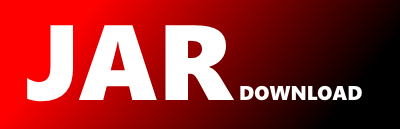
org.kie.server.services.jbpm.JBPMKieContainerCommandServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-server-services-jbpm Show documentation
Show all versions of kie-server-services-jbpm Show documentation
KIE jBPM Execution Server Extension
/*
* Copyright 2015 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kie.server.services.jbpm;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.lang3.reflect.MethodUtils;
import org.jbpm.services.api.DeploymentService;
import org.kie.server.api.commands.CommandScript;
import org.kie.server.api.commands.DescriptorCommand;
import org.kie.server.api.marshalling.MarshallingFormat;
import org.kie.server.api.marshalling.ModelWrapper;
import org.kie.server.api.model.KieServerCommand;
import org.kie.server.api.model.ServiceResponse;
import org.kie.server.api.model.ServiceResponsesList;
import org.kie.server.api.model.Wrapped;
import org.kie.server.services.api.KieContainerCommandService;
import org.kie.server.services.api.KieServerRegistry;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class JBPMKieContainerCommandServiceImpl implements KieContainerCommandService {
private static final Logger logger = LoggerFactory.getLogger(JBPMKieContainerCommandServiceImpl.class);
private KieServerRegistry context;
private DeploymentService deploymentService;
private DefinitionServiceBase definitionServiceBase;
private ProcessServiceBase processServiceBase;
private UserTaskServiceBase userTaskServiceBase;
private RuntimeDataServiceBase runtimeDataServiceBase;
private ExecutorServiceBase executorServiceBase;
private QueryDataServiceBase queryDataServiceBase;
public JBPMKieContainerCommandServiceImpl(KieServerRegistry context, DeploymentService deploymentService,
DefinitionServiceBase definitionServiceBase, ProcessServiceBase processServiceBase, UserTaskServiceBase userTaskServiceBase,
RuntimeDataServiceBase runtimeDataServiceBase, ExecutorServiceBase executorServiceBase, QueryDataServiceBase queryDataServiceBase) {
this.context = context;
this.deploymentService = deploymentService;
this.definitionServiceBase = definitionServiceBase;
this.processServiceBase = processServiceBase;
this.userTaskServiceBase = userTaskServiceBase;
this.runtimeDataServiceBase = runtimeDataServiceBase;
this.executorServiceBase = executorServiceBase;
this.queryDataServiceBase = queryDataServiceBase;
}
@Override
public ServiceResponse callContainer(String containerId, String payload, MarshallingFormat marshallingFormat, String classType) {
return null;
}
@Override
public ServiceResponsesList executeScript(CommandScript commands, MarshallingFormat marshallingFormat, String classType) {
List> responses = new ArrayList>();
for (KieServerCommand command : commands.getCommands()) {
if (!(command instanceof DescriptorCommand)) {
logger.warn("Unsupported command '{}' given, will not process it", command.getClass().getName());
continue;
}
boolean wrapResults = false;
try {
Object result = null;
Object handler = null;
DescriptorCommand descriptorCommand = (DescriptorCommand) command;
// find out the handler to call to process given command
if ("DefinitionService".equals(descriptorCommand.getService())) {
handler = definitionServiceBase;
} else if ("ProcessService".equals(descriptorCommand.getService())) {
handler = processServiceBase;
} else if ("UserTaskService".equals(descriptorCommand.getService())) {
handler = userTaskServiceBase;
} else if ("QueryService".equals(descriptorCommand.getService())) {
handler = runtimeDataServiceBase;
} else if ("JobService".equals(descriptorCommand.getService())) {
handler = executorServiceBase;
} else if ("QueryDataService".equals(descriptorCommand.getService())) {
handler = queryDataServiceBase;
// enable wrapping as in case of embedded objects jaxb does not properly parse it due to possible unknown types (List> etc)
if (marshallingFormat.equals(MarshallingFormat.JAXB)) {
wrapResults = true;
}
} else {
throw new IllegalStateException("Unable to find handler for " + descriptorCommand.getService() + " service");
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy