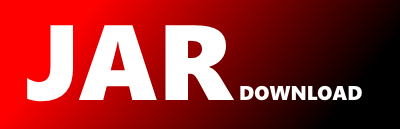
org.eclipse.dd.dc.DcPackage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*
*
* Copyright (c) 2010 SAP AG.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Reiner Hille-Doering (SAP AG) - initial API and implementation and/or initial documentation
*
*
*/
package org.eclipse.dd.dc;
import org.eclipse.emf.ecore.EAttribute;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.EReference;
/**
*
* The Package for the model.
* It contains accessors for the meta objects to represent
*
* - each class,
* - each feature of each class,
* - each enum,
* - and each data type
*
*
* @see org.eclipse.dd.dc.DcFactory
* @model kind="package"
* @generated
*/
public interface DcPackage extends EPackage {
/**
* The package name.
*
*
* @generated
*/
String eNAME = "dc";
/**
* The package namespace URI.
*
*
* @generated
*/
String eNS_URI = "http://www.omg.org/spec/DD/20100524/DC-XMI";
/**
* The package namespace name.
*
*
* @generated
*/
String eNS_PREFIX = "dc";
/**
* The singleton instance of the package.
*
*
* @generated
*/
DcPackage eINSTANCE = org.eclipse.dd.dc.impl.DcPackageImpl.init();
/**
* The meta object id for the '{@link org.eclipse.dd.dc.impl.DocumentRootImpl Document Root}' class.
*
*
* @see org.eclipse.dd.dc.impl.DocumentRootImpl
* @see org.eclipse.dd.dc.impl.DcPackageImpl#getDocumentRoot()
* @generated
*/
int DOCUMENT_ROOT = 0;
/**
* The feature id for the 'Mixed' attribute list.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__MIXED = 0;
/**
* The feature id for the 'XMLNS Prefix Map' map.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__XMLNS_PREFIX_MAP = 1;
/**
* The feature id for the 'XSI Schema Location' map.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__XSI_SCHEMA_LOCATION = 2;
/**
* The feature id for the 'Bounds' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__BOUNDS = 3;
/**
* The feature id for the 'Font' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__FONT = 4;
/**
* The feature id for the 'Point' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__POINT = 5;
/**
* The number of structural features of the 'Document Root' class.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT_FEATURE_COUNT = 6;
/**
* The meta object id for the '{@link org.eclipse.dd.dc.impl.BoundsImpl Bounds}' class.
*
*
* @see org.eclipse.dd.dc.impl.BoundsImpl
* @see org.eclipse.dd.dc.impl.DcPackageImpl#getBounds()
* @generated
*/
int BOUNDS = 1;
/**
* The feature id for the 'Height' attribute.
*
*
* @generated
* @ordered
*/
int BOUNDS__HEIGHT = 0;
/**
* The feature id for the 'Width' attribute.
*
*
* @generated
* @ordered
*/
int BOUNDS__WIDTH = 1;
/**
* The feature id for the 'X' attribute.
*
*
* @generated
* @ordered
*/
int BOUNDS__X = 2;
/**
* The feature id for the 'Y' attribute.
*
*
* @generated
* @ordered
*/
int BOUNDS__Y = 3;
/**
* The number of structural features of the 'Bounds' class.
*
*
* @generated
* @ordered
*/
int BOUNDS_FEATURE_COUNT = 4;
/**
* The meta object id for the '{@link org.eclipse.dd.dc.impl.FontImpl Font}' class.
*
*
* @see org.eclipse.dd.dc.impl.FontImpl
* @see org.eclipse.dd.dc.impl.DcPackageImpl#getFont()
* @generated
*/
int FONT = 2;
/**
* The feature id for the 'Is Bold' attribute.
*
*
* @generated
* @ordered
*/
int FONT__IS_BOLD = 0;
/**
* The feature id for the 'Is Italic' attribute.
*
*
* @generated
* @ordered
*/
int FONT__IS_ITALIC = 1;
/**
* The feature id for the 'Is Strike Through' attribute.
*
*
* @generated
* @ordered
*/
int FONT__IS_STRIKE_THROUGH = 2;
/**
* The feature id for the 'Is Underline' attribute.
*
*
* @generated
* @ordered
*/
int FONT__IS_UNDERLINE = 3;
/**
* The feature id for the 'Name' attribute.
*
*
* @generated
* @ordered
*/
int FONT__NAME = 4;
/**
* The feature id for the 'Size' attribute.
*
*
* @generated
* @ordered
*/
int FONT__SIZE = 5;
/**
* The number of structural features of the 'Font' class.
*
*
* @generated
* @ordered
*/
int FONT_FEATURE_COUNT = 6;
/**
* The meta object id for the '{@link org.eclipse.dd.dc.impl.PointImpl Point}' class.
*
*
* @see org.eclipse.dd.dc.impl.PointImpl
* @see org.eclipse.dd.dc.impl.DcPackageImpl#getPoint()
* @generated
*/
int POINT = 3;
/**
* The feature id for the 'X' attribute.
*
*
* @generated
* @ordered
*/
int POINT__X = 0;
/**
* The feature id for the 'Y' attribute.
*
*
* @generated
* @ordered
*/
int POINT__Y = 1;
/**
* The number of structural features of the 'Point' class.
*
*
* @generated
* @ordered
*/
int POINT_FEATURE_COUNT = 2;
/**
* Returns the meta object for class '{@link org.eclipse.dd.dc.DocumentRoot Document Root}'.
*
*
* @return the meta object for class 'Document Root'.
* @see org.eclipse.dd.dc.DocumentRoot
* @generated
*/
EClass getDocumentRoot();
/**
* Returns the meta object for the attribute list '{@link org.eclipse.dd.dc.DocumentRoot#getMixed Mixed}'.
*
*
* @return the meta object for the attribute list 'Mixed'.
* @see org.eclipse.dd.dc.DocumentRoot#getMixed()
* @see #getDocumentRoot()
* @generated
*/
EAttribute getDocumentRoot_Mixed();
/**
* Returns the meta object for the map '{@link org.eclipse.dd.dc.DocumentRoot#getXMLNSPrefixMap XMLNS Prefix Map}'.
*
*
* @return the meta object for the map 'XMLNS Prefix Map'.
* @see org.eclipse.dd.dc.DocumentRoot#getXMLNSPrefixMap()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_XMLNSPrefixMap();
/**
* Returns the meta object for the map '{@link org.eclipse.dd.dc.DocumentRoot#getXSISchemaLocation XSI Schema Location}'.
*
*
* @return the meta object for the map 'XSI Schema Location'.
* @see org.eclipse.dd.dc.DocumentRoot#getXSISchemaLocation()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_XSISchemaLocation();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.dd.dc.DocumentRoot#getBounds Bounds}'.
*
*
* @return the meta object for the containment reference 'Bounds'.
* @see org.eclipse.dd.dc.DocumentRoot#getBounds()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_Bounds();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.dd.dc.DocumentRoot#getFont Font}'.
*
*
* @return the meta object for the containment reference 'Font'.
* @see org.eclipse.dd.dc.DocumentRoot#getFont()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_Font();
/**
* Returns the meta object for the containment reference '{@link org.eclipse.dd.dc.DocumentRoot#getPoint Point}'.
*
*
* @return the meta object for the containment reference 'Point'.
* @see org.eclipse.dd.dc.DocumentRoot#getPoint()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_Point();
/**
* Returns the meta object for class '{@link org.eclipse.dd.dc.Bounds Bounds}'.
*
*
* @return the meta object for class 'Bounds'.
* @see org.eclipse.dd.dc.Bounds
* @generated
*/
EClass getBounds();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Bounds#getHeight Height}'.
*
*
* @return the meta object for the attribute 'Height'.
* @see org.eclipse.dd.dc.Bounds#getHeight()
* @see #getBounds()
* @generated
*/
EAttribute getBounds_Height();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Bounds#getWidth Width}'.
*
*
* @return the meta object for the attribute 'Width'.
* @see org.eclipse.dd.dc.Bounds#getWidth()
* @see #getBounds()
* @generated
*/
EAttribute getBounds_Width();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Bounds#getX X}'.
*
*
* @return the meta object for the attribute 'X'.
* @see org.eclipse.dd.dc.Bounds#getX()
* @see #getBounds()
* @generated
*/
EAttribute getBounds_X();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Bounds#getY Y}'.
*
*
* @return the meta object for the attribute 'Y'.
* @see org.eclipse.dd.dc.Bounds#getY()
* @see #getBounds()
* @generated
*/
EAttribute getBounds_Y();
/**
* Returns the meta object for class '{@link org.eclipse.dd.dc.Font Font}'.
*
*
* @return the meta object for class 'Font'.
* @see org.eclipse.dd.dc.Font
* @generated
*/
EClass getFont();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Font#isIsBold Is Bold}'.
*
*
* @return the meta object for the attribute 'Is Bold'.
* @see org.eclipse.dd.dc.Font#isIsBold()
* @see #getFont()
* @generated
*/
EAttribute getFont_IsBold();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Font#isIsItalic Is Italic}'.
*
*
* @return the meta object for the attribute 'Is Italic'.
* @see org.eclipse.dd.dc.Font#isIsItalic()
* @see #getFont()
* @generated
*/
EAttribute getFont_IsItalic();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Font#isIsStrikeThrough Is Strike Through}'.
*
*
* @return the meta object for the attribute 'Is Strike Through'.
* @see org.eclipse.dd.dc.Font#isIsStrikeThrough()
* @see #getFont()
* @generated
*/
EAttribute getFont_IsStrikeThrough();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Font#isIsUnderline Is Underline}'.
*
*
* @return the meta object for the attribute 'Is Underline'.
* @see org.eclipse.dd.dc.Font#isIsUnderline()
* @see #getFont()
* @generated
*/
EAttribute getFont_IsUnderline();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Font#getName Name}'.
*
*
* @return the meta object for the attribute 'Name'.
* @see org.eclipse.dd.dc.Font#getName()
* @see #getFont()
* @generated
*/
EAttribute getFont_Name();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Font#getSize Size}'.
*
*
* @return the meta object for the attribute 'Size'.
* @see org.eclipse.dd.dc.Font#getSize()
* @see #getFont()
* @generated
*/
EAttribute getFont_Size();
/**
* Returns the meta object for class '{@link org.eclipse.dd.dc.Point Point}'.
*
*
* @return the meta object for class 'Point'.
* @see org.eclipse.dd.dc.Point
* @generated
*/
EClass getPoint();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Point#getX X}'.
*
*
* @return the meta object for the attribute 'X'.
* @see org.eclipse.dd.dc.Point#getX()
* @see #getPoint()
* @generated
*/
EAttribute getPoint_X();
/**
* Returns the meta object for the attribute '{@link org.eclipse.dd.dc.Point#getY Y}'.
*
*
* @return the meta object for the attribute 'Y'.
* @see org.eclipse.dd.dc.Point#getY()
* @see #getPoint()
* @generated
*/
EAttribute getPoint_Y();
/**
* Returns the factory that creates the instances of the model.
*
*
* @return the factory that creates the instances of the model.
* @generated
*/
DcFactory getDcFactory();
/**
*
* Defines literals for the meta objects that represent
*
* - each class,
* - each feature of each class,
* - each enum,
* - and each data type
*
*
* @generated
*/
interface Literals {
/**
* The meta object literal for the '{@link org.eclipse.dd.dc.impl.DocumentRootImpl Document Root}' class.
*
*
* @see org.eclipse.dd.dc.impl.DocumentRootImpl
* @see org.eclipse.dd.dc.impl.DcPackageImpl#getDocumentRoot()
* @generated
*/
EClass DOCUMENT_ROOT = eINSTANCE.getDocumentRoot();
/**
* The meta object literal for the 'Mixed' attribute list feature.
*
*
* @generated
*/
EAttribute DOCUMENT_ROOT__MIXED = eINSTANCE.getDocumentRoot_Mixed();
/**
* The meta object literal for the 'XMLNS Prefix Map' map feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__XMLNS_PREFIX_MAP = eINSTANCE.getDocumentRoot_XMLNSPrefixMap();
/**
* The meta object literal for the 'XSI Schema Location' map feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__XSI_SCHEMA_LOCATION = eINSTANCE.getDocumentRoot_XSISchemaLocation();
/**
* The meta object literal for the 'Bounds' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__BOUNDS = eINSTANCE.getDocumentRoot_Bounds();
/**
* The meta object literal for the 'Font' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__FONT = eINSTANCE.getDocumentRoot_Font();
/**
* The meta object literal for the 'Point' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__POINT = eINSTANCE.getDocumentRoot_Point();
/**
* The meta object literal for the '{@link org.eclipse.dd.dc.impl.BoundsImpl Bounds}' class.
*
*
* @see org.eclipse.dd.dc.impl.BoundsImpl
* @see org.eclipse.dd.dc.impl.DcPackageImpl#getBounds()
* @generated
*/
EClass BOUNDS = eINSTANCE.getBounds();
/**
* The meta object literal for the 'Height' attribute feature.
*
*
* @generated
*/
EAttribute BOUNDS__HEIGHT = eINSTANCE.getBounds_Height();
/**
* The meta object literal for the 'Width' attribute feature.
*
*
* @generated
*/
EAttribute BOUNDS__WIDTH = eINSTANCE.getBounds_Width();
/**
* The meta object literal for the 'X' attribute feature.
*
*
* @generated
*/
EAttribute BOUNDS__X = eINSTANCE.getBounds_X();
/**
* The meta object literal for the 'Y' attribute feature.
*
*
* @generated
*/
EAttribute BOUNDS__Y = eINSTANCE.getBounds_Y();
/**
* The meta object literal for the '{@link org.eclipse.dd.dc.impl.FontImpl Font}' class.
*
*
* @see org.eclipse.dd.dc.impl.FontImpl
* @see org.eclipse.dd.dc.impl.DcPackageImpl#getFont()
* @generated
*/
EClass FONT = eINSTANCE.getFont();
/**
* The meta object literal for the 'Is Bold' attribute feature.
*
*
* @generated
*/
EAttribute FONT__IS_BOLD = eINSTANCE.getFont_IsBold();
/**
* The meta object literal for the 'Is Italic' attribute feature.
*
*
* @generated
*/
EAttribute FONT__IS_ITALIC = eINSTANCE.getFont_IsItalic();
/**
* The meta object literal for the 'Is Strike Through' attribute feature.
*
*
* @generated
*/
EAttribute FONT__IS_STRIKE_THROUGH = eINSTANCE.getFont_IsStrikeThrough();
/**
* The meta object literal for the 'Is Underline' attribute feature.
*
*
* @generated
*/
EAttribute FONT__IS_UNDERLINE = eINSTANCE.getFont_IsUnderline();
/**
* The meta object literal for the 'Name' attribute feature.
*
*
* @generated
*/
EAttribute FONT__NAME = eINSTANCE.getFont_Name();
/**
* The meta object literal for the 'Size' attribute feature.
*
*
* @generated
*/
EAttribute FONT__SIZE = eINSTANCE.getFont_Size();
/**
* The meta object literal for the '{@link org.eclipse.dd.dc.impl.PointImpl Point}' class.
*
*
* @see org.eclipse.dd.dc.impl.PointImpl
* @see org.eclipse.dd.dc.impl.DcPackageImpl#getPoint()
* @generated
*/
EClass POINT = eINSTANCE.getPoint();
/**
* The meta object literal for the 'X' attribute feature.
*
*
* @generated
*/
EAttribute POINT__X = eINSTANCE.getPoint_X();
/**
* The meta object literal for the 'Y' attribute feature.
*
*
* @generated
*/
EAttribute POINT__Y = eINSTANCE.getPoint_Y();
}
} //DcPackage
© 2015 - 2025 Weber Informatics LLC | Privacy Policy