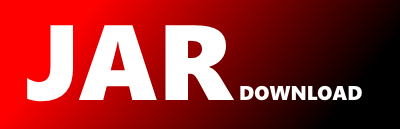
org.kie.api.executor.ExecutorQueryService Maven / Gradle / Ivy
/*
* Copyright 2013 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kie.api.executor;
import java.util.List;
import org.kie.api.runtime.query.QueryContext;
/**
* Executor query interface that provides runtime access to data.
*/
public interface ExecutorQueryService {
/**
* @return list of pending execution requests.
*/
List getPendingRequests(QueryContext queryContext);
/**
* @param id unique id of the request
* @return given pending request identified by id
*/
List getPendingRequestById(Long id);
/**
* @param id unique id of the request
* @return request identified by id
regardless of its status
*/
RequestInfo getRequestById(Long id);
/**
* Returns requests identified by businessKey
usually it should be only one with given
* business key but it does not have to as same business key requests can be processed sequentially and
* thus might be in different statuses.
*
* @param businessKey business key of the request
* @return requests identified by the business key
*/
List getRequestByBusinessKey(String businessKey, QueryContext queryContext);
/**
* Returns requests identified by businessKey
usually it should be only one with given
* business key but it does not have to as same business key requests can be processed sequentially and
* thus might be in different statuses.
*
* @param businessKey business key of the request
* @param statuses filter by job status
* @param queryContext paging and sorting controls
* @return requests identified by the business key
*/
List getRequestsByBusinessKey(String businessKey, List statuses, QueryContext queryContext);
/**
* Returns requests that are scheduled to run given command
* @param command command configured in the request
* @param queryContext paging and sorting controls
* @return requests configured with given command
*/
List getRequestByCommand(String command, QueryContext queryContext);
/**
* Returns requests that are scheduled to run given command
* @param command command configured in the request
* @param statuses filter by job status
* @param queryContext paging and sorting controls
* @return requests configured with given command
*/
List getRequestsByCommand(String command, List statuses, QueryContext queryContext);
/**
* Returns requests by deployment id
* @param deploymentId deployment id from process execution context
* @param statuses filter by job status
* @param queryContext paging and sorting controls
* @return requests scheduled for given deployment
*/
List getRequestsByDeployment(String deploymentId, List statuses, QueryContext queryContext);
/**
* Returns requests by process instance id
* @param processInstanceId process instance id from process execution context
* @param statuses filter by job status
* @param queryContext paging and sorting controls
* @return requests scheduled for given deployment
*/
List getRequestsByProcessInstance(Long processInstanceId, List statuses, QueryContext queryContext);
/**
* @param id unique id of the request
* @return all errors (if any) for given request
*/
List getErrorsByRequestId(Long id);
/**
* @return all queued requests
*/
List getQueuedRequests(QueryContext queryContext);
/**
* @return all comleted requests.
*/
List getCompletedRequests(QueryContext queryContext);
/**
* @return all requests that have errors.
*/
List getInErrorRequests(QueryContext queryContext);
/**
* @return all requests that were cancelled
*/
List getCancelledRequests(QueryContext queryContext);
/**
* @return all errors.
*/
List getAllErrors(QueryContext queryContext);
/**
* @return all requests
*/
List getAllRequests(QueryContext queryContext);
/**
* @return all currently running requests
*/
List getRunningRequests(QueryContext queryContext);
/**
* @return requests queued for future execution
*/
List getFutureQueuedRequests(QueryContext queryContext);
/**
* @param statuses statuses that requests should be in
* @return requests based on their status
*/
List getRequestsByStatus(List statuses, QueryContext queryContext);
/**
* Dedicated method for handling special case that is get the request for processing.
* To ensure its efficient use it shall perform necessary operation to minimize risk of
* race conditions and deadlock.
*/
RequestInfo getRequestForProcessing();
/**
* Dedicated method for handling special case that is get the request for processing by id.
* To ensure its efficient use it shall perform necessary operation to minimize risk of
* race conditions and deadlock.
*/
RequestInfo getRequestForProcessing(Long requestId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy