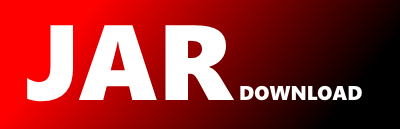
org.kie.kogito.rules.RuleUnitConfig Maven / Gradle / Ivy
package org.kie.kogito.rules;
import java.util.Optional;
import java.util.OptionalInt;
import org.kie.kogito.conf.ClockType;
import org.kie.kogito.conf.EventProcessingType;
public final class RuleUnitConfig {
public static final RuleUnitConfig Default =
new RuleUnitConfig(EventProcessingType.CLOUD, ClockType.REALTIME, null);
private final Optional eventProcessingType;
private final Optional clockType;
private final OptionalInt sessionPool;
public RuleUnitConfig(EventProcessingType eventProcessingType, ClockType clockType, Integer sessionPool) {
this.eventProcessingType = Optional.ofNullable(eventProcessingType);
this.clockType = Optional.ofNullable(clockType);
this.sessionPool = sessionPool == null? OptionalInt.empty() : OptionalInt.of(sessionPool);
}
public RuleUnitConfig(Optional eventProcessingType, Optional clockType, OptionalInt sessionPool) {
this.eventProcessingType = eventProcessingType;
this.clockType = clockType;
this.sessionPool = sessionPool;
}
public Optional getEventProcessingType() {
return eventProcessingType;
}
public EventProcessingType getDefaultedEventProcessingType() {
return getEventProcessingType().orElseGet(Default.getEventProcessingType()::get);
}
public Optional getClockType() {
return clockType;
}
public ClockType getDefaultedClockType() {
return getClockType().orElseGet(Default.getClockType()::get);
}
public OptionalInt getSessionPool() {
return sessionPool;
}
public OptionalInt getDefaultedSessionPool() {
return sessionPool.isPresent() ? sessionPool : Default.getSessionPool();
}
/**
* return the merged config with the given. Given config keys win over this
*/
public RuleUnitConfig merged(RuleUnitConfig overrides) {
if (overrides == null) {
return this;
}
return new RuleUnitConfig(
overrides.getEventProcessingType().isPresent() ? overrides.getEventProcessingType() : this.getEventProcessingType(),
overrides.getClockType().isPresent() ? overrides.getClockType() : this.getClockType(),
overrides.getSessionPool().isPresent() ? overrides.getSessionPool() : this.getSessionPool());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy