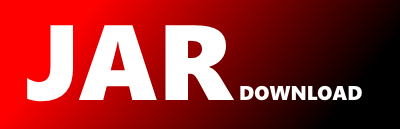
theme.keycloak.v2.account.resources.widgets.ContinueCancelModal.js.map Maven / Gradle / Ivy
{"version":3,"file":"ContinueCancelModal.js","names":["React","Modal","ModalVariant","Button","Msg","ContinueCancelModal","Component","constructor","props","_defineProperty","setState","isModalOpen","onClose","handleModalToggle","onContinue","state","render","createElement","Fragment","id","buttonId","variant","buttonVariant","onClick","isDisabled","msgKey","buttonTitle","_extends","small","title","localize","modalTitle","isOpen","actions","key","handleContinue","modalContinueButtonLabel","modalCancelButtonLabel","modalMessage","children"],"sources":["../../src/app/widgets/ContinueCancelModal.tsx"],"sourcesContent":["/*\n * Copyright 2019 Red Hat, Inc. and/or its affiliates.\n *\n * Licensed under the Apache License, Version 2.0 (the \"License\");\n * you may not use this file except in compliance with the License.\n * You may obtain a copy of the License at\n *\n * http://www.apache.org/licenses/LICENSE-2.0\n *\n * Unless required by applicable law or agreed to in writing, software\n * distributed under the License is distributed on an \"AS IS\" BASIS,\n * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.\n * See the License for the specific language governing permissions and\n * limitations under the License.\n */\n\nimport * as React from 'react';\nimport { Modal, ModalVariant, Button, ButtonProps } from '@patternfly/react-core';\nimport {Msg} from './Msg';\n\n/**\n * For any of these properties that are strings, you can\n * pass in a localization key instead of a static string.\n */\ninterface ContinueCancelModalProps {\n buttonTitle?: string;\n buttonVariant?: ButtonProps['variant'];\n buttonId?: string;\n render?(toggle: () => void): React.ReactNode;\n modalTitle: string;\n modalMessage?: string;\n modalContinueButtonLabel?: string;\n modalCancelButtonLabel?: string;\n onContinue: () => void;\n onClose?: () => void;\n isDisabled?: boolean;\n}\n\ninterface ContinueCancelModalState {\n isModalOpen: boolean;\n}\n\n/**\n * This class renders a button that provides a continue/cancel modal dialog when clicked. If the user selects 'Continue'\n * then the onContinue function is executed.\n *\n * @author Stan Silvert [email protected] (C) 2019 Red Hat Inc.\n */\nexport class ContinueCancelModal extends React.Component {\n protected static defaultProps = {\n buttonVariant: 'primary',\n modalContinueButtonLabel: 'continue',\n modalCancelButtonLabel: 'doCancel',\n isDisabled: false\n };\n\n public constructor(props: ContinueCancelModalProps) {\n super(props);\n this.state = {\n isModalOpen: false\n };\n }\n\n private handleModalToggle = () => {\n this.setState(({ isModalOpen }) => ({\n isModalOpen: !isModalOpen\n }));\n if (this.props.onClose) this.props.onClose();\n };\n\n private handleContinue = () => {\n this.handleModalToggle();\n this.props.onContinue();\n }\n\n public render(): React.ReactNode {\n const { isModalOpen } = this.state;\n\n return (\n \n {!this.props.render &&\n }\n {this.props.render && this.props.render(this.handleModalToggle)}\n \n \n ,\n \n ]}\n >\n { !this.props.modalMessage && this.props.children}\n { this.props.modalMessage && }\n \n \n );\n }\n};\n"],"mappings":";;;;AAAA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AAEA,OAAO,KAAKA,KAAK;AACjB,SAASC,KAAK,EAAEC,YAAY,EAAEC,MAAM;AACpC,SAAQC,GAAG;;AAEX;AACA;AACA;AACA;;AAmBA;AACA;AACA;AACA;AACA;AACA;AACA,OAAO,MAAMC,mBAAmB,SAASL,KAAK,CAACM,SAAS,CAAqD;EAQlGC,WAAWA,CAACC,KAA+B,EAAE;IAChD,KAAK,CAACA,KAAK,CAAC;IAACC,eAAA,4BAMW,MAAM;MAC9B,IAAI,CAACC,QAAQ,CAAC,CAAC;QAAEC;MAAY,CAAC,MAAM;QAChCA,WAAW,EAAE,CAACA;MAClB,CAAC,CAAC,CAAC;MACH,IAAI,IAAI,CAACH,KAAK,CAACI,OAAO,EAAE,IAAI,CAACJ,KAAK,CAACI,OAAO,CAAC,CAAC;IAChD,CAAC;IAAAH,eAAA,yBAEwB,MAAM;MAC3B,IAAI,CAACI,iBAAiB,CAAC,CAAC;MACxB,IAAI,CAACL,KAAK,CAACM,UAAU,CAAC,CAAC;IAC3B,CAAC;IAfG,IAAI,CAACC,KAAK,GAAG;MACTJ,WAAW,EAAE;IACjB,CAAC;EACL;EAcOK,MAAMA,CAAA,EAAoB;IAC7B,MAAM;MAAEL;IAAY,CAAC,GAAG,IAAI,CAACI,KAAK;IAElC,oBACIf,KAAA,CAAAiB,aAAA,CAACjB,KAAK,CAACkB,QAAQ,QACV,CAAC,IAAI,CAACV,KAAK,CAACQ,MAAM,iBACnBhB,KAAA,CAAAiB,aAAA,CAACd,MAAM;MAACgB,EAAE,EAAE,IAAI,CAACX,KAAK,CAACY,QAAS;MAACC,OAAO,EAAE,IAAI,CAACb,KAAK,CAACc,aAAc;MAACC,OAAO,EAAE,IAAI,CAACV,iBAAkB;MAACW,UAAU,EAAE,IAAI,CAAChB,KAAK,CAACgB;IAAW,gBACnIxB,KAAA,CAAAiB,aAAA,CAACb,GAAG;MAACqB,MAAM,EAAE,IAAI,CAACjB,KAAK,CAACkB;IAAa,CAAC,CAClC,CAAC,EACR,IAAI,CAAClB,KAAK,CAACQ,MAAM,IAAI,IAAI,CAACR,KAAK,CAACQ,MAAM,CAAC,IAAI,CAACH,iBAAiB,CAAC,eAC/Db,KAAA,CAAAiB,aAAA,CAAChB,KAAK,EAAA0B,QAAA,KACE,IAAI,CAACnB,KAAK;MACda,OAAO,EAAEnB,YAAY,CAAC0B,KAAM;MAC5BC,KAAK,EAAEzB,GAAG,CAAC0B,QAAQ,CAAC,IAAI,CAACtB,KAAK,CAACuB,UAAU,CAAE;MAC3CC,MAAM,EAAErB,WAAY;MACpBC,OAAO,EAAE,IAAI,CAACC,iBAAkB;MAChCoB,OAAO,EAAE,cACLjC,KAAA,CAAAiB,aAAA,CAACd,MAAM;QAACgB,EAAE,EAAC,eAAe;QAACe,GAAG,EAAC,SAAS;QAACb,OAAO,EAAC,SAAS;QAACE,OAAO,EAAE,IAAI,CAACY;MAAe,gBACpFnC,KAAA,CAAAiB,aAAA,CAACb,GAAG;QAACqB,MAAM,EAAE,IAAI,CAACjB,KAAK,CAAC4B;MAA0B,CAAC,CAC/C,CAAC,eACTpC,KAAA,CAAAiB,aAAA,CAACd,MAAM;QAACgB,EAAE,EAAC,cAAc;QAACe,GAAG,EAAC,QAAQ;QAACb,OAAO,EAAC,WAAW;QAACE,OAAO,EAAE,IAAI,CAACV;MAAkB,gBACvFb,KAAA,CAAAiB,aAAA,CAACb,GAAG;QAACqB,MAAM,EAAE,IAAI,CAACjB,KAAK,CAAC6B;MAAwB,CAAC,CAC7C,CAAC;IACX,IAEA,CAAC,IAAI,CAAC7B,KAAK,CAAC8B,YAAY,IAAI,IAAI,CAAC9B,KAAK,CAAC+B,QAAQ,EAC/C,IAAI,CAAC/B,KAAK,CAAC8B,YAAY,iBAAItC,KAAA,CAAAiB,aAAA,CAACb,GAAG;MAACqB,MAAM,EAAE,IAAI,CAACjB,KAAK,CAAC8B;IAAa,CAAC,CAChE,CACK,CAAC;EAEzB;AACJ;AAAC7B,eAAA,CA1DYJ,mBAAmB,kBACI;EAC5BiB,aAAa,EAAE,SAAS;EACxBc,wBAAwB,EAAE,UAAU;EACpCC,sBAAsB,EAAE,UAAU;EAClCb,UAAU,EAAE;AAChB,CAAC;AAoDJ"}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy