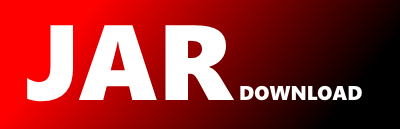
org.keycloak.models.entities.UserEntity Maven / Gradle / Ivy
/*
* Copyright 2016 Red Hat, Inc. and/or its affiliates
* and other contributors as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.keycloak.models.entities;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* @author Marek Posolda
*/
public class UserEntity extends AbstractIdentifiableEntity {
private String username;
private Long createdTimestamp;
private String firstName;
private String lastName;
private String email;
private boolean emailVerified;
private boolean totp;
private boolean enabled;
private String realmId;
private List roleIds;
private List groupIds;
private Map> attributes;
private List requiredActions;
private List credentials = new ArrayList();
private List federatedIdentities;
private String federationLink;
private String serviceAccountClientLink;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public Long getCreatedTimestamp() {
return createdTimestamp;
}
public void setCreatedTimestamp(Long timestamp) {
this.createdTimestamp = timestamp;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public boolean isEmailVerified() {
return emailVerified;
}
public void setEmailVerified(boolean emailVerified) {
this.emailVerified = emailVerified;
}
public boolean isTotp() {
return totp;
}
public void setTotp(boolean totp) {
this.totp = totp;
}
public boolean isEnabled() {
return enabled;
}
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
public String getRealmId() {
return realmId;
}
public void setRealmId(String realmId) {
this.realmId = realmId;
}
public List getRoleIds() {
return roleIds;
}
public void setRoleIds(List roleIds) {
this.roleIds = roleIds;
}
public Map> getAttributes() {
return attributes;
}
public void setAttributes(Map> attributes) {
this.attributes = attributes;
}
public List getRequiredActions() {
return requiredActions;
}
public void setRequiredActions(List requiredActions) {
this.requiredActions = requiredActions;
}
public List getCredentials() {
return credentials;
}
public void setCredentials(List credentials) {
this.credentials = credentials;
}
public List getFederatedIdentities() {
return federatedIdentities;
}
public void setFederatedIdentities(List federatedIdentities) {
this.federatedIdentities = federatedIdentities;
}
public String getFederationLink() {
return federationLink;
}
public void setFederationLink(String federationLink) {
this.federationLink = federationLink;
}
public String getServiceAccountClientLink() {
return serviceAccountClientLink;
}
public void setServiceAccountClientLink(String serviceAccountClientLink) {
this.serviceAccountClientLink = serviceAccountClientLink;
}
public List getGroupIds() {
return groupIds;
}
public void setGroupIds(List groupIds) {
this.groupIds = groupIds;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy