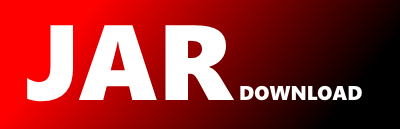
org.keycloak.quarkus.runtime.configuration.DisabledMappersInterceptor Maven / Gradle / Ivy
/*
* Copyright 2024 Red Hat, Inc. and/or its affiliates
* and other contributors as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.keycloak.quarkus.runtime.configuration;
import io.smallrye.config.ConfigSourceInterceptor;
import io.smallrye.config.ConfigSourceInterceptorContext;
import io.smallrye.config.ConfigValue;
import io.smallrye.config.Priorities;
import jakarta.annotation.Priority;
import org.apache.commons.collections4.iterators.FilterIterator;
import org.keycloak.quarkus.runtime.configuration.mappers.PropertyMappers;
import java.util.Iterator;
import static org.keycloak.quarkus.runtime.configuration.MicroProfileConfigProvider.NS_KEYCLOAK_PREFIX;
/**
* This interceptor is responsible for ignoring disabled Keycloak properties
*
*
This interceptor should execute before the {@link PropertyMappingInterceptor} so that disabled properties
* are not mapped to the Quarkus properties.
*
* The reason for the used priority is to always execute the interceptor before default Application Config Source interceptors
* and before the {@link PropertyMappingInterceptor}
*/
@Priority(Priorities.APPLICATION - 20)
public class DisabledMappersInterceptor implements ConfigSourceInterceptor {
private static final ThreadLocal ENABLED = ThreadLocal.withInitial(() -> false);
public static void enable() {
enable(true);
}
public static void disable() {
enable(false);
}
public static void enable(boolean enable) {
ENABLED.set(enable);
}
private boolean isDisabledMapper(String property) {
return property.startsWith(NS_KEYCLOAK_PREFIX) && PropertyMappers.isDisabledMapper(property);
}
Iterator filterDisabledMappers(Iterator iter) {
return new FilterIterator<>(iter, item -> !isDisabledMapper(item));
}
@Override
public Iterator iterateNames(ConfigSourceInterceptorContext context) {
return filterDisabledMappers(context.iterateNames());
}
@Override
public ConfigValue getValue(ConfigSourceInterceptorContext context, String name) {
if (isEnabled() && isDisabledMapper(name)) {
return null;
}
return context.proceed(name);
}
public static boolean isEnabled() {
return Boolean.TRUE.equals(ENABLED.get());
}
public static void runWithDisabled(Runnable execution) {
try {
disable();
execution.run();
} finally {
enable();
}
}
}