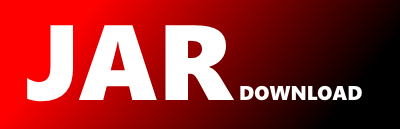
org.kefirsf.bb.EscapeProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kefirbb Show documentation
Show all versions of kefirbb Show documentation
KefirBB is a Java-library for text processing. Initially it was developed for BB2HTML translation.
But flexible configuration allows to use it in different cases. For example for parsing Markdown, Textile,
and for HTML filtration.
The newest version!
package org.kefirsf.bb;
import java.util.Arrays;
/**
* Class for escape processing. For example, EscapeXmlProcessorFactory use this class for create EscapeXmlProcessor.
*
* @author Kefir
*/
public class EscapeProcessor extends TextProcessorAdapter {
/**
* Escape symbols with replacement.
*/
private final String[][] escape;
/**
* Construct the escape processor with special escape symbols.
*
* @param escape escape symbols with replacement. This is a array of array wich consist of two strings
* the pattern and the replacement
*/
public EscapeProcessor(String[][] escape) {
this.escape = escape;
}
/**
* Process the text
*
* @param source the sourcetext
* @return the result of text processing
* @see TextProcessor#process(CharSequence)
*/
public CharSequence process(CharSequence source) {
if (source != null && source.length() > 0) {
String stringSource = source instanceof String ? (String) source : source.toString();
// Array to cache founded indexes of sequences
int[] indexes = new int[escape.length];
Arrays.fill(indexes, -1);
int length = source.length();
int offset = 0;
StringBuilder result = new StringBuilder(length);
while (offset < length) {
// Find next escape sequence
int escPosition = -1;
int escIndex = -1;
for (int i = 0; i < escape.length; i++) {
int index;
if (indexes[i] < offset) {
index = stringSource.indexOf(escape[i][0], offset);
indexes[i] = index;
} else {
index = indexes[i];
}
if (index >= 0 && (index < escPosition || escPosition < 0)) {
escPosition = index;
escIndex = i;
}
}
// If escape sequence is found
if (escPosition >= 0) {
// replace chars before escape sequence
result.append(stringSource, offset, escPosition);
// Replace sequence
result.append(escape[escIndex][1]);
offset = escPosition + escape[escIndex][0].length();
} else {
// Put other string to result sequence
result.append(stringSource, offset, length);
offset = length;
}
}
return result;
} else {
return new StringBuilder(0);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy