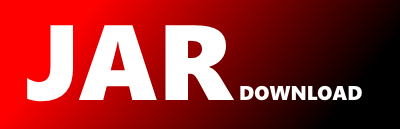
templates.json.JsonReader.vm Maven / Gradle / Ivy
#* @vtlvariable name="ctx" type="org.kevoree.modeling.kotlin.generator.GenerationContext" *#
#* @vtlvariable name="helper" type="org.kevoree.modeling.kotlin.generator.ProcessorHelperClass" *#
package ${helper.fqn($ctx, $ctx.getBasePackageForUtilitiesGeneration())}.loader
import java.io.InputStream
class JsonReader(ins: InputStream) {
val PEEKED_NONE = 0;
val PEEKED_BEGIN_OBJECT = 1;
val PEEKED_END_OBJECT = 2;
val PEEKED_BEGIN_ARRAY = 3;
val PEEKED_END_ARRAY = 4;
val PEEKED_TRUE = 5;
val PEEKED_FALSE = 6;
val PEEKED_NULL = 7;
val PEEKED_SINGLE_QUOTED = 8;
val PEEKED_DOUBLE_QUOTED = 9;
val PEEKED_UNQUOTED = 10;
val PEEKED_BUFFERED = 11;
val PEEKED_SINGLE_QUOTED_NAME = 12;
val PEEKED_DOUBLE_QUOTED_NAME = 13;
val PEEKED_UNQUOTED_NAME = 14;
val PEEKED_LONG = 15;
val PEEKED_NUMBER = 16;
val PEEKED_EOF = 17;
var lexer: Lexer = Lexer(ins)
var token: Any? = null
fun hasNext(): Boolean {
if (token == null) {
doPeek()
}
val t = token as Token
return t.tokenType != Type.RIGHT_BRACE && t.tokenType != Type.RIGHT_BRACKET
}
private fun doPeek(): Any? {
token = lexer.nextToken()
val t = token as Token
if (t.tokenType == Type.COLON || t.tokenType == Type.COMMA) doPeek()
return token
}
fun peek(): Int {
if (token == null) doPeek()
val t = token as Token
when (t.tokenType) {
Type.LEFT_BRACE -> return JsonToken.BEGIN_OBJECT
Type.RIGHT_BRACE -> return JsonToken.END_OBJECT
Type.LEFT_BRACKET -> return JsonToken.BEGIN_ARRAY
Type.RIGHT_BRACKET -> return JsonToken.END_ARRAY
Type.VALUE -> return JsonToken.NAME
Type.EOF -> return JsonToken.END_DOCUMENT
else -> return JsonToken.NULL // we don't care actually cause only END_DOCUMENT is used
}
}
fun beginObject() {
if (token == null) {
doPeek()
}
val t = token as Token
if (t.tokenType == Type.LEFT_BRACE) {
token = null
} else {
throw IllegalStateException("Expected LEFT_BRACE but was " + peek());
}
}
fun endObject() {
if (token == null) doPeek()
val t = token as Token
if (t.tokenType == Type.RIGHT_BRACE) {
token = null
} else {
throw IllegalStateException("Expected RIGHT_BRACE but was " + peek());
}
}
fun beginArray() {
if (token == null) doPeek()
val t = token as Token
if (t.tokenType == Type.LEFT_BRACKET) {
token = null
} else {
throw IllegalStateException("Expected LEFT_BRACKET but was " + peek());
}
}
fun endArray() {
if (token == null) doPeek()
val t = token as Token
if (t.tokenType == Type.RIGHT_BRACKET) {
token = null
} else {
throw IllegalStateException("Expected RIGHT_BRACKET but was " + peek());
}
}
fun nextBoolean(): Boolean {
if (token == null) doPeek()
val t = token as Token
var ret: Boolean = false
if (t.tokenType == Type.VALUE) {
#if($ctx.jS)
when (t.value) {
"true", true -> ret = true
"false", false -> ret = false
else -> ret = false
}
#else
ret = t.value as Boolean
#end
token = null
} else {
throw IllegalStateException("Expected VALUE(Boolean) but was " + peek());
}
return ret
}
fun nextString(): String {
if (token == null) {
doPeek()
}
val t = token as Token
var ret: String = ""
if (t.tokenType == Type.VALUE) {
ret = t.value as String
token = null
} else {
throw IllegalStateException("Expected VALUE(String) but was " + peek());
}
return ret
}
fun nextInt(): Int {
if (token == null) doPeek()
val t = token as Token
var ret: Int = 42
if (t.tokenType == Type.VALUE) {
#if($ctx.jS)
val tret = java.lang.IntegerParser.parseInt(t.value as String)
if(tret != null){
ret = tret
}
#else
ret = Integer.parseInt(t.value as String)
#end
token = null
} else {
throw IllegalStateException("Expected VALUE(Int) but was " + peek());
}
return ret
}
fun nextName(): String {
if (token == null) {
doPeek()
}
val t = token as Token
var ret: String = ""
if (t.tokenType == Type.VALUE) {
ret = t.value as String
token = null
} else {
throw IllegalStateException("Expected VALUE(Name) but was " + peek());
}
return ret
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy