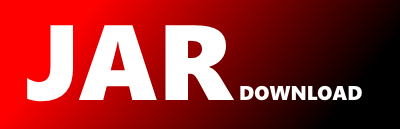
theme.keycloak.v2.account.resources.account-service.account.service.js.map Maven / Gradle / Ivy
{"version":3,"file":"account.service.js","names":["ContentAlert","AccountServiceError","Error","constructor","response","statusText","AccountServiceClient","keycloakService","_defineProperty","kcSvc","accountUrl","authServerUrl","realm","doGet","endpoint","config","doRequest","method","doDelete","doPost","body","JSON","stringify","doPut","fetch","makeUrl","toString","makeConfig","data","json","e","ok","handleError","status","authenticated","audiencePresent","window","location","href","baseUrl","login","err","danger","startsWith","URL","url","hasOwnProperty","params","Object","keys","forEach","key","searchParams","append","Promise","resolve","getToken","then","token","headers","Authorization","catch","addEventListener","event","promise","error","preventDefault"],"sources":["../../src/app/account-service/account.service.ts"],"sourcesContent":["/*\n * Copyright 2018 Red Hat Inc. and/or its affiliates and other contributors\n * as indicated by the @author tags. All rights reserved.\n *\n * Licensed under the Apache License, Version 2.0 (the \"License\"); you may not\n * use this file except in compliance with the License. You may obtain a copy of\n * the License at\n *\n * http://www.apache.org/licenses/LICENSE-2.0\n *\n * Unless required by applicable law or agreed to in writing, software\n * distributed under the License is distributed on an \"AS IS\" BASIS, WITHOUT\n * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the\n * License for the specific language governing permissions and limitations under\n * the License.\n */\n\nimport {KeycloakService} from '../keycloak-service/keycloak.service';\nimport {ContentAlert} from '../content/ContentAlert';\n\ndeclare const baseUrl: string;\n\ntype ConfigResolve = (config: RequestInit) => void;\n\nexport interface HttpResponse extends Response {\n data?: T;\n}\n\nexport interface RequestInitWithParams extends RequestInit {\n params?: {[name: string]: string | number};\n}\n\nexport class AccountServiceError extends Error {\n constructor(public response: HttpResponse) {\n super(response.statusText);\n }\n}\n\n/**\n *\n * @author Stan Silvert [email protected] (C) 2018 Red Hat Inc.\n */\nexport class AccountServiceClient {\n private kcSvc: KeycloakService;\n private accountUrl: string;\n\n public constructor(keycloakService: KeycloakService) {\n this.kcSvc = keycloakService;\n this.accountUrl = this.kcSvc.authServerUrl() + 'realms/' + this.kcSvc.realm() + '/account';\n }\n\n public async doGet(endpoint: string,\n config?: RequestInitWithParams): Promise> {\n return this.doRequest(endpoint, {...config, method: 'get'});\n }\n\n public async doDelete(endpoint: string,\n config?: RequestInitWithParams): Promise> {\n return this.doRequest(endpoint, {...config, method: 'delete'});\n }\n\n public async doPost(endpoint: string,\n body: string | {},\n config?: RequestInitWithParams): Promise> {\n return this.doRequest(endpoint, {...config, body: JSON.stringify(body), method: 'post'});\n }\n\n public async doPut(endpoint: string,\n body: string | {},\n config?: RequestInitWithParams): Promise> {\n return this.doRequest(endpoint, {...config, body: JSON.stringify(body), method: 'put'});\n }\n\n public async doRequest(endpoint: string,\n config?: RequestInitWithParams): Promise> {\n\n const response: HttpResponse = await fetch(this.makeUrl(endpoint, config).toString(),\n await this.makeConfig(config));\n\n try {\n response.data = await response.json();\n } catch (e) {} // ignore. Might be empty\n\n if (!response.ok) {\n this.handleError(response);\n throw new AccountServiceError(response);\n }\n\n return response;\n }\n\n private handleError(response: HttpResponse): void {\n if (response !== null && response.status === 401) {\n if (this.kcSvc.authenticated() && !this.kcSvc.audiencePresent()) {\n // authenticated and the audience is not present => not allowed\n window.location.href = baseUrl + '#/forbidden';\n } else {\n // session timed out?\n this.kcSvc.login();\n }\n }\n\n if (response !== null && response.status === 403) {\n window.location.href = baseUrl + '#/forbidden';\n }\n\n if (response !== null && response.data != null) {\n if (response.data['errors'] != null) {\n for(let err of response.data['errors'])\n ContentAlert.danger(err['errorMessage'], err['params']);\n } else {\n ContentAlert.danger(\n `${response.statusText}: ${response.data['errorMessage'] ? response.data['errorMessage'] : ''} ${response.data['error'] ? response.data['error'] : ''}`);\n };\n } else {\n ContentAlert.danger(response.statusText);\n }\n }\n\n private makeUrl(endpoint: string, config?: RequestInitWithParams): URL {\n if (endpoint.startsWith('http')) return new URL(endpoint);\n const url = new URL(this.accountUrl + endpoint);\n\n // add request params\n if (config && config.hasOwnProperty('params')) {\n const params: {[name: string]: string} = config.params as {} || {};\n Object.keys(params).forEach(key => url.searchParams.append(key, params[key]))\n }\n\n return url;\n }\n\n private makeConfig(config: RequestInit = {}): Promise {\n return new Promise( (resolve: ConfigResolve) => {\n this.kcSvc.getToken()\n .then( (token: string) => {\n resolve( {\n ...config,\n headers: {'Content-Type': 'application/json',\n ...config.headers,\n Authorization: 'Bearer ' + token}\n });\n }).catch(() => {\n this.kcSvc.login();\n });\n });\n }\n\n}\n\nwindow.addEventListener(\"unhandledrejection\", (event: PromiseRejectionEvent) => {\n event.promise.catch(error => {\n if (error instanceof AccountServiceError) {\n // We already handled the error. Ignore unhandled rejection.\n event.preventDefault();\n }\n });\n});\n"],"mappings":";;;AAAA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AAGA,SAAQA,YAAY;AAcpB,OAAO,MAAMC,mBAAmB,SAASC,KAAK,CAAC;EAC3CC,WAAWA,CAAQC,QAAsB,EAAE;IACvC,KAAK,CAACA,QAAQ,CAACC,UAAU,CAAC;IAAC,KADZD,QAAsB,GAAtBA,QAAsB;EAEzC;AACJ;;AAEA;AACA;AACA;AACA;AACA,OAAO,MAAME,oBAAoB,CAAC;EAIvBH,WAAWA,CAACI,eAAgC,EAAE;IAAAC,eAAA;IAAAA,eAAA;IACjD,IAAI,CAACC,KAAK,GAAGF,eAAe;IAC5B,IAAI,CAACG,UAAU,GAAG,IAAI,CAACD,KAAK,CAACE,aAAa,CAAC,CAAC,GAAG,SAAS,GAAG,IAAI,CAACF,KAAK,CAACG,KAAK,CAAC,CAAC,GAAG,UAAU;EAC9F;EAEA,MAAaC,KAAKA,CAAIC,QAAgB,EAChBC,MAA8B,EAA4B;IAC5E,OAAO,IAAI,CAACC,SAAS,CAACF,QAAQ,EAAE;MAAC,GAAGC,MAAM;MAAEE,MAAM,EAAE;IAAK,CAAC,CAAC;EAC/D;EAEA,MAAaC,QAAQA,CAAIJ,QAAgB,EACjBC,MAA8B,EAA4B;IAC9E,OAAO,IAAI,CAACC,SAAS,CAACF,QAAQ,EAAE;MAAC,GAAGC,MAAM;MAAEE,MAAM,EAAE;IAAQ,CAAC,CAAC;EAClE;EAEA,MAAaE,MAAMA,CAAIL,QAAgB,EACjBM,IAAiB,EACjBL,MAA8B,EAA4B;IAC5E,OAAO,IAAI,CAACC,SAAS,CAACF,QAAQ,EAAE;MAAC,GAAGC,MAAM;MAAEK,IAAI,EAAEC,IAAI,CAACC,SAAS,CAACF,IAAI,CAAC;MAAEH,MAAM,EAAE;IAAM,CAAC,CAAC;EAC5F;EAEA,MAAaM,KAAKA,CAAIT,QAAgB,EACjBM,IAAiB,EACjBL,MAA8B,EAA4B;IAC3E,OAAO,IAAI,CAACC,SAAS,CAACF,QAAQ,EAAE;MAAC,GAAGC,MAAM;MAAEK,IAAI,EAAEC,IAAI,CAACC,SAAS,CAACF,IAAI,CAAC;MAAEH,MAAM,EAAE;IAAK,CAAC,CAAC;EAC3F;EAEA,MAAaD,SAASA,CAAIF,QAAgB,EAChBC,MAA8B,EAA4B;IAEhF,MAAMX,QAAyB,GAAG,MAAMoB,KAAK,CAAC,IAAI,CAACC,OAAO,CAACX,QAAQ,EAAEC,MAAM,CAAC,CAACW,QAAQ,CAAC,CAAC,EACzC,MAAM,IAAI,CAACC,UAAU,CAACZ,MAAM,CAAC,CAAC;IAE5E,IAAI;MACAX,QAAQ,CAACwB,IAAI,GAAG,MAAMxB,QAAQ,CAACyB,IAAI,CAAC,CAAC;IACzC,CAAC,CAAC,OAAOC,CAAC,EAAE,CAAC,CAAC,CAAC;;IAEf,IAAI,CAAC1B,QAAQ,CAAC2B,EAAE,EAAE;MACd,IAAI,CAACC,WAAW,CAAC5B,QAAQ,CAAC;MAC1B,MAAM,IAAIH,mBAAmB,CAACG,QAAQ,CAAC;IAC3C;IAEA,OAAOA,QAAQ;EACnB;EAEQ4B,WAAWA,CAAC5B,QAAsB,EAAQ;IAC9C,IAAIA,QAAQ,KAAK,IAAI,IAAIA,QAAQ,CAAC6B,MAAM,KAAK,GAAG,EAAE;MAC9C,IAAI,IAAI,CAACxB,KAAK,CAACyB,aAAa,CAAC,CAAC,IAAI,CAAC,IAAI,CAACzB,KAAK,CAAC0B,eAAe,CAAC,CAAC,EAAE;QAC7D;QACAC,MAAM,CAACC,QAAQ,CAACC,IAAI,GAAGC,OAAO,GAAG,aAAa;MAClD,CAAC,MAAM;QACH;QACA,IAAI,CAAC9B,KAAK,CAAC+B,KAAK,CAAC,CAAC;MACtB;IACJ;IAEA,IAAIpC,QAAQ,KAAK,IAAI,IAAIA,QAAQ,CAAC6B,MAAM,KAAK,GAAG,EAAE;MAC9CG,MAAM,CAACC,QAAQ,CAACC,IAAI,GAAGC,OAAO,GAAG,aAAa;IAClD;IAEA,IAAInC,QAAQ,KAAK,IAAI,IAAIA,QAAQ,CAACwB,IAAI,IAAI,IAAI,EAAE;MAC5C,IAAIxB,QAAQ,CAACwB,IAAI,CAAC,QAAQ,CAAC,IAAI,IAAI,EAAE;QACjC,KAAI,IAAIa,GAAG,IAAIrC,QAAQ,CAACwB,IAAI,CAAC,QAAQ,CAAC,EAClC5B,YAAY,CAAC0C,MAAM,CAACD,GAAG,CAAC,cAAc,CAAC,EAAEA,GAAG,CAAC,QAAQ,CAAC,CAAC;MAC/D,CAAC,MAAM;QACHzC,YAAY,CAAC0C,MAAM,CAClB,GAAEtC,QAAQ,CAACC,UAAW,KAAID,QAAQ,CAACwB,IAAI,CAAC,cAAc,CAAC,GAAGxB,QAAQ,CAACwB,IAAI,CAAC,cAAc,CAAC,GAAG,EAAG,IAAGxB,QAAQ,CAACwB,IAAI,CAAC,OAAO,CAAC,GAAGxB,QAAQ,CAACwB,IAAI,CAAC,OAAO,CAAC,GAAG,EAAG,EAAC,CAAC;MAC5J;MAAC;IACL,CAAC,MAAM;MACH5B,YAAY,CAAC0C,MAAM,CAACtC,QAAQ,CAACC,UAAU,CAAC;IAC5C;EACJ;EAEQoB,OAAOA,CAACX,QAAgB,EAAEC,MAA8B,EAAO;IACnE,IAAID,QAAQ,CAAC6B,UAAU,CAAC,MAAM,CAAC,EAAE,OAAO,IAAIC,GAAG,CAAC9B,QAAQ,CAAC;IACzD,MAAM+B,GAAG,GAAG,IAAID,GAAG,CAAC,IAAI,CAAClC,UAAU,GAAGI,QAAQ,CAAC;;IAE/C;IACA,IAAIC,MAAM,IAAIA,MAAM,CAAC+B,cAAc,CAAC,QAAQ,CAAC,EAAE;MAC3C,MAAMC,MAAgC,GAAGhC,MAAM,CAACgC,MAAM,IAAU,CAAC,CAAC;MAClEC,MAAM,CAACC,IAAI,CAACF,MAAM,CAAC,CAACG,OAAO,CAACC,GAAG,IAAIN,GAAG,CAACO,YAAY,CAACC,MAAM,CAACF,GAAG,EAAEJ,MAAM,CAACI,GAAG,CAAC,CAAC,CAAC;IACjF;IAEA,OAAON,GAAG;EACd;EAEQlB,UAAUA,CAACZ,MAAmB,GAAG,CAAC,CAAC,EAAwB;IAC/D,OAAO,IAAIuC,OAAO,CAAGC,OAAsB,IAAK;MAC5C,IAAI,CAAC9C,KAAK,CAAC+C,QAAQ,CAAC,CAAC,CAChBC,IAAI,CAAGC,KAAa,IAAK;QACtBH,OAAO,CAAE;UACL,GAAGxC,MAAM;UACT4C,OAAO,EAAE;YAAC,cAAc,EAAE,kBAAkB;YACnC,GAAG5C,MAAM,CAAC4C,OAAO;YAChBC,aAAa,EAAE,SAAS,GAAGF;UAAK;QAC9C,CAAC,CAAC;MACN,CAAC,CAAC,CAACG,KAAK,CAAC,MAAM;QACX,IAAI,CAACpD,KAAK,CAAC+B,KAAK,CAAC,CAAC;MACtB,CAAC,CAAC;IACV,CAAC,CAAC;EACN;AAEJ;AAEAJ,MAAM,CAAC0B,gBAAgB,CAAC,oBAAoB,EAAGC,KAA4B,IAAK;EAC5EA,KAAK,CAACC,OAAO,CAACH,KAAK,CAACI,KAAK,IAAI;IACzB,IAAIA,KAAK,YAAYhE,mBAAmB,EAAE;MACtC;MACA8D,KAAK,CAACG,cAAc,CAAC,CAAC;IAC1B;EACJ,CAAC,CAAC;AACN,CAAC,CAAC"}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy