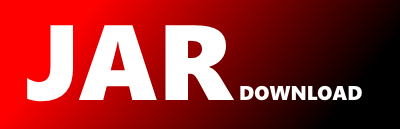
org.keycloak.representations.idm.UserRepresentation Maven / Gradle / Ivy
/*
* Copyright 2016 Red Hat, Inc. and/or its affiliates
* and other contributors as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.keycloak.representations.idm;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* @author Bill Burke
* @version $Revision: 1 $
*/
public class UserRepresentation extends AbstractUserRepresentation{
protected String self; // link
protected String origin;
protected Long createdTimestamp;
protected Boolean enabled;
protected Boolean totp;
protected String federationLink;
protected String serviceAccountClientId; // For rep, it points to clientId (not DB ID)
protected List credentials;
protected Set disableableCredentialTypes;
protected List requiredActions;
protected List federatedIdentities;
protected List realmRoles;
protected Map> clientRoles;
protected List clientConsents;
protected Integer notBefore;
@Deprecated
protected Map> applicationRoles;
@Deprecated
protected List socialLinks;
protected List groups;
private Map access;
public UserRepresentation() {
}
public UserRepresentation(UserRepresentation rep) {
// AbstractUserRepresentation
this.id = rep.getId();
this.username = rep.getUsername();
this.firstName = rep.getFirstName();
this.lastName = rep.getLastName();
this.email = rep.getEmail();
this.emailVerified = rep.isEmailVerified();
this.attributes = rep.getAttributes();
this.setUserProfileMetadata(rep.getUserProfileMetadata());
this.self = rep.getSelf();
this.createdTimestamp = rep.getCreatedTimestamp();
this.enabled = rep.isEnabled();
this.totp = rep.isTotp();
this.federationLink = rep.getFederationLink();
this.serviceAccountClientId = rep.getServiceAccountClientId();
this.credentials = rep.getCredentials();
this.disableableCredentialTypes = rep.getDisableableCredentialTypes();
this.requiredActions = rep.getRequiredActions();
this.federatedIdentities = rep.getFederatedIdentities();
this.realmRoles = rep.getRealmRoles();
this.clientRoles = rep.getClientRoles();
this.clientConsents = rep.getClientConsents();
this.notBefore = rep.getNotBefore();
this.applicationRoles = rep.getApplicationRoles();
this.socialLinks = rep.getSocialLinks();
this.groups = rep.getGroups();
this.access = rep.getAccess();
}
public String getSelf() {
return self;
}
public void setSelf(String self) {
this.self = self;
}
public Long getCreatedTimestamp() {
return createdTimestamp;
}
public void setCreatedTimestamp(Long createdTimestamp) {
this.createdTimestamp = createdTimestamp;
}
public Boolean isEnabled() {
return enabled;
}
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
@Deprecated
public Boolean isTotp() {
return totp;
}
@Deprecated
public void setTotp(Boolean totp) {
this.totp = totp;
}
public List getCredentials() {
return credentials;
}
public void setCredentials(List credentials) {
this.credentials = credentials;
}
public List getRequiredActions() {
return requiredActions;
}
public void setRequiredActions(List requiredActions) {
this.requiredActions = requiredActions;
}
public List getFederatedIdentities() {
return federatedIdentities;
}
public void setFederatedIdentities(List federatedIdentities) {
this.federatedIdentities = federatedIdentities;
}
public List getSocialLinks() {
return socialLinks;
}
public void setSocialLinks(List socialLinks) {
this.socialLinks = socialLinks;
}
public List getRealmRoles() {
return realmRoles;
}
public void setRealmRoles(List realmRoles) {
this.realmRoles = realmRoles;
}
public Map> getClientRoles() {
return clientRoles;
}
public void setClientRoles(Map> clientRoles) {
this.clientRoles = clientRoles;
}
public List getClientConsents() {
return clientConsents;
}
public void setClientConsents(List clientConsents) {
this.clientConsents = clientConsents;
}
public Integer getNotBefore() {
return notBefore;
}
public void setNotBefore(Integer notBefore) {
this.notBefore = notBefore;
}
@Deprecated
public Map> getApplicationRoles() {
return applicationRoles;
}
public String getFederationLink() {
return federationLink;
}
public void setFederationLink(String federationLink) {
this.federationLink = federationLink;
}
public String getServiceAccountClientId() {
return serviceAccountClientId;
}
public void setServiceAccountClientId(String serviceAccountClientId) {
this.serviceAccountClientId = serviceAccountClientId;
}
public List getGroups() {
return groups;
}
public void setGroups(List groups) {
this.groups = groups;
}
/**
* Returns id of UserStorageProvider that loaded this user
*
* @return NULL if user stored locally
* @deprecated Use {@link #getFederationLink()} instead
*/
@Deprecated
public String getOrigin() {
return federationLink;
}
/**
*
* @param origin the origin
* @deprecated Use {@link #setFederationLink(String)} instead
*/
@Deprecated
public void setOrigin(String origin) {
// deprecated
}
public Set getDisableableCredentialTypes() {
return disableableCredentialTypes;
}
public void setDisableableCredentialTypes(Set disableableCredentialTypes) {
this.disableableCredentialTypes = disableableCredentialTypes;
}
public Map getAccess() {
return access;
}
public void setAccess(Map access) {
this.access = access;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy