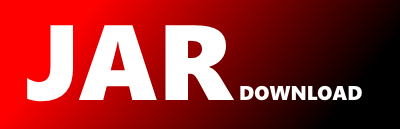
org.eclipse.bpmn2.di.DocumentRoot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*
*
* Copyright (c) 2010 SAP AG.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Reiner Hille-Doering (SAP AG) - initial API and implementation and/or initial documentation
*
*
*/
package org.eclipse.bpmn2.di;
import org.eclipse.emf.common.util.EMap;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.util.FeatureMap;
/**
*
* A representation of the model object 'Document Root'.
*
*
*
* The following features are supported:
*
*
* - {@link org.eclipse.bpmn2.di.DocumentRoot#getMixed Mixed}
* - {@link org.eclipse.bpmn2.di.DocumentRoot#getXMLNSPrefixMap XMLNS Prefix Map}
* - {@link org.eclipse.bpmn2.di.DocumentRoot#getXSISchemaLocation XSI Schema Location}
* - {@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNDiagram BPMN Diagram}
* - {@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNEdge BPMN Edge}
* - {@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNLabel BPMN Label}
* - {@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNLabelStyle BPMN Label Style}
* - {@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNPlane BPMN Plane}
* - {@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNShape BPMN Shape}
*
*
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot()
* @model extendedMetaData="name='' kind='mixed'"
* @generated
*/
public interface DocumentRoot extends EObject {
/**
* Returns the value of the 'Mixed' attribute list.
* The list contents are of type {@link org.eclipse.emf.ecore.util.FeatureMap.Entry}.
*
*
* @return the value of the 'Mixed' attribute list.
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot_Mixed()
* @model unique="false" dataType="org.eclipse.emf.ecore.EFeatureMapEntry" many="true"
* extendedMetaData="kind='elementWildcard' name=':mixed'"
* @generated
*/
FeatureMap getMixed();
/**
* Returns the value of the 'XMLNS Prefix Map' map.
* The key is of type {@link java.lang.String},
* and the value is of type {@link java.lang.String},
*
*
* @return the value of the 'XMLNS Prefix Map' map.
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot_XMLNSPrefixMap()
* @model mapType="org.eclipse.emf.ecore.EStringToStringMapEntry<org.eclipse.emf.ecore.EString, org.eclipse.emf.ecore.EString>" transient="true"
* extendedMetaData="kind='attribute' name='xmlns:prefix'"
* @generated
*/
EMap getXMLNSPrefixMap();
/**
* Returns the value of the 'XSI Schema Location' map.
* The key is of type {@link java.lang.String},
* and the value is of type {@link java.lang.String},
*
*
* @return the value of the 'XSI Schema Location' map.
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot_XSISchemaLocation()
* @model mapType="org.eclipse.emf.ecore.EStringToStringMapEntry<org.eclipse.emf.ecore.EString, org.eclipse.emf.ecore.EString>" transient="true"
* extendedMetaData="kind='attribute' name='xsi:schemaLocation'"
* @generated
*/
EMap getXSISchemaLocation();
/**
* Returns the value of the 'BPMN Diagram' containment reference.
*
*
* @return the value of the 'BPMN Diagram' containment reference.
* @see #setBPMNDiagram(BPMNDiagram)
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot_BPMNDiagram()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='BPMNDiagram' namespace='http://www.omg.org/spec/BPMN/20100524/DI'"
* @generated
*/
BPMNDiagram getBPMNDiagram();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNDiagram BPMN Diagram}' containment reference.
*
*
* @param value the new value of the 'BPMN Diagram' containment reference.
* @see #getBPMNDiagram()
* @generated
*/
void setBPMNDiagram(BPMNDiagram value);
/**
* Returns the value of the 'BPMN Edge' containment reference.
*
*
* @return the value of the 'BPMN Edge' containment reference.
* @see #setBPMNEdge(BPMNEdge)
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot_BPMNEdge()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='BPMNEdge' namespace='http://www.omg.org/spec/BPMN/20100524/DI' affiliation='http://www.omg.org/spec/DD/20100524/DI#DiagramElement'"
* @generated
*/
BPMNEdge getBPMNEdge();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNEdge BPMN Edge}' containment reference.
*
*
* @param value the new value of the 'BPMN Edge' containment reference.
* @see #getBPMNEdge()
* @generated
*/
void setBPMNEdge(BPMNEdge value);
/**
* Returns the value of the 'BPMN Label' containment reference.
*
*
* @return the value of the 'BPMN Label' containment reference.
* @see #setBPMNLabel(BPMNLabel)
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot_BPMNLabel()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='BPMNLabel' namespace='http://www.omg.org/spec/BPMN/20100524/DI'"
* @generated
*/
BPMNLabel getBPMNLabel();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNLabel BPMN Label}' containment reference.
*
*
* @param value the new value of the 'BPMN Label' containment reference.
* @see #getBPMNLabel()
* @generated
*/
void setBPMNLabel(BPMNLabel value);
/**
* Returns the value of the 'BPMN Label Style' containment reference.
*
*
* @return the value of the 'BPMN Label Style' containment reference.
* @see #setBPMNLabelStyle(BPMNLabelStyle)
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot_BPMNLabelStyle()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='BPMNLabelStyle' namespace='http://www.omg.org/spec/BPMN/20100524/DI'"
* @generated
*/
BPMNLabelStyle getBPMNLabelStyle();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNLabelStyle BPMN Label Style}' containment reference.
*
*
* @param value the new value of the 'BPMN Label Style' containment reference.
* @see #getBPMNLabelStyle()
* @generated
*/
void setBPMNLabelStyle(BPMNLabelStyle value);
/**
* Returns the value of the 'BPMN Plane' containment reference.
*
*
* @return the value of the 'BPMN Plane' containment reference.
* @see #setBPMNPlane(BPMNPlane)
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot_BPMNPlane()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='BPMNPlane' namespace='http://www.omg.org/spec/BPMN/20100524/DI'"
* @generated
*/
BPMNPlane getBPMNPlane();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNPlane BPMN Plane}' containment reference.
*
*
* @param value the new value of the 'BPMN Plane' containment reference.
* @see #getBPMNPlane()
* @generated
*/
void setBPMNPlane(BPMNPlane value);
/**
* Returns the value of the 'BPMN Shape' containment reference.
*
*
* @return the value of the 'BPMN Shape' containment reference.
* @see #setBPMNShape(BPMNShape)
* @see org.eclipse.bpmn2.di.BpmnDiPackage#getDocumentRoot_BPMNShape()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='BPMNShape' namespace='http://www.omg.org/spec/BPMN/20100524/DI' affiliation='http://www.omg.org/spec/DD/20100524/DI#DiagramElement'"
* @generated
*/
BPMNShape getBPMNShape();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.di.DocumentRoot#getBPMNShape BPMN Shape}' containment reference.
*
*
* @param value the new value of the 'BPMN Shape' containment reference.
* @see #getBPMNShape()
* @generated
*/
void setBPMNShape(BPMNShape value);
} // DocumentRoot
© 2015 - 2025 Weber Informatics LLC | Privacy Policy