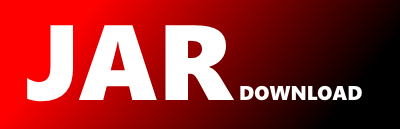
jsyntaxpane.actions.gui.ShowAbbsDialog Maven / Gradle / Ivy
/*
* Copyright 2008 Ayman Al-Sairafi [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License
* at http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jsyntaxpane.actions.gui;
import java.util.Arrays;
import java.util.Map;
import javax.swing.JEditorPane;
import javax.swing.SwingUtilities;
import jsyntaxpane.util.SwingUtils;
/**
* Show abbreviations for a JEditorPane.
*
* @author Ayman Al-Sairafi
*/
public class ShowAbbsDialog
extends javax.swing.JDialog implements EscapeListener {
/**
* Creates new form ShowAbbsDialog
* @param parent
* @param abbs
*/
public ShowAbbsDialog(JEditorPane parent, Map abbs) {
super(SwingUtilities.getWindowAncestor(parent), ModalityType.APPLICATION_MODAL);
initComponents();
Object[] abbsList = abbs.keySet().toArray();
Arrays.sort(abbsList);
jLstAbbs.setListData(abbsList);
this.abbs = abbs;
jEdtAbbr.setEditorKit(parent.getEditorKit());
jLstAbbs.setSelectedIndex(0);
SwingUtils.addEscapeListener(this);
setVisible(true);
}
/**
* This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
jSplitPane1 = new javax.swing.JSplitPane();
jScrollPane1 = new javax.swing.JScrollPane();
jLstAbbs = new javax.swing.JList();
jScrollPane2 = new javax.swing.JScrollPane();
jEdtAbbr = new javax.swing.JEditorPane();
setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE);
java.util.ResourceBundle bundle = java.util.ResourceBundle.getBundle("jsyntaxpane/Bundle"); // NOI18N
setTitle(bundle.getString("ShowAbbsDialog.title")); // NOI18N
setLocationByPlatform(true);
setMinimumSize(new java.awt.Dimension(600, 300));
setModal(true);
setName("dlgShowAbbs"); // NOI18N
jSplitPane1.setDividerLocation(150);
jSplitPane1.setDividerSize(3);
jScrollPane1.setPreferredSize(new java.awt.Dimension(258, 400));
jLstAbbs.setSelectionMode(javax.swing.ListSelectionModel.SINGLE_SELECTION);
jLstAbbs.addListSelectionListener(new javax.swing.event.ListSelectionListener() {
public void valueChanged(javax.swing.event.ListSelectionEvent evt) {
jLstAbbsValueChanged(evt);
}
});
jScrollPane1.setViewportView(jLstAbbs);
jSplitPane1.setLeftComponent(jScrollPane1);
jEdtAbbr.setEditable(false);
jEdtAbbr.setMinimumSize(new java.awt.Dimension(106, 400));
jScrollPane2.setViewportView(jEdtAbbr);
jSplitPane1.setRightComponent(jScrollPane2);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jSplitPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 580, Short.MAX_VALUE)
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jSplitPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 337, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
pack();
}// //GEN-END:initComponents
private void jLstAbbsValueChanged(javax.swing.event.ListSelectionEvent evt) {//GEN-FIRST:event_jLstAbbsValueChanged
if (evt.getValueIsAdjusting() == false) {
Object selected = jLstAbbs.getSelectedValue();
if (selected != null) {
jEdtAbbr.setText(abbs.get(selected.toString()));
}
}
}//GEN-LAST:event_jLstAbbsValueChanged
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JEditorPane jEdtAbbr;
private javax.swing.JList jLstAbbs;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JScrollPane jScrollPane2;
private javax.swing.JSplitPane jSplitPane1;
// End of variables declaration//GEN-END:variables
Map abbs;
@Override
public void escapePressed() {
setVisible(false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy