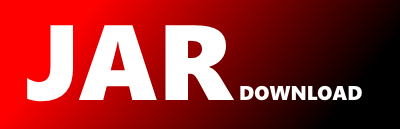
org.mwg.Graph Maven / Gradle / Ivy
/**
* Copyright 2017 The MWG Authors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.mwg;
import org.mwg.chunk.ChunkSpace;
import org.mwg.plugin.*;
import org.mwg.struct.Buffer;
import org.mwg.task.TaskActionFactory;
import org.mwg.task.TaskHook;
/**
* Graph is the main structure of mwDB.
* Use the {@link GraphBuilder} to get an instance.
*/
public interface Graph {
/**
* Creates a new (generic) {@link Node Node} in the Graph and returns the new Node.
*
* @param world initial world of the node
* @param time initial time of the node
* @return newly created node
*/
Node newNode(long world, long time);
/**
* Creates a new {@link Node Node} using the {@link NodeFactory} previously declared with the {@link GraphBuilder#withPlugin(Plugin)} method and returns the new Node.
*
* @param world initial world of the node
* @param time initial time of the node
* @param nodeType name of the {@link NodeFactory} to be used, as previously declared with the {@link GraphBuilder#withPlugin(Plugin)} method.
* @return newly created node
*/
Node newTypedNode(long world, long time, String nodeType);
/**
* Create a copy of this node that can be freed independently
*
* @param origin node to be cloned
* @return cloned node (aka pointer)
*/
Node cloneNode(Node origin);
/**
* Asynchronous lookup of a particular node.
* Based on the tuple <World, Time, Node_ID> this method seeks a {@link Node} in the Graph and returns it to the callback.
*
* @param world The world identifier in which the Node must be searched.
* @param time The time at which the Node must be resolved.
* @param id The unique identifier of the {@link Node} ({@link Node#id()}) researched.
* @param callback The task to be called when the {@link Node} is retrieved.
* @param the type of the parameter returned in the callback (should extend {@link Node}).
*/
void lookup(long world, long time, long id, Callback callback);
/**
* Asynchronous lookup of a nodes with different worlds and times.
* Based on the tuple <World, Time, Node_ID> this method seeks a {@link Node} in the Graph and returns it to the callback.
*
* @param worlds The worlds at which Nodes must be resolved.
* @param times The times at which Nodes must be resolved.
* @param ids The unique identifier of {@link Node} array ({@link Node#id()}) researched.
* @param callback The task to be called when the {@link Node} is retrieved.
*/
void lookupBatch(long worlds[], long times[], long[] ids, Callback callback);
/**
* Asynchronous lookup of a nodes.
* Based on the tuple <World, Time, Node_ID> this method seeks a {@link Node} in the Graph and returns it to the callback.
*
* @param world The world identifier in which the Node must be searched.
* @param time The time at which the Node must be resolved.
* @param ids The unique identifier of {@link Node} array ({@link Node#id()}) researched.
* @param callback The task to be called when the {@link Node} is retrieved.
*/
void lookupAll(long world, long time, long[] ids, Callback callback);
/**
* Asynchronous lookup of a nodes.
* Based on the tuple <World, Time, Node_ID> this method seeks a {@link Node} in the Graph and returns it to the callback.
*
* @param world The world identifier in which the Node must be searched.
* @param from The time at which the range extract should start.
* @param to The time at which the range extract should end.
* @param id The unique identifier of the {@link Node} ({@link Node#id()}) researched.
* @param callback The task to be called when the {@link Node} is retrieved.
*/
void lookupTimes(long world, long from, long to, long id, Callback callback);
/**
* Asynchronous lookup of a nodes.
* Based on the tuple <World, Time, Node_ID> this method seeks a {@link Node} in the Graph and returns it to the callback.
*
* @param world The world identifier in which the Node must be searched.
* @param from The time at which the range extract should start.
* @param to The time at which the range extract should end.
* @param ids The unique identifier of {@link Node} array ({@link Node#id()}) researched.
* @param callback The task to be called when the {@link Node} is retrieved.
*/
void lookupAllTimes(long world, long from, long to, long[] ids, Callback callback);
/**
* Creates a spin-off world from the world given as parameter.
* The forked world can then be altered independently of its parent.
* Every modification in the parent world will nevertheless be inherited.
* In case of concurrent change, changes in the forked world overrides changes from the parent.
*
* @param world origin world id
* @return newly created child world id (to be used later in lookup method for instance)
*/
long fork(long world);
/**
* Triggers a save task for the current graph.
* This method synchronizes the physical storage with the current in-memory graph.
*
* @param callback called when the save is finished. The parameter specifies whether or not the task succeeded.
*/
void save(Callback callback);
/**
* Connects the current graph to its storage (mandatory before any other method call)
*
* @param callback Called when the connection is done. The parameter specifies whether or not the connection succeeded.
*/
void connect(Callback callback);
/**
* Disconnects the current graph from its storage (a save will be trigger safely before the exit)
*
* @param callback Called when the disconnection is completed. The parameter specifies whether or not the disconnection succeeded.
*/
void disconnect(Callback callback);
/**
* Retrieve a named global index, at a precise world and time.
* Creates the index if it does not exist.
*
* @param world The world id in which the index has to be looked for
* @param time The time at which the index has to be looked for
* @param name The name of the index
* @param callback The callback to be called when the index lookup is complete.
*/
void index(long world, long time, String name, Callback callback);
/**
* Retrieve a named global index, at a precise world and time.
* Returns null to the callback if it does not exist.
*
* @param world The world id in which the index has to be looked for
* @param time The time at which the index has to be looked for
* @param name The name of the index
* @param callback The callback to be called when the index lookup is complete.
*/
void indexIfExists(long world, long time, String name, Callback callback);
/**
* Retrieve the list of indexes.
*
* @param world The world id in which the search must be performed.
* @param time The timepoint at which the search must be performed.
* @param callback Called when the retrieval is complete. Returns the retrieved indexes names, empty array otherwise.
*/
void indexNames(long world, long time, Callback callback);
/**
* Utility method to create a waiter based on a counter
*
* @param expectedEventsCount number of events expected before running a task.
* @return The waiter object.
*/
DeferCounter newCounter(int expectedEventsCount);
/**
* Utility method to create a sync waiter based on a counter
*
* @param expectedEventsCount number of events expected before running a task.
* @return The waiter object.
*/
DeferCounterSync newSyncCounter(int expectedEventsCount);
/**
* Retrieves the current resolver
*
* @return current resolver
*/
Resolver resolver();
/**
* Retrieves the current scheduler
*
* @return current scheduler
*/
Scheduler scheduler();
/**
* Retrieves the current space
*
* @return current space
*/
ChunkSpace space();
/**
* Retrieves the current storage
*
* @return current storage
*/
Storage storage();
/**
* Creates a new buffer for serialization and loading methods
*
* @return newly created buffer
*/
Buffer newBuffer();
/**
* Creates a new query that can be executed on the graph.
*
* @return newly created query
*/
Query newQuery();
/**
* Free the array of nodes (sequentially call the free method on all nodes)
*
* @param nodes the array of nodes to free
*/
void freeNodes(Node[] nodes);
/**
* Retrieve the default task hook factory
*
* @return the current default task hook factory
*/
TaskHook[] taskHooks();
ActionRegistry actionRegistry();
NodeRegistry nodeRegistry();
Graph setMemoryFactory(MemoryFactory factory);
Graph addGlobalTaskHook(TaskHook taskHook);
}