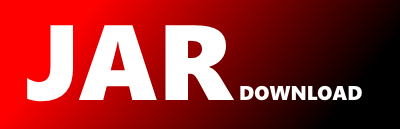
bpsim.BpsimFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package bpsim;
import org.eclipse.emf.ecore.EFactory;
/**
*
* The Factory for the model.
* It provides a create method for each non-abstract class of the model.
*
* @see bpsim.BpsimPackage
* @generated
*/
public interface BpsimFactory extends EFactory {
/**
* The singleton instance of the factory.
*
*
* @generated
*/
BpsimFactory eINSTANCE = bpsim.impl.BpsimFactoryImpl.init();
/**
* Returns a new object of class 'Beta Distribution Type'.
*
*
* @return a new object of class 'Beta Distribution Type'.
* @generated
*/
BetaDistributionType createBetaDistributionType();
/**
* Returns a new object of class 'Binomial Distribution Type'.
*
*
* @return a new object of class 'Binomial Distribution Type'.
* @generated
*/
BinomialDistributionType createBinomialDistributionType();
/**
* Returns a new object of class 'Boolean Parameter Type'.
*
*
* @return a new object of class 'Boolean Parameter Type'.
* @generated
*/
BooleanParameterType createBooleanParameterType();
/**
* Returns a new object of class 'BP Sim Data Type'.
*
*
* @return a new object of class 'BP Sim Data Type'.
* @generated
*/
BPSimDataType createBPSimDataType();
/**
* Returns a new object of class 'Calendar'.
*
*
* @return a new object of class 'Calendar'.
* @generated
*/
Calendar createCalendar();
/**
* Returns a new object of class 'Constant Parameter'.
*
*
* @return a new object of class 'Constant Parameter'.
* @generated
*/
ConstantParameter createConstantParameter();
/**
* Returns a new object of class 'Control Parameters'.
*
*
* @return a new object of class 'Control Parameters'.
* @generated
*/
ControlParameters createControlParameters();
/**
* Returns a new object of class 'Cost Parameters'.
*
*
* @return a new object of class 'Cost Parameters'.
* @generated
*/
CostParameters createCostParameters();
/**
* Returns a new object of class 'Date Time Parameter Type'.
*
*
* @return a new object of class 'Date Time Parameter Type'.
* @generated
*/
DateTimeParameterType createDateTimeParameterType();
/**
* Returns a new object of class 'Distribution Parameter'.
*
*
* @return a new object of class 'Distribution Parameter'.
* @generated
*/
DistributionParameter createDistributionParameter();
/**
* Returns a new object of class 'Document Root'.
*
*
* @return a new object of class 'Document Root'.
* @generated
*/
DocumentRoot createDocumentRoot();
/**
* Returns a new object of class 'Duration Parameter Type'.
*
*
* @return a new object of class 'Duration Parameter Type'.
* @generated
*/
DurationParameterType createDurationParameterType();
/**
* Returns a new object of class 'Element Parameters'.
*
*
* @return a new object of class 'Element Parameters'.
* @generated
*/
ElementParameters createElementParameters();
/**
* Returns a new object of class 'Element Parameters Type'.
*
*
* @return a new object of class 'Element Parameters Type'.
* @generated
*/
ElementParametersType createElementParametersType();
/**
* Returns a new object of class 'Enum Parameter Type'.
*
*
* @return a new object of class 'Enum Parameter Type'.
* @generated
*/
EnumParameterType createEnumParameterType();
/**
* Returns a new object of class 'Erlang Distribution Type'.
*
*
* @return a new object of class 'Erlang Distribution Type'.
* @generated
*/
ErlangDistributionType createErlangDistributionType();
/**
* Returns a new object of class 'Expression Parameter Type'.
*
*
* @return a new object of class 'Expression Parameter Type'.
* @generated
*/
ExpressionParameterType createExpressionParameterType();
/**
* Returns a new object of class 'Floating Parameter Type'.
*
*
* @return a new object of class 'Floating Parameter Type'.
* @generated
*/
FloatingParameterType createFloatingParameterType();
/**
* Returns a new object of class 'Gamma Distribution Type'.
*
*
* @return a new object of class 'Gamma Distribution Type'.
* @generated
*/
GammaDistributionType createGammaDistributionType();
/**
* Returns a new object of class 'Log Normal Distribution Type'.
*
*
* @return a new object of class 'Log Normal Distribution Type'.
* @generated
*/
LogNormalDistributionType createLogNormalDistributionType();
/**
* Returns a new object of class 'Negative Exponential Distribution Type'.
*
*
* @return a new object of class 'Negative Exponential Distribution Type'.
* @generated
*/
NegativeExponentialDistributionType createNegativeExponentialDistributionType();
/**
* Returns a new object of class 'Normal Distribution Type'.
*
*
* @return a new object of class 'Normal Distribution Type'.
* @generated
*/
NormalDistributionType createNormalDistributionType();
/**
* Returns a new object of class 'Numeric Parameter Type'.
*
*
* @return a new object of class 'Numeric Parameter Type'.
* @generated
*/
NumericParameterType createNumericParameterType();
/**
* Returns a new object of class 'Parameter'.
*
*
* @return a new object of class 'Parameter'.
* @generated
*/
Parameter createParameter();
/**
* Returns a new object of class 'Parameter Value'.
*
*
* @return a new object of class 'Parameter Value'.
* @generated
*/
ParameterValue createParameterValue();
/**
* Returns a new object of class 'Poisson Distribution Type'.
*
*
* @return a new object of class 'Poisson Distribution Type'.
* @generated
*/
PoissonDistributionType createPoissonDistributionType();
/**
* Returns a new object of class 'Priority Parameters'.
*
*
* @return a new object of class 'Priority Parameters'.
* @generated
*/
PriorityParameters createPriorityParameters();
/**
* Returns a new object of class 'Property Parameters'.
*
*
* @return a new object of class 'Property Parameters'.
* @generated
*/
PropertyParameters createPropertyParameters();
/**
* Returns a new object of class 'Property Type'.
*
*
* @return a new object of class 'Property Type'.
* @generated
*/
PropertyType createPropertyType();
/**
* Returns a new object of class 'Resource Parameters'.
*
*
* @return a new object of class 'Resource Parameters'.
* @generated
*/
ResourceParameters createResourceParameters();
/**
* Returns a new object of class 'Scenario'.
*
*
* @return a new object of class 'Scenario'.
* @generated
*/
Scenario createScenario();
/**
* Returns a new object of class 'Scenario Parameters'.
*
*
* @return a new object of class 'Scenario Parameters'.
* @generated
*/
ScenarioParameters createScenarioParameters();
/**
* Returns a new object of class 'Scenario Parameters Type'.
*
*
* @return a new object of class 'Scenario Parameters Type'.
* @generated
*/
ScenarioParametersType createScenarioParametersType();
/**
* Returns a new object of class 'String Parameter Type'.
*
*
* @return a new object of class 'String Parameter Type'.
* @generated
*/
StringParameterType createStringParameterType();
/**
* Returns a new object of class 'Time Parameters'.
*
*
* @return a new object of class 'Time Parameters'.
* @generated
*/
TimeParameters createTimeParameters();
/**
* Returns a new object of class 'Triangular Distribution Type'.
*
*
* @return a new object of class 'Triangular Distribution Type'.
* @generated
*/
TriangularDistributionType createTriangularDistributionType();
/**
* Returns a new object of class 'Truncated Normal Distribution Type'.
*
*
* @return a new object of class 'Truncated Normal Distribution Type'.
* @generated
*/
TruncatedNormalDistributionType createTruncatedNormalDistributionType();
/**
* Returns a new object of class 'Uniform Distribution Type'.
*
*
* @return a new object of class 'Uniform Distribution Type'.
* @generated
*/
UniformDistributionType createUniformDistributionType();
/**
* Returns a new object of class 'User Distribution Data Point Type'.
*
*
* @return a new object of class 'User Distribution Data Point Type'.
* @generated
*/
UserDistributionDataPointType createUserDistributionDataPointType();
/**
* Returns a new object of class 'User Distribution Type'.
*
*
* @return a new object of class 'User Distribution Type'.
* @generated
*/
UserDistributionType createUserDistributionType();
/**
* Returns a new object of class 'Vendor Extension'.
*
*
* @return a new object of class 'Vendor Extension'.
* @generated
*/
VendorExtension createVendorExtension();
/**
* Returns a new object of class 'Weibull Distribution Type'.
*
*
* @return a new object of class 'Weibull Distribution Type'.
* @generated
*/
WeibullDistributionType createWeibullDistributionType();
/**
* Returns the package supported by this factory.
*
*
* @return the package supported by this factory.
* @generated
*/
BpsimPackage getBpsimPackage();
} //BpsimFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy