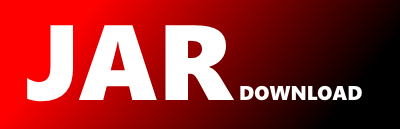
jsyntaxpane.lexers.XHTMLLexer Maven / Gradle / Ivy
/* The following code was generated by JFlex 1.4.3 on 12/10/15 17:13 */
/*
* Copyright 2008 Ayman Al-Sairafi [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License
* at http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jsyntaxpane.lexers;
import jsyntaxpane.Token;
import jsyntaxpane.TokenType;
/**
* This class is a scanner generated by
* JFlex 1.4.3
* on 12/10/15 17:13 from the specification file
* /home/leiko/dev/kevoree/kevoree/target/checkout/kevoree-tools/org.kevoree.tools.ui.kevscript/src/main/jflex/jsyntaxpane/lexers/xhtml.flex
*/
public final class XHTMLLexer extends DefaultJFlexLexer {
/** This character denotes the end of file */
public static final int YYEOF = -1;
/** initial size of the lookahead buffer */
private static final int ZZ_BUFFERSIZE = 16384;
/** lexical states */
public static final int INSTR = 8;
public static final int YYINITIAL = 0;
public static final int COMMENT = 2;
public static final int CDATA = 4;
public static final int TAG = 6;
public static final int DOCTYPE = 10;
/**
* ZZ_LEXSTATE[l] is the state in the DFA for the lexical state l
* ZZ_LEXSTATE[l+1] is the state in the DFA for the lexical state l
* at the beginning of a line
* l is of the form l = 2*k, k a non negative integer
*/
private static final int ZZ_LEXSTATE[] = {
0, 0, 1, 1, 2, 2, 3, 3, 4, 4, 5, 5
};
/**
* Translates characters to character classes
*/
private static final String ZZ_CMAP_PACKED =
"\11\0\1\1\1\2\2\0\1\2\22\0\1\1\1\4\1\104\1\110"+
"\2\0\1\106\1\105\5\0\1\5\1\12\1\37\1\13\6\75\3\13"+
"\1\7\1\107\1\3\1\40\1\6\1\14\1\0\1\34\1\47\1\21"+
"\1\15\1\31\1\51\1\57\1\73\1\55\1\10\1\61\1\45\1\71"+
"\1\53\1\17\1\27\1\63\1\41\1\43\1\23\1\65\1\67\1\100"+
"\1\76\1\25\1\102\1\33\1\0\1\36\1\0\1\7\1\0\1\35"+
"\1\50\1\22\1\16\1\32\1\52\1\60\1\74\1\56\1\11\1\62"+
"\1\46\1\72\1\54\1\20\1\30\1\64\1\42\1\44\1\24\1\66"+
"\1\70\1\101\1\77\1\26\1\103\74\0\1\12\u05a8\0\12\111\206\0"+
"\12\111\306\0\12\111\u019c\0\12\111\166\0\12\111\166\0\12\111\166\0"+
"\12\111\166\0\12\111\166\0\12\111\166\0\12\111\166\0\12\111\166\0"+
"\12\111\340\0\12\111\166\0\12\111\106\0\12\111\u0116\0\12\111\106\0"+
"\12\111\u0746\0\12\111\46\0\12\111\u012c\0\12\111\200\0\12\111\246\0"+
"\12\111\6\0\12\111\266\0\12\111\126\0\12\111\206\0\12\111\6\0"+
"\12\111\u89c6\0\12\111\u02a6\0\12\111\46\0\12\111\306\0\12\111\166\0"+
"\12\111\u0196\0\12\111\u5316\0\12\111\346\0";
/**
* Translates characters to character classes
*/
private static final char [] ZZ_CMAP = zzUnpackCMap(ZZ_CMAP_PACKED);
/**
* Translates DFA states to action switch labels.
*/
private static final int [] ZZ_ACTION = zzUnpackAction();
private static final String ZZ_ACTION_PACKED_0 =
"\5\0\10\1\1\2\32\1\1\3\1\0\1\4\1\0"+
"\4\4\2\5\1\0\2\4\1\5\11\4\11\0\1\6"+
"\23\0\1\7\47\0\1\10\4\0\1\11\1\5\17\4"+
"\23\0\6\4\1\5\16\4\1\12\2\0\1\13\1\0"+
"\1\14\72\0\1\15\2\0\10\4\1\0\1\16\14\0"+
"\1\17\32\0\16\4\1\20\55\0\5\4\26\0\3\4"+
"\1\5\2\4\41\0\2\4\13\0\3\4\21\0\1\4"+
"\5\0\3\4\16\0\1\4\2\0\1\21\1\22\1\0";
private static int [] zzUnpackAction() {
int [] result = new int[498];
int offset = 0;
offset = zzUnpackAction(ZZ_ACTION_PACKED_0, offset, result);
return result;
}
private static int zzUnpackAction(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/**
* Translates a state to a row index in the transition table
*/
private static final int [] ZZ_ROWMAP = zzUnpackRowMap();
private static final String ZZ_ROWMAP_PACKED_0 =
"\0\0\0\112\0\224\0\336\0\u0128\0\u0172\0\u01bc\0\u0206"+
"\0\u0250\0\u029a\0\u02e4\0\u032e\0\u0378\0\u01bc\0\u03c2\0\u040c"+
"\0\u0456\0\u04a0\0\u04ea\0\u0534\0\u057e\0\u05c8\0\u0612\0\u065c"+
"\0\u06a6\0\u06f0\0\u073a\0\u0784\0\u07ce\0\u0818\0\u0862\0\u08ac"+
"\0\u08f6\0\u0940\0\u098a\0\u09d4\0\u0a1e\0\u0a68\0\u0ab2\0\u0afc"+
"\0\u01bc\0\u0b46\0\u0b90\0\u0bda\0\u0c24\0\u0c6e\0\u0cb8\0\u0d02"+
"\0\u0d4c\0\u0d96\0\u0de0\0\u0e2a\0\u0e74\0\u0ebe\0\u0f08\0\u0f52"+
"\0\u0f9c\0\u0fe6\0\u1030\0\u107a\0\u10c4\0\u110e\0\u1158\0\u11a2"+
"\0\u11ec\0\u029a\0\u1236\0\u1280\0\u032e\0\u12ca\0\u1314\0\u03c2"+
"\0\u01bc\0\u135e\0\u13a8\0\u13f2\0\u143c\0\u1486\0\u14d0\0\u151a"+
"\0\u1564\0\u15ae\0\u15f8\0\u1642\0\u168c\0\u16d6\0\u1720\0\u176a"+
"\0\u17b4\0\u17fe\0\u1848\0\u1892\0\u01bc\0\u18dc\0\u1926\0\u1970"+
"\0\u19ba\0\u1a04\0\u1a4e\0\u1a98\0\u1ae2\0\u1b2c\0\u1b76\0\u1bc0"+
"\0\u1c0a\0\u1c54\0\u1c9e\0\u1ce8\0\u1d32\0\u1d7c\0\u1dc6\0\u1e10"+
"\0\u1e5a\0\u1ea4\0\u1eee\0\u1f38\0\u1f82\0\u1fcc\0\u2016\0\u2060"+
"\0\u20aa\0\u20f4\0\u213e\0\u2188\0\u21d2\0\u221c\0\u2266\0\u22b0"+
"\0\u22fa\0\u2344\0\u238e\0\u0a1e\0\u01bc\0\u0a68\0\u23d8\0\u2422"+
"\0\u246c\0\u24b6\0\u0b90\0\u2500\0\u254a\0\u2594\0\u25de\0\u2628"+
"\0\u2672\0\u26bc\0\u2706\0\u2750\0\u279a\0\u27e4\0\u282e\0\u2878"+
"\0\u28c2\0\u290c\0\u2956\0\u29a0\0\u29ea\0\u2a34\0\u2a7e\0\u2ac8"+
"\0\u2b12\0\u2b5c\0\u2ba6\0\u2bf0\0\u2c3a\0\u2c84\0\u2cce\0\u2d18"+
"\0\u2d62\0\u2dac\0\u2df6\0\u2e40\0\u2e8a\0\u2ed4\0\u2f1e\0\u2f68"+
"\0\u2fb2\0\u2ffc\0\u3046\0\u3090\0\u30da\0\u3124\0\u316e\0\u31b8"+
"\0\u3202\0\u324c\0\u3296\0\u32e0\0\u332a\0\u3374\0\u33be\0\u3408"+
"\0\u3452\0\u349c\0\u01bc\0\u34e6\0\u3530\0\u01bc\0\u357a\0\u01bc"+
"\0\u35c4\0\u360e\0\u3658\0\u36a2\0\u36ec\0\u3736\0\u3780\0\u37ca"+
"\0\u3814\0\u385e\0\u38a8\0\u38f2\0\u393c\0\u3986\0\u39d0\0\u3a1a"+
"\0\u3a64\0\u3aae\0\u3af8\0\u3b42\0\u3b8c\0\u3bd6\0\u3c20\0\u3c6a"+
"\0\u3cb4\0\u3cfe\0\u3d48\0\u3d92\0\u3ddc\0\u3e26\0\u3e70\0\u3eba"+
"\0\u3f04\0\u3f4e\0\u3f98\0\u3fe2\0\u402c\0\u4076\0\u40c0\0\u410a"+
"\0\u4154\0\u419e\0\u41e8\0\u4232\0\u427c\0\u42c6\0\u4310\0\u435a"+
"\0\u43a4\0\u43ee\0\u4438\0\u4482\0\u44cc\0\u4516\0\u4560\0\u45aa"+
"\0\u45f4\0\u463e\0\u01bc\0\u4688\0\u46d2\0\u471c\0\u4766\0\u47b0"+
"\0\u47fa\0\u4844\0\u488e\0\u48d8\0\u4922\0\u496c\0\u01bc\0\u49b6"+
"\0\u4a00\0\u4a4a\0\u4a94\0\u4ade\0\u4b28\0\u4b72\0\u4bbc\0\u4c06"+
"\0\u4c50\0\u4c9a\0\u4ce4\0\u01bc\0\u4d2e\0\u4d78\0\u4dc2\0\u4e0c"+
"\0\u4e56\0\u4ea0\0\u4eea\0\u4f34\0\u4f7e\0\u4fc8\0\u5012\0\u505c"+
"\0\u50a6\0\u50f0\0\u513a\0\u5184\0\u51ce\0\u5218\0\u5262\0\u52ac"+
"\0\u52f6\0\u5340\0\u538a\0\u53d4\0\u541e\0\u5468\0\u54b2\0\u54fc"+
"\0\u5546\0\u5590\0\u55da\0\u5624\0\u566e\0\u56b8\0\u5702\0\u574c"+
"\0\u5796\0\u57e0\0\u582a\0\u5874\0\u01bc\0\u58be\0\u5908\0\u5952"+
"\0\u599c\0\u59e6\0\u5a30\0\u5a7a\0\u5ac4\0\u5b0e\0\u5b58\0\u5ba2"+
"\0\u5bec\0\u5c36\0\u5c80\0\u5cca\0\u5d14\0\u5d5e\0\u5da8\0\u5df2"+
"\0\u5e3c\0\u5e86\0\u5ed0\0\u5f1a\0\u5f64\0\u5fae\0\u5ff8\0\u6042"+
"\0\u608c\0\u60d6\0\u6120\0\u616a\0\u61b4\0\u61fe\0\u6248\0\u6292"+
"\0\u62dc\0\u6326\0\u6370\0\u63ba\0\u6404\0\u644e\0\u6498\0\u64e2"+
"\0\u652c\0\u6576\0\u65c0\0\u660a\0\u6654\0\u669e\0\u66e8\0\u6732"+
"\0\u677c\0\u67c6\0\u6810\0\u685a\0\u68a4\0\u68ee\0\u6938\0\u6982"+
"\0\u69cc\0\u6a16\0\u6a60\0\u6aaa\0\u6af4\0\u6b3e\0\u6b88\0\u6bd2"+
"\0\u6c1c\0\u6c66\0\u6cb0\0\u6cfa\0\u6d44\0\u6d8e\0\u6dd8\0\u6e22"+
"\0\u6e6c\0\u6eb6\0\u6f00\0\u6f4a\0\u6f94\0\u6fde\0\u7028\0\u7072"+
"\0\u70bc\0\u7106\0\u7150\0\u719a\0\u71e4\0\u722e\0\u7278\0\u72c2"+
"\0\u730c\0\u7356\0\u73a0\0\u73ea\0\u7434\0\u747e\0\u74c8\0\u7512"+
"\0\u755c\0\u75a6\0\u75f0\0\u763a\0\u7684\0\u76ce\0\u7718\0\u7762"+
"\0\u77ac\0\u77f6\0\u7840\0\u788a\0\u78d4\0\u791e\0\u7968\0\u79b2"+
"\0\u79fc\0\u7a46\0\u7a90\0\u7ada\0\u7b24\0\u7b6e\0\u7bb8\0\u7c02"+
"\0\u7c4c\0\u7c96\0\u7ce0\0\u7d2a\0\u7d74\0\u7dbe\0\u7e08\0\u7e52"+
"\0\u7e9c\0\u7ee6\0\u7f30\0\u7f7a\0\u7fc4\0\u800e\0\u8058\0\u80a2"+
"\0\u80ec\0\u8136\0\u8180\0\u81ca\0\u8214\0\u825e\0\u82a8\0\u82f2"+
"\0\u833c\0\u8386\0\u83d0\0\u841a\0\u8464\0\u84ae\0\u84f8\0\u8542"+
"\0\u858c\0\u85d6\0\u8620\0\u866a\0\u86b4\0\u86fe\0\u8748\0\u8792"+
"\0\u87dc\0\u8826\0\u8870\0\u88ba\0\u8904\0\u894e\0\u8998\0\u01bc"+
"\0\u01bc\0\u89e2";
private static int [] zzUnpackRowMap() {
int [] result = new int[498];
int offset = 0;
offset = zzUnpackRowMap(ZZ_ROWMAP_PACKED_0, offset, result);
return result;
}
private static int zzUnpackRowMap(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int high = packed.charAt(i++) << 16;
result[j++] = high | packed.charAt(i++);
}
return j;
}
/**
* The transition table of the DFA
*/
private static final int [] ZZ_TRANS = zzUnpackTrans();
private static final String ZZ_TRANS_PACKED_0 =
"\3\7\1\10\102\7\1\11\3\7\5\12\1\13\104\12"+
"\36\14\1\15\53\14\6\7\1\16\3\17\3\7\2\20"+
"\2\21\2\22\2\23\2\17\2\24\2\25\1\7\2\26"+
"\1\7\1\27\1\7\2\30\2\31\2\32\2\33\2\34"+
"\2\35\2\36\2\37\4\17\2\40\2\41\2\42\2\43"+
"\1\7\2\17\2\44\2\17\1\45\1\46\13\7\3\17"+
"\2\7\1\47\16\17\1\7\2\17\3\7\34\17\1\7"+
"\6\17\1\45\1\46\4\7\6\50\1\51\103\50\116\0"+
"\1\52\2\0\3\53\2\0\1\54\2\55\2\56\2\57"+
"\2\60\2\53\2\61\2\53\1\0\2\62\1\0\1\63"+
"\1\0\2\53\2\64\2\65\2\66\2\67\2\70\2\71"+
"\2\53\2\72\2\53\2\73\2\74\2\75\2\76\1\0"+
"\2\77\4\53\17\0\1\100\4\0\1\100\1\0\1\100"+
"\1\0\1\100\1\0\1\100\1\0\1\100\1\0\1\100"+
"\1\0\1\100\2\0\1\100\4\0\1\100\1\0\1\100"+
"\1\0\1\100\1\0\1\100\1\0\1\100\1\0\1\100"+
"\1\0\1\100\1\0\1\100\1\0\1\100\1\0\1\100"+
"\1\0\1\100\1\0\1\100\1\0\1\100\1\0\1\100"+
"\2\0\1\100\1\0\1\100\1\0\1\100\4\0\1\101"+
"\1\0\5\102\1\103\111\102\1\104\104\102\36\105\1\106"+
"\111\105\1\107\53\105\5\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\14\110\2\112\1\0\2\113\2\0"+
"\1\111\14\110\2\114\25\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\6\110\2\115"+
"\33\110\13\0\1\110\1\0\5\110\1\0\2\110\2\116"+
"\10\110\2\117\1\0\2\110\2\0\1\111\4\110\2\120"+
"\6\110\2\121\14\110\2\122\7\110\13\0\1\110\1\0"+
"\5\110\1\0\10\110\2\123\2\110\2\124\1\0\2\125"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\2\126\41\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\12\110\2\127\27\110\13\0\1\110\1\0\5\110"+
"\1\0\4\110\2\130\10\110\1\0\2\110\2\0\1\111"+
"\2\131\2\110\2\132\2\133\25\110\2\134\4\110\14\0"+
"\1\135\110\0\1\110\1\0\5\110\1\0\2\110\2\136"+
"\10\110\2\137\1\0\2\110\2\0\1\111\24\110\2\140"+
"\15\110\13\0\1\110\1\0\5\110\1\0\4\110\2\141"+
"\2\142\2\110\2\143\2\144\1\0\2\110\2\0\1\111"+
"\2\145\12\110\2\146\6\110\2\147\4\110\2\150\7\110"+
"\13\0\1\110\1\0\5\110\1\0\2\110\2\151\12\110"+
"\1\0\2\152\2\0\1\111\14\110\2\153\25\110\13\0"+
"\1\110\1\0\5\110\1\0\2\110\2\154\12\110\1\0"+
"\2\155\2\0\1\111\16\110\2\156\23\110\13\0\1\110"+
"\1\0\5\110\1\0\2\110\2\157\12\110\1\0\2\160"+
"\2\0\1\111\2\161\41\110\13\0\1\110\1\0\5\110"+
"\1\0\2\110\2\162\12\110\1\0\2\163\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\2\164\14\110"+
"\1\0\2\110\2\0\1\111\2\110\2\165\37\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\24\110\2\166\15\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\2\167\2\170"+
"\37\110\13\0\1\110\1\0\5\110\1\0\14\110\2\171"+
"\1\0\2\172\2\0\1\111\2\110\2\173\2\174\35\110"+
"\13\0\1\110\1\0\5\110\1\0\14\110\2\175\1\0"+
"\2\176\2\0\1\111\24\110\2\177\15\110\13\0\1\110"+
"\1\0\5\110\1\0\14\110\2\200\1\0\2\110\2\0"+
"\1\111\2\201\2\173\37\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\2\202\12\110"+
"\2\203\25\110\6\0\2\204\1\0\101\204\1\205\5\204"+
"\2\206\1\0\102\206\1\205\4\206\6\0\1\51\103\0"+
"\6\50\1\0\103\50\5\0\1\207\7\0\2\210\14\0"+
"\1\211\63\0\1\53\1\0\5\53\1\0\16\53\1\0"+
"\2\53\3\0\43\53\15\0\3\212\3\0\16\212\1\0"+
"\2\212\3\0\34\212\1\0\6\212\13\0\1\53\1\0"+
"\5\53\1\0\2\213\4\53\2\213\6\53\1\0\2\53"+
"\3\0\4\53\2\213\2\53\2\214\2\53\2\215\25\53"+
"\13\0\1\53\1\0\5\53\1\0\12\53\2\216\2\53"+
"\1\0\2\53\3\0\4\53\2\213\35\53\13\0\1\53"+
"\1\0\5\53\1\0\2\53\2\217\10\53\2\220\1\0"+
"\2\221\3\0\14\53\2\222\25\53\13\0\1\53\1\0"+
"\5\53\1\0\2\213\4\53\2\213\4\53\2\223\1\0"+
"\2\224\3\0\2\213\12\53\2\225\14\53\2\213\7\53"+
"\13\0\1\53\1\0\5\53\1\0\16\53\1\0\2\226"+
"\3\0\2\227\41\53\13\0\1\53\1\0\5\53\1\0"+
"\2\230\10\53\2\231\2\53\1\0\2\53\3\0\2\232"+
"\41\53\15\0\3\233\3\0\2\234\2\235\2\236\2\237"+
"\2\233\2\240\2\233\1\0\2\241\3\0\2\233\2\242"+
"\2\243\2\244\2\245\2\246\2\247\2\233\2\250\2\233"+
"\2\251\2\252\2\253\2\254\1\0\2\255\4\233\13\0"+
"\1\53\1\0\5\53\1\0\4\53\2\256\2\257\2\53"+
"\2\260\2\261\1\0\2\77\3\0\24\53\2\262\2\53"+
"\2\263\11\53\13\0\1\53\1\0\5\53\1\0\16\53"+
"\1\0\2\53\3\0\14\53\2\264\25\53\13\0\1\53"+
"\1\0\5\53\1\0\2\53\2\265\12\53\1\0\2\266"+
"\3\0\2\213\2\53\2\267\6\53\2\270\25\53\13\0"+
"\1\53\1\0\5\53\1\0\2\53\2\271\12\53\1\0"+
"\2\53\3\0\43\53\13\0\1\53\1\0\5\53\1\0"+
"\2\53\2\272\12\53\1\0\2\53\3\0\43\53\13\0"+
"\1\53\1\0\5\53\1\0\16\53\1\0\2\53\3\0"+
"\2\53\2\273\6\53\2\274\14\53\2\270\11\53\13\0"+
"\1\53\1\0\5\53\1\0\16\53\1\0\2\53\3\0"+
"\6\53\2\275\33\53\13\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\4\53\2\213\35\53\13\0"+
"\1\53\1\0\5\53\1\0\16\53\1\0\2\276\3\0"+
"\43\53\13\0\1\53\1\0\5\53\1\0\14\53\2\277"+
"\1\0\2\300\3\0\43\53\13\0\1\53\1\0\5\53"+
"\1\0\6\53\2\301\4\53\2\302\1\0\2\53\3\0"+
"\2\213\32\53\1\213\6\53\13\0\1\53\1\0\5\53"+
"\1\0\16\53\1\0\2\53\3\0\30\53\2\300\11\53"+
"\17\0\1\100\4\0\1\100\1\0\1\100\1\0\1\100"+
"\1\0\1\100\1\0\1\100\1\0\1\100\1\0\1\100"+
"\2\0\1\100\4\0\1\100\1\0\1\100\1\0\1\100"+
"\1\0\1\100\1\0\1\100\1\0\1\100\1\0\1\100"+
"\1\0\1\100\1\0\1\100\1\0\1\100\1\0\1\100"+
"\1\0\1\100\1\0\1\100\1\0\1\100\2\0\1\100"+
"\1\0\1\100\1\0\1\100\3\0\1\303\15\0\1\304"+
"\61\0\1\304\13\0\1\304\5\102\1\305\111\102\1\305"+
"\1\306\103\102\36\105\1\307\61\105\1\310\27\105\1\307"+
"\53\105\5\0\1\110\1\0\5\110\1\0\4\110\2\311"+
"\10\110\1\0\2\110\2\0\1\111\10\110\2\312\31\110"+
"\13\0\1\110\1\0\5\110\1\0\6\110\2\313\6\110"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\2\164"+
"\2\314\37\110\13\0\1\110\1\0\1\110\2\315\2\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\43\110\13\0"+
"\1\110\1\0\5\110\1\0\2\316\2\317\12\110\1\0"+
"\2\110\2\0\1\111\4\110\2\320\4\110\2\321\14\110"+
"\2\322\11\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\4\110\2\323\35\110\13\0"+
"\1\110\1\0\5\110\1\0\14\110\2\324\1\0\2\325"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\6\110\2\326\6\110\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\14\110\2\327\1\0"+
"\2\330\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\12\110\2\326\2\110\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\35\110\2\331\4\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\2\332\4\110\2\333\33\110\13\0\1\110\1\0\5\110"+
"\1\0\2\110\2\334\12\110\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\4\110\2\335"+
"\10\110\1\0\2\110\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\4\110\2\336\2\337\6\110\1\0"+
"\2\110\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\4\110\2\340\10\110\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\6\110\2\164"+
"\6\110\1\0\2\110\2\0\1\111\14\110\2\341\25\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\6\110\2\157\33\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\14\110"+
"\2\342\25\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\37\110\2\343\2\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\344\2\0"+
"\1\111\4\110\2\164\20\110\2\164\13\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\4\110\2\345\35\110\13\0\1\110\1\0\5\110\1\0"+
"\2\110\2\123\12\110\1\0\2\110\2\0\1\111\2\346"+
"\30\110\2\347\7\110\13\0\1\110\1\0\5\110\1\0"+
"\10\110\2\350\4\110\1\0\2\351\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\352"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\4\110\2\353\35\110"+
"\13\0\1\110\1\0\5\110\1\0\4\110\2\164\10\110"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\41\110"+
"\2\326\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\30\110\2\354\11\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\123\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\12\110\2\355\23\110\2\356\2\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\12\110\2\357\27\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\12\110"+
"\2\360\27\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\2\361\41\110\13\0\1\110"+
"\1\0\5\110\1\0\4\110\2\362\10\110\1\0\2\110"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\4\110\2\363\10\110\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\2\164\41\110\13\0\1\110\1\0\5\110"+
"\1\0\4\110\2\326\10\110\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\163\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\32\110\2\364"+
"\3\110\2\365\2\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\30\110\2\326\11\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\303\43\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\30\110\2\202\11\110"+
"\13\0\1\110\1\0\5\110\1\0\6\110\2\366\6\110"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\4\110"+
"\2\164\35\110\13\0\1\110\1\0\5\110\1\0\14\110"+
"\2\165\1\0\2\110\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\2\367\41\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\4\110\2\370\35\110\13\0"+
"\1\110\1\0\5\110\1\0\12\110\2\371\2\110\1\0"+
"\2\110\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\14\110\2\153"+
"\25\110\13\0\1\110\1\0\5\110\1\0\6\110\2\372"+
"\6\110\1\0\2\110\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\2\373\33\110\2\374\4\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\4\110\2\375"+
"\35\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\376\2\0\1\111\14\110\2\377\25\110\13\0\1\110"+
"\1\0\5\110\1\0\14\110\2\u0100\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\u0101\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\2\u0102\14\110\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\u0103\123\0\2\u0104\112\0\2\u0105\74\0"+
"\1\212\1\0\5\212\1\0\16\212\1\0\2\212\3\0"+
"\43\212\13\0\1\53\1\0\5\53\1\0\16\53\1\0"+
"\2\53\3\0\12\53\2\213\27\53\13\0\1\53\1\0"+
"\5\53\1\0\16\53\1\0\2\53\3\0\2\213\24\53"+
"\2\213\13\53\13\0\1\53\1\0\5\53\1\0\6\53"+
"\2\u0106\6\53\1\0\2\53\3\0\43\53\13\0\1\53"+
"\1\0\5\53\1\0\2\227\14\53\1\0\2\53\3\0"+
"\43\53\13\0\1\53\1\0\5\53\1\0\16\53\1\0"+
"\2\53\3\0\12\53\2\u0107\27\53\13\0\1\53\1\0"+
"\5\53\1\0\12\53\2\216\2\53\1\0\2\53\3\0"+
"\43\53\13\0\1\53\1\0\5\53\1\0\6\53\2\227"+
"\6\53\1\0\2\53\3\0\43\53\13\0\1\53\1\0"+
"\5\53\1\0\16\53\1\0\2\53\3\0\35\53\2\u0108"+
"\4\53\13\0\1\53\1\0\5\53\1\0\16\53\1\0"+
"\2\53\3\0\6\53\2\u0109\33\53\13\0\1\53\1\0"+
"\5\53\1\0\6\53\2\u0109\6\53\1\0\2\53\3\0"+
"\43\53\13\0\1\53\1\0\5\53\1\0\16\53\1\0"+
"\2\53\3\0\2\u010a\41\53\13\0\1\53\1\0\5\53"+
"\1\0\14\53\2\213\1\0\2\53\3\0\43\53\13\0"+
"\1\53\1\0\5\53\1\0\2\u010b\14\53\1\0\2\53"+
"\3\0\43\53\13\0\1\53\1\0\5\53\1\0\12\53"+
"\2\u010c\2\53\1\0\2\53\3\0\43\53\13\0\1\53"+
"\1\0\5\53\1\0\14\53\2\u010d\1\0\2\53\3\0"+
"\43\53\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\16\233\1\0\2\233\3\0\43\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\2\u0110\4\233\2\u0110\6\233"+
"\1\0\2\233\3\0\4\233\2\u0110\2\233\2\u0111\2\233"+
"\2\u0112\25\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\12\233\2\u0113\2\233\1\0\2\233\3\0\4\233"+
"\2\u0110\35\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\2\233\2\u0114\10\233\2\u0115\1\0\2\u0116\3\0"+
"\14\233\2\u0117\25\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\2\u0110\4\233\2\u0110\4\233\2\u0118\1\0"+
"\2\u0119\3\0\2\u0110\12\233\2\u011a\14\233\2\u0110\7\233"+
"\7\0\2\u011b\2\0\1\233\1\u011c\5\233\1\0\16\233"+
"\1\0\2\u011d\3\0\2\u011e\41\233\7\0\2\u011b\2\0"+
"\1\233\1\u011c\5\233\1\0\2\u011f\10\233\2\u0120\2\233"+
"\1\0\2\233\3\0\2\u0121\41\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\4\233\2\u0122\2\u0123\2\233"+
"\2\u0124\2\u0125\1\0\2\255\3\0\24\233\2\u0126\2\233"+
"\2\u0127\11\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\16\233\1\0\2\233\3\0\14\233\2\u0128\25\233"+
"\7\0\2\u011b\2\0\1\233\1\u011c\5\233\1\0\2\233"+
"\2\u0129\12\233\1\0\2\u012a\3\0\2\u0110\2\233\2\u012b"+
"\6\233\2\u012c\25\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\2\233\2\u012d\12\233\1\0\2\233\3\0"+
"\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\2\233\2\u012e\12\233\1\0\2\233\3\0\43\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0"+
"\2\233\3\0\2\233\2\u012f\6\233\2\u0130\14\233\2\u012c"+
"\11\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\16\233\1\0\2\233\3\0\6\233\2\u0131\33\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0"+
"\2\233\3\0\4\233\2\u0110\35\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\16\233\1\0\2\u0132\3\0"+
"\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\14\233\2\u0133\1\0\2\u0134\3\0\43\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\6\233\2\u0135\4\233"+
"\2\u0136\1\0\2\233\3\0\2\u0110\32\233\1\u0110\6\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233"+
"\1\0\2\233\3\0\30\233\2\u0134\11\233\13\0\1\53"+
"\1\0\5\53\1\0\16\53\1\0\2\53\3\0\2\u0137"+
"\41\53\13\0\1\53\1\0\5\53\1\0\10\53\2\u0109"+
"\4\53\1\0\2\53\3\0\2\u0138\41\53\13\0\1\53"+
"\1\0\5\53\1\0\16\53\1\0\2\214\3\0\43\53"+
"\13\0\1\53\1\0\5\53\1\0\16\53\1\0\2\53"+
"\3\0\4\53\2\u0139\35\53\13\0\1\53\1\0\5\53"+
"\1\0\12\53\2\213\2\53\1\0\2\53\3\0\6\53"+
"\2\213\33\53\13\0\1\53\1\0\5\53\1\0\16\53"+
"\1\0\2\u013a\3\0\43\53\13\0\1\53\1\0\5\53"+
"\1\0\16\53\1\0\2\53\3\0\12\53\2\u013b\27\53"+
"\13\0\1\53\1\0\5\53\1\0\2\u013c\14\53\1\0"+
"\2\53\3\0\43\53\13\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\2\53\2\u013d\37\53\13\0"+
"\1\53\1\0\5\53\1\0\2\53\2\u013e\12\53\1\0"+
"\2\53\3\0\43\53\13\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\16\53\2\213\23\53\13\0"+
"\1\53\1\0\5\53\1\0\16\53\1\0\2\53\3\0"+
"\2\u013f\10\53\2\u0140\27\53\13\0\1\53\1\0\5\53"+
"\1\0\16\53\1\0\2\53\3\0\2\53\2\u0141\37\53"+
"\13\0\1\53\1\0\5\53\1\0\16\53\1\0\2\53"+
"\3\0\14\53\2\u0142\25\53\13\0\1\53\1\0\5\53"+
"\1\0\12\53\2\u0143\2\53\1\0\2\53\3\0\43\53"+
"\13\0\1\53\1\0\5\53\1\0\2\213\14\53\1\0"+
"\2\53\3\0\43\53\13\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\2\213\41\53\13\0\1\53"+
"\1\0\5\53\1\0\6\53\2\u010d\6\53\1\0\2\53"+
"\3\0\12\53\2\u0144\27\53\13\0\1\53\1\0\5\53"+
"\1\0\12\53\2\213\2\53\1\0\2\53\3\0\43\53"+
"\13\0\1\53\1\0\5\53\1\0\16\53\1\0\2\53"+
"\3\0\30\53\2\73\11\53\13\0\1\53\1\0\5\53"+
"\1\0\16\53\1\0\2\275\3\0\43\53\21\0\1\304"+
"\61\0\1\304\11\0\1\303\1\0\1\304\5\102\1\305"+
"\1\u0145\103\102\6\105\1\u0145\27\105\1\307\53\105\5\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\4\110\2\u0146\35\110\13\0\1\110\1\0\5\110"+
"\1\0\14\110\2\157\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\14\110\2\u0147\1\0"+
"\2\164\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\u0148\2\0\1\111\43\110\13\0"+
"\1\110\1\0\5\110\1\0\14\110\2\u0149\1\0\2\110"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\14\110\2\u014a\1\0\2\110\2\0\1\111\43\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\2\u014b\41\110\13\0\1\110\1\0\5\110\1\0"+
"\2\110\2\157\12\110\1\0\2\110\2\0\1\111\2\110"+
"\2\u014c\37\110\13\0\1\110\1\0\5\110\1\0\6\110"+
"\2\u014d\6\110\1\0\2\110\2\0\1\111\43\110\13\0"+
"\1\110\1\0\5\110\1\0\12\110\2\u014e\2\110\1\0"+
"\2\110\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\4\110\2\u014f"+
"\35\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\157\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\2\110\2\u0150"+
"\37\110\13\0\1\110\1\0\5\110\1\0\14\110\2\164"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\4\110\2\u0151\10\110\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\2\u0152\41\110\13\0\1\110"+
"\1\0\5\110\1\0\6\110\2\164\6\110\1\0\2\110"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\16\110\2\u0153\23\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\14\110\2\u0154\25\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\10\110"+
"\2\u0155\16\110\2\u0156\11\110\13\0\1\110\1\0\5\110"+
"\1\0\6\110\2\u0157\6\110\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\14\110\2\u0158"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\14\110"+
"\2\u0159\25\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\32\110\2\u015a\7\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\12\110\2\360\2\110\2\352\23\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\2\110\2\164\37\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\2\110\2\u014c\37\110"+
"\13\0\1\110\1\0\5\110\1\0\2\u015b\14\110\1\0"+
"\2\110\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\14\110\2\342\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\2\110\2\u015c\12\110"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\14\110\2\163\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\4\110\2\326\35\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\2\331\10\110\2\u015d\27\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\12\110\2\164"+
"\27\110\13\0\1\110\1\0\5\110\1\0\14\110\2\u015e"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\30\110"+
"\2\u015f\11\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\16\110\2\u0160\23\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\2\110\2\u0161\37\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\16\110\2\u0162"+
"\23\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\20\110\2\164\21\110\13\0\1\110"+
"\1\0\5\110\1\0\2\u0163\14\110\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\20\110\2\u0164\21\110\13\0"+
"\1\110\1\0\5\110\1\0\2\110\2\u0165\12\110\1\0"+
"\2\110\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\2\u0166\41\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\2\202\41\110\13\0\1\110\1\0\5\110"+
"\1\0\6\110\2\312\6\110\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\2\110\2\337\37\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\14\110\2\u0167\6\110\2\u0168\15\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\160\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\32\110\2\u0169\7\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\16\110"+
"\2\u016a\23\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\4\110\2\u016b\35\110\13\0"+
"\1\110\1\0\5\110\1\0\6\110\2\u016c\6\110\1\0"+
"\2\110\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\2\u016d\14\110\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\16\110\2\u016e\23\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\10\110"+
"\2\u016f\31\110\13\0\1\110\1\0\5\110\1\0\12\110"+
"\2\164\2\110\1\0\2\110\2\0\1\111\43\110\13\0"+
"\1\110\1\0\5\110\1\0\6\110\2\u0170\6\110\1\0"+
"\2\110\2\0\1\111\43\110\27\0\2\u0171\104\0\2\u0172"+
"\100\0\1\53\1\0\5\53\1\0\16\53\1\0\2\53"+
"\3\0\14\53\2\u0173\25\53\13\0\1\53\1\0\5\53"+
"\1\0\6\53\2\u0174\6\53\1\0\2\53\3\0\43\53"+
"\13\0\1\53\1\0\5\53\1\0\6\53\2\u0175\6\53"+
"\1\0\2\53\3\0\43\53\13\0\1\53\1\0\5\53"+
"\1\0\16\53\1\0\2\53\3\0\4\53\2\227\35\53"+
"\13\0\1\53\1\0\5\53\1\0\16\53\1\0\2\u013f"+
"\3\0\43\53\13\0\1\53\1\0\5\53\1\0\16\53"+
"\1\0\2\53\3\0\2\u0176\41\53\13\0\1\53\1\0"+
"\5\53\1\0\16\53\1\0\2\53\3\0\4\53\2\u0177"+
"\35\53\13\0\1\53\1\0\5\53\1\0\16\53\1\0"+
"\2\213\3\0\43\53\7\0\2\u010e\3\0\1\u010f\104\0"+
"\2\u011b\2\0\1\233\1\u011c\5\233\1\0\16\233\1\0"+
"\2\233\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\16\233\1\0\2\233\3\0\12\233\2\u0110"+
"\27\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\16\233\1\0\2\233\3\0\2\u0110\24\233\2\u0110\13\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\6\233"+
"\2\u0178\6\233\1\0\2\233\3\0\43\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\2\u011e\14\233\1\0"+
"\2\233\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\16\233\1\0\2\233\3\0\12\233\2\u0179"+
"\27\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\12\233\2\u0113\2\233\1\0\2\233\3\0\43\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\6\233\2\u011e"+
"\6\233\1\0\2\233\3\0\43\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\16\233\1\0\2\233\3\0"+
"\35\233\2\u017a\4\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\16\233\1\0\2\233\3\0\6\233\2\u017b"+
"\33\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\6\233\2\u017b\6\233\1\0\2\233\3\0\43\233\7\0"+
"\2\u011b\3\0\1\u011c\104\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\16\233\1\0\2\233\3\0\2\u017c\41\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\14\233"+
"\2\u0110\1\0\2\233\3\0\43\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\2\u017d\14\233\1\0\2\233"+
"\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\12\233\2\u017e\2\233\1\0\2\233\3\0\43\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\14\233"+
"\2\u017f\1\0\2\233\3\0\43\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\16\233\1\0\2\233\3\0"+
"\2\u0180\41\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\10\233\2\u017b\4\233\1\0\2\233\3\0\2\u0181"+
"\41\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\16\233\1\0\2\u0111\3\0\43\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\16\233\1\0\2\233\3\0"+
"\4\233\2\u0182\35\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\12\233\2\u0110\2\233\1\0\2\233\3\0"+
"\6\233\2\u0110\33\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\16\233\1\0\2\u0183\3\0\43\233\7\0"+
"\2\u011b\2\0\1\233\1\u011c\5\233\1\0\16\233\1\0"+
"\2\233\3\0\12\233\2\u0184\27\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\2\u0185\14\233\1\0\2\233"+
"\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\16\233\1\0\2\233\3\0\2\233\2\u0186\37\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\2\233"+
"\2\u0187\12\233\1\0\2\233\3\0\43\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0\2\233"+
"\3\0\16\233\2\u0110\23\233\7\0\2\u010e\2\0\1\233"+
"\1\u010f\5\233\1\0\16\233\1\0\2\233\3\0\2\u0188"+
"\10\233\2\u0189\27\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\16\233\1\0\2\233\3\0\2\233\2\u018a"+
"\37\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\16\233\1\0\2\233\3\0\14\233\2\u018b\25\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\12\233\2\u018c"+
"\2\233\1\0\2\233\3\0\43\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\2\u0110\14\233\1\0\2\233"+
"\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\16\233\1\0\2\233\3\0\2\u0110\41\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\6\233\2\u017f"+
"\6\233\1\0\2\233\3\0\12\233\2\u018d\27\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\12\233\2\u0110"+
"\2\233\1\0\2\233\3\0\43\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\16\233\1\0\2\233\3\0"+
"\30\233\2\251\11\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\16\233\1\0\2\u0131\3\0\43\233\13\0"+
"\1\53\1\0\5\53\1\0\16\53\1\0\2\53\3\0"+
"\14\53\2\u018e\25\53\13\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\14\53\2\u018f\25\53\13\0"+
"\1\53\1\0\5\53\1\0\14\53\2\u0190\1\0\2\53"+
"\3\0\43\53\13\0\1\53\1\0\5\53\1\0\16\53"+
"\1\0\2\53\3\0\4\53\2\73\35\53\13\0\1\53"+
"\1\0\5\53\1\0\16\53\1\0\2\53\3\0\20\53"+
"\2\213\21\53\13\0\1\53\1\0\5\53\1\0\10\53"+
"\2\213\4\53\1\0\2\53\3\0\43\53\13\0\1\53"+
"\1\0\5\53\1\0\14\53\2\u0191\1\0\2\53\3\0"+
"\43\53\13\0\1\53\1\0\5\53\1\0\4\53\2\u0192"+
"\10\53\1\0\2\53\3\0\43\53\13\0\1\53\1\0"+
"\5\53\1\0\16\53\1\0\2\53\3\0\30\53\2\213"+
"\11\53\13\0\1\53\1\0\5\53\1\0\6\53\2\213"+
"\6\53\1\0\2\53\3\0\43\53\13\0\1\53\1\0"+
"\5\53\1\0\4\53\2\256\10\53\1\0\2\53\3\0"+
"\43\53\13\0\1\53\1\0\5\53\1\0\16\53\1\0"+
"\2\53\3\0\12\53\2\u0193\27\53\13\0\1\53\1\0"+
"\5\53\1\0\16\53\1\0\2\53\3\0\24\53\2\u0140"+
"\15\53\13\0\1\53\1\0\5\53\1\0\16\53\1\0"+
"\2\53\3\0\24\53\2\213\15\53\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\u0194\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\6\110\2\u0195\6\110"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\6\110"+
"\2\u0196\33\110\13\0\1\110\1\0\5\110\1\0\4\110"+
"\2\331\10\110\1\0\2\110\2\0\1\111\43\110\13\0"+
"\1\110\1\0\5\110\1\0\6\110\2\u0157\6\110\1\0"+
"\2\110\2\0\1\303\6\110\2\u0197\33\110\13\0\1\110"+
"\1\0\5\110\1\0\2\342\14\110\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\12\110"+
"\2\143\2\110\1\0\2\110\2\0\1\303\43\110\13\0"+
"\1\110\1\0\5\110\1\0\14\110\2\u0198\1\0\2\110"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\u0149\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\12\110\2\u0199\2\110\1\0\2\110"+
"\2\0\1\111\2\110\2\u019a\37\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\2\110"+
"\2\u019b\37\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\20\110\2\u019c\21\110\13\0"+
"\1\110\1\0\5\110\1\0\2\110\2\u019d\12\110\1\0"+
"\2\110\2\0\1\303\2\110\2\u0153\37\110\13\0\1\110"+
"\1\0\5\110\1\0\14\110\2\331\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\12\110\2\u019e\27\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\14\110\2\350\25\110\13\0\1\110\1\0\5\110"+
"\1\0\12\110\2\331\2\110\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\10\110\2\123"+
"\4\110\1\0\2\110\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\12\110\2\331\2\110\1\0\2\110"+
"\2\0\1\111\2\110\2\u019f\37\110\13\0\1\110\1\0"+
"\5\110\1\0\2\110\2\352\12\110\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\14\110\2\u01a0\25\110\13\0"+
"\1\110\1\0\5\110\1\0\2\110\2\u01a1\12\110\1\0"+
"\2\110\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\4\110\2\u01a2"+
"\35\110\13\0\1\110\1\0\5\110\1\0\2\u01a3\14\110"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\4\110\2\u01a4\10\110\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\u01a5\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\2\u01a6\14\110\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\2\145\41\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\303\24\110"+
"\2\u01a7\15\110\13\0\1\110\1\0\5\110\1\0\14\110"+
"\2\u01a8\1\0\2\110\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\16\110\2\u01a9\23\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\4\110\2\u01aa\35\110"+
"\13\0\1\110\1\0\5\110\1\0\14\110\2\u01ab\1\0"+
"\2\110\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\16\110\2\352"+
"\23\110\13\0\1\110\1\0\5\110\1\0\14\110\2\u01ac"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\2\110\2\u01ad\12\110\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\14\110\2\u01ae\25\110\13\0"+
"\1\110\1\0\5\110\1\0\14\110\2\u01af\1\0\2\110"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\14\110\2\u01b0\25\110"+
"\13\0\1\110\1\0\5\110\1\0\14\110\2\u01b1\1\0"+
"\2\110\2\0\1\111\43\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\32\110\2\331"+
"\7\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\303\4\110\2\u01b2\35\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\32\110\2\164\7\110\31\0\2\u01b3\121\0\2\u01b4\61\0"+
"\1\53\1\0\5\53\1\0\2\53\2\214\12\53\1\0"+
"\2\53\3\0\43\53\13\0\1\53\1\0\5\53\1\0"+
"\14\53\2\276\1\0\2\53\3\0\43\53\13\0\1\53"+
"\1\0\5\53\1\0\16\53\1\0\2\u01b5\3\0\43\53"+
"\13\0\1\53\1\0\5\53\1\0\14\53\2\u01b6\1\0"+
"\2\53\3\0\43\53\13\0\1\53\1\0\5\53\1\0"+
"\14\53\2\u0140\1\0\2\53\3\0\43\53\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0\2\233"+
"\3\0\14\233\2\u01b7\25\233\7\0\2\u010e\2\0\1\233"+
"\1\u010f\5\233\1\0\6\233\2\u01b8\6\233\1\0\2\233"+
"\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\6\233\2\u01b9\6\233\1\0\2\233\3\0\43\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233"+
"\1\0\2\233\3\0\4\233\2\u011e\35\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0\2\u0188"+
"\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\16\233\1\0\2\233\3\0\2\u01ba\41\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0"+
"\2\233\3\0\4\233\2\u01bb\35\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\16\233\1\0\2\u0110\3\0"+
"\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\16\233\1\0\2\233\3\0\14\233\2\u01bc\25\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0"+
"\2\233\3\0\14\233\2\u01bd\25\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\14\233\2\u01be\1\0\2\233"+
"\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\16\233\1\0\2\233\3\0\4\233\2\251\35\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233"+
"\1\0\2\233\3\0\20\233\2\u0110\21\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\10\233\2\u0110\4\233"+
"\1\0\2\233\3\0\43\233\7\0\2\u010e\2\0\1\233"+
"\1\u010f\5\233\1\0\14\233\2\u01bf\1\0\2\233\3\0"+
"\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\4\233\2\u01c0\10\233\1\0\2\233\3\0\43\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0"+
"\2\233\3\0\30\233\2\u0110\11\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\6\233\2\u0110\6\233\1\0"+
"\2\233\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\4\233\2\u0122\10\233\1\0\2\233\3\0"+
"\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0"+
"\16\233\1\0\2\233\3\0\12\233\2\u01c1\27\233\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0"+
"\2\233\3\0\24\233\2\u0189\15\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\16\233\1\0\2\233\3\0"+
"\24\233\2\u0110\15\233\13\0\1\53\1\0\5\53\1\0"+
"\12\53\2\u0140\2\53\1\0\2\53\3\0\43\53\13\0"+
"\1\53\1\0\5\53\1\0\16\53\1\0\2\53\3\0"+
"\20\53\2\227\21\53\13\0\1\53\1\0\5\53\1\0"+
"\4\53\2\u0140\10\53\1\0\2\53\3\0\43\53\13\0"+
"\1\53\1\0\5\53\1\0\16\53\1\0\2\53\3\0"+
"\10\53\2\u01c2\31\53\13\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\20\53\2\u01c3\21\53\13\0"+
"\1\53\1\0\5\53\1\0\2\u01c4\14\53\1\0\2\53"+
"\3\0\43\53\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\2\326\41\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\14\110\2\163\25\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\4\110\2\u019c\35\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\u01c5"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\12\110\2\331\27\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\u01c6"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\12\110\2\u01c7\2\110\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\303\14\110\2\u01ad\25\110\13\0\1\110\1\0"+
"\5\110\1\0\14\110\2\u01ad\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\10\110\2\u01ab\31\110\13\0\1\110"+
"\1\0\5\110\1\0\2\u01c8\14\110\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\2\110\2\u01c9\37\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\26\110\2\326\13\110\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\12\110\2\u01ca"+
"\27\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\4\110\2\u01cb\35\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\6\110\2\u01cc\33\110\13\0\1\110\1\0\5\110\1\0"+
"\6\110\2\u019c\6\110\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\2\u01cc\41\110\13\0\1\110\1\0\5\110"+
"\1\0\14\110\2\u01cd\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\u01ce"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\2\u01cf\41\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\2\u01d0\41\110\13\0\1\110\1\0\5\110\1\0"+
"\2\110\2\157\12\110\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\10\110\2\164\31\110\13\0\1\110\1\0"+
"\5\110\1\0\6\110\2\u0157\6\110\1\0\2\110\2\0"+
"\1\303\43\110\13\0\1\110\1\0\5\110\1\0\2\164"+
"\14\110\1\0\2\110\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\12\110\2\u01d1\27\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\12\110\2\u01d2\27\110"+
"\13\0\1\110\1\0\5\110\1\0\12\110\2\350\2\110"+
"\1\0\2\110\2\0\1\111\43\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\2\342"+
"\41\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\u01d3\2\0\1\111\43\110\33\0\2\u01d4\106\0\2\u01d5"+
"\72\0\1\53\1\0\5\53\1\0\16\53\1\0\2\53"+
"\3\0\2\232\41\53\13\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\2\53\2\u01d6\37\53\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\2\233\2\u0111"+
"\12\233\1\0\2\233\3\0\43\233\7\0\2\u010e\2\0"+
"\1\233\1\u010f\5\233\1\0\14\233\2\u0132\1\0\2\233"+
"\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\16\233\1\0\2\u01d7\3\0\43\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\14\233\2\u01d8\1\0"+
"\2\233\3\0\43\233\7\0\2\u010e\2\0\1\233\1\u010f"+
"\5\233\1\0\14\233\2\u0189\1\0\2\233\3\0\43\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\12\233"+
"\2\u0189\2\233\1\0\2\233\3\0\43\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0\2\233"+
"\3\0\20\233\2\u011e\21\233\7\0\2\u010e\2\0\1\233"+
"\1\u010f\5\233\1\0\4\233\2\u0189\10\233\1\0\2\233"+
"\3\0\43\233\7\0\2\u011b\2\0\1\233\1\u011c\5\233"+
"\1\0\16\233\1\0\2\233\3\0\10\233\2\u01d9\31\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233"+
"\1\0\2\233\3\0\20\233\2\u01da\21\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\2\u01db\14\233\1\0"+
"\2\233\3\0\43\233\13\0\1\53\1\0\5\53\1\0"+
"\2\53\2\u01dc\12\53\1\0\2\53\3\0\43\53\13\0"+
"\1\53\1\0\5\53\1\0\16\53\1\0\2\53\3\0"+
"\22\53\2\u01dd\17\53\13\0\1\53\1\0\5\53\1\0"+
"\14\53\2\u01de\1\0\2\53\3\0\43\53\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\2\110\2\326\37\110\13\0\1\110\1\0\5\110\1\0"+
"\2\u01df\14\110\1\0\2\110\2\0\1\111\43\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\u01e0\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\14\110"+
"\2\u01e1\1\0\2\110\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\20\110\2\u01e2\21\110\13\0\1\110\1\0\5\110\1\0"+
"\16\110\1\0\2\110\2\0\1\111\4\110\2\u01cc\35\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\14\110\2\u01d3\25\110\13\0\1\110\1\0"+
"\5\110\1\0\10\110\2\164\4\110\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\2\110\2\145\37\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\16\110\2\326\23\110\13\0\1\110\1\0\5\110"+
"\1\0\4\110\2\363\10\110\1\0\2\110\2\0\1\303"+
"\43\110\13\0\1\110\1\0\5\110\1\0\2\110\2\u01e3"+
"\12\110\1\0\2\110\2\0\1\111\43\110\13\0\1\110"+
"\1\0\5\110\1\0\16\110\1\0\2\110\2\0\1\111"+
"\32\110\2\u01e4\3\110\2\u01e5\2\110\13\0\1\110\1\0"+
"\5\110\1\0\16\110\1\0\2\110\2\0\1\111\16\110"+
"\2\u0102\23\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\12\110\2\u01e6\27\110\35\0"+
"\2\u01e7\115\0\2\u01e8\61\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\2\53\2\213\37\53\7\0"+
"\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0"+
"\2\233\3\0\2\u0121\41\233\7\0\2\u010e\2\0\1\233"+
"\1\u010f\5\233\1\0\16\233\1\0\2\233\3\0\2\233"+
"\2\u01e9\37\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\2\233\2\u01ea\12\233\1\0\2\233\3\0\43\233"+
"\7\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233"+
"\1\0\2\233\3\0\22\233\2\u01eb\17\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\14\233\2\u01ec\1\0"+
"\2\233\3\0\43\233\13\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\12\53\2\u0140\27\53\13\0"+
"\1\53\1\0\5\53\1\0\16\53\1\0\2\53\3\0"+
"\24\53\2\u01ed\15\53\13\0\1\53\1\0\5\53\1\0"+
"\16\53\1\0\2\53\3\0\35\53\2\213\4\53\13\0"+
"\1\110\1\0\5\110\1\0\2\u01cb\14\110\1\0\2\110"+
"\2\0\1\111\43\110\13\0\1\110\1\0\5\110\1\0"+
"\4\110\2\u01cb\10\110\1\0\2\110\2\0\1\111\43\110"+
"\13\0\1\110\1\0\5\110\1\0\16\110\1\0\2\110"+
"\2\0\1\111\35\110\2\164\4\110\13\0\1\110\1\0"+
"\5\110\1\0\14\110\2\u01cc\1\0\2\110\2\0\1\111"+
"\43\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\24\110\2\u01ee\15\110\13\0\1\110"+
"\1\0\5\110\1\0\14\110\2\u01ef\1\0\2\110\2\0"+
"\1\111\43\110\13\0\1\110\1\0\5\110\1\0\16\110"+
"\1\0\2\110\2\0\1\111\14\110\2\203\25\110\13\0"+
"\1\110\1\0\5\110\1\0\16\110\1\0\2\110\2\0"+
"\1\111\16\110\2\164\23\110\37\0\2\u01f0\112\0\1\u01f1"+
"\57\0\2\u010e\2\0\1\233\1\u010f\5\233\1\0\16\233"+
"\1\0\2\233\3\0\2\233\2\u0110\37\233\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\16\233\1\0\2\233"+
"\3\0\12\233\2\u0189\27\233\7\0\2\u010e\2\0\1\233"+
"\1\u010f\5\233\1\0\16\233\1\0\2\233\3\0\24\233"+
"\2\u01f2\15\233\7\0\2\u010e\2\0\1\233\1\u010f\5\233"+
"\1\0\16\233\1\0\2\233\3\0\35\233\2\u0110\4\233"+
"\13\0\1\53\1\0\5\53\1\0\2\53\2\222\12\53"+
"\1\0\2\53\3\0\43\53\13\0\1\110\1\0\5\110"+
"\1\0\16\110\1\0\2\110\2\0\1\111\12\110\2\u01ad"+
"\27\110\13\0\1\110\1\0\5\110\1\0\16\110\1\0"+
"\2\110\2\0\1\111\14\110\2\377\25\110\7\0\2\u010e"+
"\2\0\1\233\1\u010f\5\233\1\0\2\233\2\u0117\12\233"+
"\1\0\2\233\3\0\43\233\6\0";
private static int [] zzUnpackTrans() {
int [] result = new int[35372];
int offset = 0;
offset = zzUnpackTrans(ZZ_TRANS_PACKED_0, offset, result);
return result;
}
private static int zzUnpackTrans(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
value--;
do result[j++] = value; while (--count > 0);
}
return j;
}
/* error codes */
private static final int ZZ_UNKNOWN_ERROR = 0;
private static final int ZZ_NO_MATCH = 1;
private static final int ZZ_PUSHBACK_2BIG = 2;
/* error messages for the codes above */
private static final String ZZ_ERROR_MSG[] = {
"Unkown internal scanner error",
"Error: could not match input",
"Error: pushback value was too large"
};
/**
* ZZ_ATTRIBUTE[aState] contains the attributes of state aState
*/
private static final int [] ZZ_ATTRIBUTE = zzUnpackAttribute();
private static final String ZZ_ATTRIBUTE_PACKED_0 =
"\5\0\1\1\1\11\6\1\1\11\32\1\1\11\1\0"+
"\1\1\1\0\6\1\1\0\14\1\11\0\1\11\23\0"+
"\1\11\47\0\1\11\4\0\21\1\23\0\25\1\1\11"+
"\2\0\1\11\1\0\1\11\72\0\1\11\2\0\10\1"+
"\1\0\1\11\14\0\1\11\32\0\16\1\1\11\55\0"+
"\5\1\26\0\6\1\41\0\2\1\13\0\3\1\21\0"+
"\1\1\5\0\3\1\16\0\1\1\2\0\2\11\1\0";
private static int [] zzUnpackAttribute() {
int [] result = new int[498];
int offset = 0;
offset = zzUnpackAttribute(ZZ_ATTRIBUTE_PACKED_0, offset, result);
return result;
}
private static int zzUnpackAttribute(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/** the input device */
private java.io.Reader zzReader;
/** the current state of the DFA */
private int zzState;
/** the current lexical state */
private int zzLexicalState = YYINITIAL;
/** this buffer contains the current text to be matched and is
the source of the yytext() string */
private char zzBuffer[] = new char[ZZ_BUFFERSIZE];
/** the textposition at the last accepting state */
private int zzMarkedPos;
/** the current text position in the buffer */
private int zzCurrentPos;
/** startRead marks the beginning of the yytext() string in the buffer */
private int zzStartRead;
/** endRead marks the last character in the buffer, that has been read
from input */
private int zzEndRead;
/** number of newlines encountered up to the start of the matched text */
private int yyline;
/** the number of characters up to the start of the matched text */
private int yychar;
/**
* the number of characters from the last newline up to the start of the
* matched text
*/
private int yycolumn;
/**
* zzAtBOL == true <=> the scanner is currently at the beginning of a line
*/
private boolean zzAtBOL = true;
/** zzAtEOF == true <=> the scanner is at the EOF */
private boolean zzAtEOF;
/** denotes if the user-EOF-code has already been executed */
private boolean zzEOFDone;
/* user code: */
/**
* Create an empty lexer, yyrset will be called later to reset and assign
* the reader
*/
public XHTMLLexer() {
super();
}
@Override
public int yychar() {
return yychar;
}
private static final byte TAG_OPEN = 1;
private static final byte TAG_CLOSE = -1;
private static final byte INSTR_OPEN = 2;
private static final byte INSTR_CLOSE = -2;
private static final byte CDATA_OPEN = 3;
private static final byte CDATA_CLOSE = -3;
private static final byte COMMENT_OPEN = 4;
private static final byte COMMENT_CLOSE = -4;
/**
* Creates a new scanner
* There is also a java.io.InputStream version of this constructor.
*
* @param in the java.io.Reader to read input from.
*/
public XHTMLLexer(java.io.Reader in) {
this.zzReader = in;
}
/**
* Creates a new scanner.
* There is also java.io.Reader version of this constructor.
*
* @param in the java.io.Inputstream to read input from.
*/
public XHTMLLexer(java.io.InputStream in) {
this(new java.io.InputStreamReader(in));
}
/**
* Unpacks the compressed character translation table.
*
* @param packed the packed character translation table
* @return the unpacked character translation table
*/
private static char [] zzUnpackCMap(String packed) {
char [] map = new char[0x10000];
int i = 0; /* index in packed string */
int j = 0; /* index in unpacked array */
while (i < 312) {
int count = packed.charAt(i++);
char value = packed.charAt(i++);
do map[j++] = value; while (--count > 0);
}
return map;
}
/**
* Refills the input buffer.
*
* @return false
, iff there was new input.
*
* @exception java.io.IOException if any I/O-Error occurs
*/
private boolean zzRefill() throws java.io.IOException {
/* first: make room (if you can) */
if (zzStartRead > 0) {
System.arraycopy(zzBuffer, zzStartRead,
zzBuffer, 0,
zzEndRead-zzStartRead);
/* translate stored positions */
zzEndRead-= zzStartRead;
zzCurrentPos-= zzStartRead;
zzMarkedPos-= zzStartRead;
zzStartRead = 0;
}
/* is the buffer big enough? */
if (zzCurrentPos >= zzBuffer.length) {
/* if not: blow it up */
char newBuffer[] = new char[zzCurrentPos*2];
System.arraycopy(zzBuffer, 0, newBuffer, 0, zzBuffer.length);
zzBuffer = newBuffer;
}
/* finally: fill the buffer with new input */
int numRead = zzReader.read(zzBuffer, zzEndRead,
zzBuffer.length-zzEndRead);
if (numRead > 0) {
zzEndRead+= numRead;
return false;
}
// unlikely but not impossible: read 0 characters, but not at end of stream
if (numRead == 0) {
int c = zzReader.read();
if (c == -1) {
return true;
} else {
zzBuffer[zzEndRead++] = (char) c;
return false;
}
}
// numRead < 0
return true;
}
/**
* Closes the input stream.
*/
public final void yyclose() throws java.io.IOException {
zzAtEOF = true; /* indicate end of file */
zzEndRead = zzStartRead; /* invalidate buffer */
if (zzReader != null)
zzReader.close();
}
/**
* Resets the scanner to read from a new input stream.
* Does not close the old reader.
*
* All internal variables are reset, the old input stream
* cannot be reused (internal buffer is discarded and lost).
* Lexical state is set to ZZ_INITIAL.
*
* @param reader the new input stream
*/
public final void yyreset(java.io.Reader reader) {
zzReader = reader;
zzAtBOL = true;
zzAtEOF = false;
zzEOFDone = false;
zzEndRead = zzStartRead = 0;
zzCurrentPos = zzMarkedPos = 0;
yyline = yychar = yycolumn = 0;
zzLexicalState = YYINITIAL;
}
/**
* Returns the current lexical state.
*/
public final int yystate() {
return zzLexicalState;
}
/**
* Enters a new lexical state
*
* @param newState the new lexical state
*/
public final void yybegin(int newState) {
zzLexicalState = newState;
}
/**
* Returns the text matched by the current regular expression.
*/
public final String yytext() {
return new String( zzBuffer, zzStartRead, zzMarkedPos-zzStartRead );
}
/**
* Returns the character at position pos from the
* matched text.
*
* It is equivalent to yytext().charAt(pos), but faster
*
* @param pos the position of the character to fetch.
* A value from 0 to yylength()-1.
*
* @return the character at position pos
*/
public final char yycharat(int pos) {
return zzBuffer[zzStartRead+pos];
}
/**
* Returns the length of the matched text region.
*/
public final int yylength() {
return zzMarkedPos-zzStartRead;
}
/**
* Reports an error that occured while scanning.
*
* In a wellformed scanner (no or only correct usage of
* yypushback(int) and a match-all fallback rule) this method
* will only be called with things that "Can't Possibly Happen".
* If this method is called, something is seriously wrong
* (e.g. a JFlex bug producing a faulty scanner etc.).
*
* Usual syntax/scanner level error handling should be done
* in error fallback rules.
*
* @param errorCode the code of the errormessage to display
*/
private void zzScanError(int errorCode) {
String message;
try {
message = ZZ_ERROR_MSG[errorCode];
}
catch (ArrayIndexOutOfBoundsException e) {
message = ZZ_ERROR_MSG[ZZ_UNKNOWN_ERROR];
}
throw new Error(message);
}
/**
* Pushes the specified amount of characters back into the input stream.
*
* They will be read again by then next call of the scanning method
*
* @param number the number of characters to be read again.
* This number must not be greater than yylength()!
*/
public void yypushback(int number) {
if ( number > yylength() )
zzScanError(ZZ_PUSHBACK_2BIG);
zzMarkedPos -= number;
}
/**
* Resumes scanning until the next regular expression is matched,
* the end of input is encountered or an I/O-Error occurs.
*
* @return the next token
* @exception java.io.IOException if any I/O-Error occurs
*/
public Token yylex() throws java.io.IOException {
int zzInput;
int zzAction;
// cached fields:
int zzCurrentPosL;
int zzMarkedPosL;
int zzEndReadL = zzEndRead;
char [] zzBufferL = zzBuffer;
char [] zzCMapL = ZZ_CMAP;
int [] zzTransL = ZZ_TRANS;
int [] zzRowMapL = ZZ_ROWMAP;
int [] zzAttrL = ZZ_ATTRIBUTE;
while (true) {
zzMarkedPosL = zzMarkedPos;
yychar+= zzMarkedPosL-zzStartRead;
zzAction = -1;
zzCurrentPosL = zzCurrentPos = zzStartRead = zzMarkedPosL;
zzState = ZZ_LEXSTATE[zzLexicalState];
zzForAction: {
while (true) {
if (zzCurrentPosL < zzEndReadL)
zzInput = zzBufferL[zzCurrentPosL++];
else if (zzAtEOF) {
zzInput = YYEOF;
break zzForAction;
}
else {
// store back cached positions
zzCurrentPos = zzCurrentPosL;
zzMarkedPos = zzMarkedPosL;
boolean eof = zzRefill();
// get translated positions and possibly new buffer
zzCurrentPosL = zzCurrentPos;
zzMarkedPosL = zzMarkedPos;
zzBufferL = zzBuffer;
zzEndReadL = zzEndRead;
if (eof) {
zzInput = YYEOF;
break zzForAction;
}
else {
zzInput = zzBufferL[zzCurrentPosL++];
}
}
int zzNext = zzTransL[ zzRowMapL[zzState] + zzCMapL[zzInput] ];
if (zzNext == -1) break zzForAction;
zzState = zzNext;
int zzAttributes = zzAttrL[zzState];
if ( (zzAttributes & 1) == 1 ) {
zzAction = zzState;
zzMarkedPosL = zzCurrentPosL;
if ( (zzAttributes & 8) == 8 ) break zzForAction;
}
}
}
// store back cached position
zzMarkedPos = zzMarkedPosL;
switch (zzAction < 0 ? zzAction : ZZ_ACTION[zzAction]) {
case 14:
{ return token(TokenType.KEYWORD, TAG_CLOSE);
}
case 19: break;
case 11:
{ yybegin(YYINITIAL);
return token(TokenType.COMMENT2, COMMENT_CLOSE);
}
case 20: break;
case 7:
{ yybegin(YYINITIAL);
return token(TokenType.KEYWORD, TAG_CLOSE);
}
case 21: break;
case 4:
{ yybegin(TAG);
return token(TokenType.KEYWORD, TAG_OPEN);
}
case 22: break;
case 8:
{ return token(TokenType.STRING);
}
case 23: break;
case 15:
{ return token(TokenType.KEYWORD2, TAG_CLOSE);
}
case 24: break;
case 2:
{ yybegin(YYINITIAL);
return token(TokenType.KEYWORD);
}
case 25: break;
case 6:
{ return token(TokenType.IDENTIFIER);
}
case 26: break;
case 10:
{ return token(TokenType.KEYWORD2);
}
case 27: break;
case 9:
{ yybegin(INSTR);
return token(TokenType.TYPE2, INSTR_OPEN);
}
case 28: break;
case 13:
{ yybegin(COMMENT);
return token(TokenType.COMMENT2, COMMENT_OPEN);
}
case 29: break;
case 5:
{ yybegin(TAG);
return token(TokenType.KEYWORD2, TAG_OPEN);
}
case 30: break;
case 18:
{ yybegin(CDATA);
return token(TokenType.COMMENT2, CDATA_OPEN);
}
case 31: break;
case 16:
{ yypushback(3);
return token(TokenType.COMMENT);
}
case 32: break;
case 17:
{ yybegin(DOCTYPE);
return token(TokenType.TYPE2, INSTR_OPEN);
}
case 33: break;
case 3:
{ yybegin(YYINITIAL);
return token(TokenType.TYPE2, INSTR_CLOSE);
}
case 34: break;
case 1:
{
}
case 35: break;
case 12:
{ yybegin(YYINITIAL);
return token(TokenType.COMMENT2, CDATA_CLOSE);
}
case 36: break;
default:
if (zzInput == YYEOF && zzStartRead == zzCurrentPos) {
zzAtEOF = true;
switch (zzLexicalState) {
case INSTR: {
return null;
}
case 499: break;
case YYINITIAL: {
return null;
}
case 500: break;
case COMMENT: {
return null;
}
case 501: break;
case CDATA: {
return null;
}
case 502: break;
case TAG: {
return null;
}
case 503: break;
default:
return null;
}
}
else {
zzScanError(ZZ_NO_MATCH);
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy