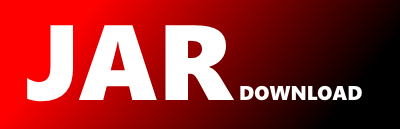
theme.keycloak.v2.account.resources.keycloak-service.keycloak.service.js.map Maven / Gradle / Ivy
{"version":3,"file":"keycloak.service.js","names":["KeycloakService","constructor","keycloak","_defineProperty","keycloakAuth","authenticated","audiencePresent","tokenParsed","audience","Array","isArray","indexOf","login","options","logout","redirectUri","baseUrl","account","accountManagement","authServerUrl","charAt","length","realm","getToken","Promise","resolve","reject","token","updateToken","then","catch"],"sources":["../../src/app/keycloak-service/keycloak.service.ts"],"sourcesContent":["/*\n * Copyright 2017 Red Hat, Inc. and/or its affiliates\n * and other contributors as indicated by the @author tags.\n *\n * Licensed under the Apache License, Version 2.0 (the \"License\");\n * you may not use this file except in compliance with the License.\n * You may obtain a copy of the License at\n *\n * http://www.apache.org/licenses/LICENSE-2.0\n *\n * Unless required by applicable law or agreed to in writing, software\n * distributed under the License is distributed on an \"AS IS\" BASIS,\n * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.\n * See the License for the specific language governing permissions and\n * limitations under the License.\n */\nimport Keycloak, { KeycloakLoginOptions } from \"../../../../../../../../../../js/libs/keycloak-js\";\n\ndeclare const baseUrl: string;\nexport type KeycloakClient = Keycloak;\n\nexport class KeycloakService {\n private keycloakAuth: KeycloakClient;\n\n public constructor(keycloak: KeycloakClient) {\n this.keycloakAuth = keycloak;\n }\n\n public authenticated(): boolean {\n return this.keycloakAuth.authenticated ? this.keycloakAuth.authenticated : false;\n }\n\n public audiencePresent(): boolean {\n if (this.keycloakAuth.tokenParsed) {\n const audience = this.keycloakAuth.tokenParsed['aud'];\n return audience === 'account' || (Array.isArray(audience) && audience.indexOf('account') >= 0);\n }\n return false;\n }\n\n public login(options?: KeycloakLoginOptions): void {\n this.keycloakAuth.login(options);\n }\n\n public logout(redirectUri: string = baseUrl): void {\n this.keycloakAuth.logout({redirectUri: redirectUri});\n }\n\n public account(): void {\n this.keycloakAuth.accountManagement();\n }\n\n public authServerUrl(): string | undefined {\n const authServerUrl = this.keycloakAuth.authServerUrl;\n return authServerUrl!.charAt(authServerUrl!.length - 1) === '/' ? authServerUrl : authServerUrl + '/';\n }\n\n public realm(): string | undefined {\n return this.keycloakAuth.realm;\n }\n\n public getToken(): Promise {\n return new Promise((resolve, reject) => {\n if (this.keycloakAuth.token) {\n this.keycloakAuth\n .updateToken(5)\n .then(() => {\n resolve(this.keycloakAuth.token as string);\n })\n .catch(() => {\n reject('Failed to refresh token');\n });\n } else {\n reject('Not logged in');\n }\n });\n }\n}\n"],"mappings":";;;AAAA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AAMA,OAAO,MAAMA,eAAe,CAAC;EAGlBC,WAAWA,CAACC,QAAwB,EAAE;IAAAC,eAAA;IACzC,IAAI,CAACC,YAAY,GAAGF,QAAQ;EAChC;EAEOG,aAAaA,CAAA,EAAY;IAC5B,OAAO,IAAI,CAACD,YAAY,CAACC,aAAa,GAAG,IAAI,CAACD,YAAY,CAACC,aAAa,GAAG,KAAK;EACpF;EAEOC,eAAeA,CAAA,EAAY;IAC9B,IAAI,IAAI,CAACF,YAAY,CAACG,WAAW,EAAE;MAC/B,MAAMC,QAAQ,GAAG,IAAI,CAACJ,YAAY,CAACG,WAAW,CAAC,KAAK,CAAC;MACrD,OAAOC,QAAQ,KAAK,SAAS,IAAKC,KAAK,CAACC,OAAO,CAACF,QAAQ,CAAC,IAAIA,QAAQ,CAACG,OAAO,CAAC,SAAS,CAAC,IAAI,CAAE;IAClG;IACA,OAAO,KAAK;EAChB;EAEOC,KAAKA,CAACC,OAA8B,EAAQ;IAC/C,IAAI,CAACT,YAAY,CAACQ,KAAK,CAACC,OAAO,CAAC;EACpC;EAEOC,MAAMA,CAACC,WAAmB,GAAGC,OAAO,EAAQ;IAC/C,IAAI,CAACZ,YAAY,CAACU,MAAM,CAAC;MAACC,WAAW,EAAEA;IAAW,CAAC,CAAC;EACxD;EAEOE,OAAOA,CAAA,EAAS;IACnB,IAAI,CAACb,YAAY,CAACc,iBAAiB,CAAC,CAAC;EACzC;EAEOC,aAAaA,CAAA,EAAuB;IACvC,MAAMA,aAAa,GAAG,IAAI,CAACf,YAAY,CAACe,aAAa;IACrD,OAAOA,aAAa,CAAEC,MAAM,CAACD,aAAa,CAAEE,MAAM,GAAG,CAAC,CAAC,KAAK,GAAG,GAAGF,aAAa,GAAGA,aAAa,GAAG,GAAG;EACzG;EAEOG,KAAKA,CAAA,EAAuB;IAC/B,OAAO,IAAI,CAAClB,YAAY,CAACkB,KAAK;EAClC;EAEOC,QAAQA,CAAA,EAAoB;IAC/B,OAAO,IAAIC,OAAO,CAAS,CAACC,OAAO,EAAEC,MAAM,KAAK;MAC5C,IAAI,IAAI,CAACtB,YAAY,CAACuB,KAAK,EAAE;QACzB,IAAI,CAACvB,YAAY,CACZwB,WAAW,CAAC,CAAC,CAAC,CACdC,IAAI,CAAC,MAAM;UACRJ,OAAO,CAAC,IAAI,CAACrB,YAAY,CAACuB,KAAe,CAAC;QAC9C,CAAC,CAAC,CACDG,KAAK,CAAC,MAAM;UACTJ,MAAM,CAAC,yBAAyB,CAAC;QACrC,CAAC,CAAC;MACV,CAAC,MAAM;QACHA,MAAM,CAAC,eAAe,CAAC;MAC3B;IACJ,CAAC,CAAC;EACN;AACJ"}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy