenConstants.GIT_USER_ID, gitUserId);
if ((additionalProperties.get(CodegenConstants.GIT_REPO_ID) != null) && (!additionalProperties.get(CodegenConstants.GIT_REPO_ID).toString().trim().isEmpty()))
gitRepoId = additionalProperties.get(CodegenConstants.GIT_REPO_ID).toString().trim();
else
additionalProperties.put(CodegenConstants.GIT_REPO_ID, gitRepoId);
if ((additionalProperties.get(CodegenConstants.RELEASE_NOTE) != null) && (!additionalProperties.get(CodegenConstants.RELEASE_NOTE).toString().trim().isEmpty()))
releaseNote = additionalProperties.get(CodegenConstants.RELEASE_NOTE).toString().trim();
else
additionalProperties.put(CodegenConstants.RELEASE_NOTE, gitRepoId);
if ((additionalProperties.get(CodegenConstants.HTTP_USER_AGENT) != null) && (!additionalProperties.get(CodegenConstants.HTTP_USER_AGENT).toString().trim().isEmpty()))
httpUserAgent = additionalProperties.get(CodegenConstants.HTTP_USER_AGENT).toString().trim();
else
additionalProperties.put(CodegenConstants.HTTP_USER_AGENT, gitRepoId);
if (additionalProperties.containsKey(CodegenConstants.PACKAGE_NAME)) {
setPackageName((String) additionalProperties.get(CodegenConstants.PACKAGE_NAME));
}
if (additionalProperties.containsKey(CodegenConstants.PACKAGE_VERSION)) {
setPackageVersion((String) additionalProperties.get(CodegenConstants.PACKAGE_VERSION));
} else {
setPackageVersion("1.0.0-SNAPSHOT");
additionalProperties.put(CodegenConstants.PACKAGE_VERSION, this.packageVersion);
}
if (Boolean.TRUE.equals(additionalProperties.get(SUPPORT_PYTHON2))) {
additionalProperties.put(SUPPORT_PYTHON2, Boolean.TRUE);
typeMapping.put("long", "long");
}
intializeAdditionalProperties();
}
private void setArtifactIdApi(String artifactVersionApi) {
this.artifactVersionApi = artifactVersionApi;
}
public void intializeAdditionalProperties() {
additionalProperties.put("artifactName", generateArtifactName(artifactId));
packageName = artifactId = artifactId.toLowerCase();
additionalProperties.put("artifactId", artifactId);
packageVersion = artifactVersion;
moduleName = sourceFolder = projectFolder = artifactId.replace('-', '_');
modulePrefix = projectFolder.substring(0, projectFolder.lastIndexOf('_') + 1);
packagePrefix = modulePrefix.replace('_', '-');
apiName = StringUtils.capitalize(apiPackage);
additionalProperties.put("apiName", apiName);
modelPackage = packagePrefix + KathraLibraryType.MODEL.getName().toLowerCase();
modelModule = modelPackage.replace('-', '_');
/*
* Template Location. This is the location templates will be read from.
* The generator will use the resource stream to attempt to read the templates.
*/
embeddedTemplateDir = templateDir = "Kathra/Python/" + getLibrary();
if (KathraLibraryType.MODEL.getName().equalsIgnoreCase(getLibrary())) {
apiSuffix = "Model";
modelTemplateFiles.put(MODEL_MUSTACHE, ".py");
} else if (KathraLibraryType.INTERFACE.getName().equalsIgnoreCase(getLibrary())) {
apiTemplateFiles.put(SERVICE_MUSTACHE, ".py");
apiSuffix = "Service";
} else if (KathraLibraryType.IMPLEM.getName().equalsIgnoreCase(getLibrary())) {
apiSuffix = "Controller";
apiTemplateFiles.put("controller.mustache", ".py");
supportingFiles.add(new SupportingFile(SWAGGER_MUSTACHE, "", SWAGGER_YAML));
supportingFiles.add(new SupportingFile(LAUNCHER_MUSTACHE, "", "launcher.py"));
supportingFiles.add(new SupportingFile("Dockerfile.mustache", "", "Dockerfile"));
supportingFiles.add(new SupportingFile("Pipfile.mustache", "", "Pipfile"));
supportingFiles.add(new SupportingFile("README.mustache", "", "README.md"));
interfaceModule = (modulePrefix + KathraLibraryType.INTERFACE.getName() + "." + underscore(apiPackage) + "_service").toLowerCase();
} else if (KathraLibraryType.CLIENT.getName().equalsIgnoreCase(getLibrary())) {
apiSuffix = "Controller";
}
supportingFiles.add(new SupportingFile(INIT_MUSTACHE, sourceFolder, "__init__.py"));
supportingFiles.add(new SupportingFile("requirements.mustache", sourceFolder, "requirements.txt"));
//The other library (implementation, client, interface) must import packages and classes of model
//The implementation library must import packages and classes of model and interface
//The implementation class must import its own classes
additionalProperties.put(SOURCE_FOLDER_ATTRIBUTE_NAME, sourceFolder);
additionalProperties.put("packageName", packageName);
additionalProperties.put("packageVersion", packageVersion);
additionalProperties.put("moduleName", moduleName);
additionalProperties.put("modulePrefix", modulePrefix);
additionalProperties.put("packagePrefix", packagePrefix);
additionalProperties.put("modelPackage", modelPackage);
additionalProperties.put("modelModule", modelModule);
additionalProperties.put("interfaceModule", interfaceModule);
additionalProperties.put("className", apiName + apiSuffix);
additionalProperties.put("classFileName", underscore(apiName + apiSuffix));
}
private String generateArtifactName(String artifactId) {
String[] parts = artifactId.split("\\-");
StringBuilder f = new StringBuilder();
for (int i = 0; i < parts.length; i++) {
String z = parts[i];
if (z.length() > 0) {
if (i == 0) {
f.append(z.toUpperCase());
} else {
f.append(StringUtils.capitalize(z));
}
if (i < parts.length - 1) {
f.append(" :: ");
}
}
}
return f.toString();
}
@Override
public void preprocessSwagger(Swagger swagger) {
super.preprocessSwagger(swagger);
if (swagger.getInfo().getTitle() == null || swagger.getInfo().getTitle().isEmpty()) {
swagger.getInfo().setTitle(apiName);
}
handleKathraOperationId(swagger);
handleKathraDependencies(swagger);
handleKathraTags(swagger);
}
private void handleKathraOperationId(Swagger swagger) {
// need vendor extensions for x-swagger-router-controller
Map paths = swagger.getPaths();
if (paths != null) {
for (String pathname : paths.keySet()) {
Path path = paths.get(pathname);
Map operationMap = path.getOperationMap();
if (operationMap != null) {
for (HttpMethod method : operationMap.keySet()) {
Operation operation = operationMap.get(method);
String tag = apiName;
if (operation.getTags() != null && operation.getTags().size() > 0) {
tag = operation.getTags().get(0);
}
String operationId = operation.getOperationId();
if (operationId == null) {
operationId = getOrGenerateOperationId(operation, pathname, method.toString());
}
operation.setOperationId(toOperationId(operationId));
for (Parameter param : operation.getParameters()) {
// sanitize the param name but don't underscore it since it's used for request mapping
String name = param.getName();
String paramName = sanitizeName(name);
if (!paramName.equals(name)) {
LOGGER.warn(name + " cannot be used as parameter name with flask-connexion and was sanitized as " + paramName);
}
param.setName(paramName);
}
}
}
}
}
}
private void handleKathraTags(Swagger swagger) {
if (KathraLibraryType.IMPLEM.getName().equalsIgnoreCase(getLibrary()) || KathraLibraryType.INTERFACE.getName().equalsIgnoreCase(getLibrary()) || KathraLibraryType.CLIENT.getName().equalsIgnoreCase(getLibrary())) {
for (Path p : swagger.getPaths().values()) {
for (Operation op : p.getOperations()) {
if (op.getTags() == null || op.getTags().isEmpty()) {
op.addTag(apiName);
}
}
}
}
}
private void handleKathraDependencies(Swagger swagger) {
Map definitions = swagger.getDefinitions();
if (definitions == null) definitions = new HashMap();
HashMap dependencies = new HashMap();
List artifactDependencies = parseKathraDependencies(swagger.getVendorExtensions());
additionalProperties.put("artifactDependencies", artifactDependencies);
for (Iterator> iterator = definitions.entrySet().iterator(); iterator.hasNext(); ) {
Map.Entry modelEntry = iterator.next();
Model model = modelEntry.getValue();
String modelName = modelEntry.getKey();
// Handling external models from other Api definitions
if (model.getProperties() == null && !(model instanceof ComposedModel)) {
dependencies.putAll(handleExternalModel(modelName, model.getVendorExtensions(), artifactDependencies));
continue;
}
// Handling autogenerated model from same Api definition
if (!KathraLibraryType.MODEL.getName().equalsIgnoreCase(getLibrary())) {
handleInternalModel(dependencies, modelName);
}
}
if (KathraLibraryType.MODEL.getName().equalsIgnoreCase(getLibrary())) {
for (Map.Entry modelEntry : definitions.entrySet()) {
Model model = modelEntry.getValue();
String modelName = modelEntry.getKey();
processModelDescription(modelName, model);
processModelProperties(dependencies, model);
}
for (HashMap dep : dependencies.values()) {
if (!((List) dep.get(OBJECTS)).isEmpty()) {
addArtifactDependency(artifactDependencies, dep);
}
}
} else {
for (HashMap dep : dependencies.values()) {
addArtifactDependency(artifactDependencies, dep);
}
if (KathraLibraryType.IMPLEM.getName().equalsIgnoreCase(getLibrary())) {
HashMap dep = new HashMap();
dep.put(ARTIFACT_ID, packagePrefix + KathraLibraryType.INTERFACE.getName());
dep.put(ARTIFACT_VERSION, artifactVersionApi);
addArtifactDependency(artifactDependencies, dep);
}
}
}
private List parseKathraDependencies(Map vendorExtensions) {
if (vendorExtensions != null && !vendorExtensions.isEmpty() && vendorExtensions.containsKey("x-dependencies")) {
return (List) vendorExtensions.get("x-dependencies");
}
return new ArrayList();
}
private void processModelProperties(HashMap dependencies, Model model) {
if (model.getProperties() != null) {
for (Map.Entry propertyEntry : model.getProperties().entrySet()) {
Property property = propertyEntry.getValue();
String refName = null;
if (property instanceof RefProperty) refName = ((RefProperty) property).getSimpleRef();
else if (property instanceof RefModel) refName = ((RefModel) property).getSimpleRef();
countDependency(dependencies, refName);
}
} else if (model instanceof ComposedModel) {
ComposedModel cmodel = (ComposedModel) model;
processModelProperties(dependencies, cmodel.getChild());
countDependency(dependencies, cmodel.getInterfaces().get(0).getSimpleRef());
}
}
private void countDependency(HashMap dependencies, String refName) {
if (refName != null && kathraImports.containsKey(refName)) {
HashMap dep = dependencies.get(kathraImports.get(refName));
if (!((ArrayList) dep.get(OBJECTS)).contains(refName)) {
((ArrayList) dep.get(OBJECTS)).add(refName);
}
}
}
private void processModelDescription(String modelName, Model model) {
if (model.getDescription() == null || model.getDescription().isEmpty()) {
model.setDescription(modelName);
}
if (model.getProperties() != null) {
for (Map.Entry propertyEntry : model.getProperties().entrySet()) {
Property property = propertyEntry.getValue();
if (property.getDescription() == null || property.getDescription().isEmpty()) {
property.setDescription(propertyEntry.getKey());
}
}
} else if (model instanceof ComposedModel) {
ComposedModel cmodel = (ComposedModel) model;
cmodel.setParent(cmodel.getInterfaces().get(0));
if (cmodel.getChild() == null) {
cmodel.setChild(new ModelImpl().type("object"));
}
ModelImpl modelImpl = (ModelImpl) cmodel.getChild();
processModelDescription(modelName, modelImpl);
}
}
private void handleInternalModel(HashMap dependencies, String modelName) {
kathraImports.put(modelName, modelModule + "." + underscore(modelName));
if (!dependencies.containsKey(kathraImports.get(modelName))) {
HashMap dep = new HashMap();
dep.put(ARTIFACT_ID, modelPackage);
dep.put(ARTIFACT_VERSION, artifactVersionApi);
dependencies.put(kathraImports.get(modelName), dep);
}
}
@Override
public String toModelImport(String name) {
String modelImport = "from ";
if (KathraLibraryType.MODEL.getName().equalsIgnoreCase(getLibrary())) {
modelImport += modelModule + "." + underscore(name);
} else {
if (kathraImports.containsKey(name)) {
modelImport += kathraImports.get(name);
} else {
return invokerPackage + "." + apiPackage.toLowerCase() + "." + name;
}
}
return modelImport + " import " + name;
}
private Map handleExternalModel(String modelName, Map vendorExtensions, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy