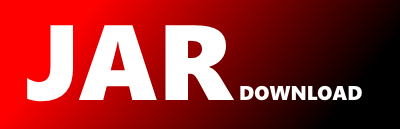
org.kie.server.client.KieServicesClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-server-client Show documentation
Show all versions of kie-server-client Show documentation
KIE Execution Server Client
/*
* Copyright 2015 - 2017 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kie.server.client;
import javax.ws.rs.core.Response.Status;
import org.kie.api.command.Command;
import org.kie.server.api.commands.CommandScript;
import org.kie.server.api.model.KieContainerResource;
import org.kie.server.api.model.KieContainerResourceFilter;
import org.kie.server.api.model.KieContainerResourceList;
import org.kie.server.api.model.KieScannerResource;
import org.kie.server.api.model.KieServerInfo;
import org.kie.server.api.model.KieServerStateInfo;
import org.kie.server.api.model.ReleaseId;
import org.kie.server.api.model.ServiceResponse;
import org.kie.server.api.model.ServiceResponsesList;
import org.kie.server.client.jms.ResponseHandler;
public interface KieServicesClient {
T getServicesClient(Class serviceClient);
ServiceResponse getServerInfo();
ServiceResponse listContainers();
ServiceResponse listContainers(KieContainerResourceFilter containerFilter);
ServiceResponse createContainer(String id, KieContainerResource resource);
ServiceResponse activateContainer(String id);
ServiceResponse deactivateContainer(String id);
ServiceResponse getContainerInfo(String id);
ServiceResponse disposeContainer(String id);
ServiceResponsesList executeScript(CommandScript script);
ServiceResponse getScannerInfo(String id);
ServiceResponse updateScanner(String id, KieScannerResource resource);
ServiceResponse getReleaseId(String containerId);
ServiceResponse updateReleaseId(String id, ReleaseId releaseId);
ServiceResponse updateReleaseId(String id, ReleaseId releaseId, boolean resetBeforeUpdate);
ServiceResponse getServerState();
void close();
// for backward compatibility reason
/**
* This method is deprecated on KieServicesClient as it was moved to RuleServicesClient
* @see RuleServicesClient#executeCommands(String, String)
* @deprecated
*/
@Deprecated
ServiceResponse executeCommands(String id, String payload);
/**
* This method is deprecated on KieServicesClient as it was moved to RuleServicesClient
* @see RuleServicesClient#executeCommands(String, Command)
* @deprecated
*/
@Deprecated
default ServiceResponse executeCommands(String id, Command> cmd) {
return executeCommands(id, cmd, Status.OK);
}
/**
* This method is deprecated on KieServicesClient as it was moved to RuleServicesClient
* @see RuleServicesClient#executeCommands(String, Command)
* @deprecated
*/
@Deprecated
default ServiceResponse executeCommands(String id, Command> cmd, Status response) {
throw new UnsupportedOperationException();
}
/**
* Sets the classloader for user class unmarshalling
* @param classLoader
*/
void setClassLoader(ClassLoader classLoader);
/**
* Returns the current classloader in use for unmarshalling
* @return
*/
ClassLoader getClassLoader();
String getConversationId();
void completeConversation();
void setResponseHandler(ResponseHandler responseHandler);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy