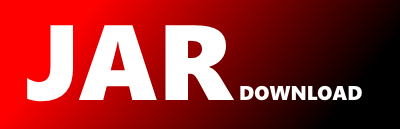
org.jboss.drools.DocumentRoot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package org.jboss.drools;
import java.math.BigInteger;
import org.eclipse.emf.common.util.EMap;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.util.FeatureMap;
/**
*
* A representation of the model object 'Document Root'.
*
*
*
* The following features are supported:
*
*
* - {@link org.jboss.drools.DocumentRoot#getMixed Mixed}
* - {@link org.jboss.drools.DocumentRoot#getXMLNSPrefixMap XMLNS Prefix Map}
* - {@link org.jboss.drools.DocumentRoot#getXSISchemaLocation XSI Schema Location}
* - {@link org.jboss.drools.DocumentRoot#getGlobal Global}
* - {@link org.jboss.drools.DocumentRoot#getImport Import}
* - {@link org.jboss.drools.DocumentRoot#getMetaData Meta Data}
* - {@link org.jboss.drools.DocumentRoot#getOnEntryScript On Entry Script}
* - {@link org.jboss.drools.DocumentRoot#getOnExitScript On Exit Script}
* - {@link org.jboss.drools.DocumentRoot#getPackageName Package Name}
* - {@link org.jboss.drools.DocumentRoot#getPriority Priority}
* - {@link org.jboss.drools.DocumentRoot#getRuleFlowGroup Rule Flow Group}
* - {@link org.jboss.drools.DocumentRoot#getTaskName Task Name}
* - {@link org.jboss.drools.DocumentRoot#getVersion Version}
*
*
* @see org.jboss.drools.DroolsPackage#getDocumentRoot()
* @model extendedMetaData="name='' kind='mixed'"
* @generated
*/
public interface DocumentRoot extends EObject {
/**
* Returns the value of the 'Mixed' attribute list.
* The list contents are of type {@link org.eclipse.emf.ecore.util.FeatureMap.Entry}.
*
*
* @return the value of the 'Mixed' attribute list.
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_Mixed()
* @model unique="false" dataType="org.eclipse.emf.ecore.EFeatureMapEntry" many="true"
* extendedMetaData="kind='elementWildcard' name=':mixed'"
* @generated
*/
FeatureMap getMixed();
/**
* Returns the value of the 'XMLNS Prefix Map' map.
* The key is of type {@link java.lang.String},
* and the value is of type {@link java.lang.String},
*
*
* @return the value of the 'XMLNS Prefix Map' map.
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_XMLNSPrefixMap()
* @model mapType="org.eclipse.emf.ecore.EStringToStringMapEntry<org.eclipse.emf.ecore.EString, org.eclipse.emf.ecore.EString>" transient="true"
* extendedMetaData="kind='attribute' name='xmlns:prefix'"
* @generated
*/
EMap getXMLNSPrefixMap();
/**
* Returns the value of the 'XSI Schema Location' map.
* The key is of type {@link java.lang.String},
* and the value is of type {@link java.lang.String},
*
*
* @return the value of the 'XSI Schema Location' map.
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_XSISchemaLocation()
* @model mapType="org.eclipse.emf.ecore.EStringToStringMapEntry<org.eclipse.emf.ecore.EString, org.eclipse.emf.ecore.EString>" transient="true"
* extendedMetaData="kind='attribute' name='xsi:schemaLocation'"
* @generated
*/
EMap getXSISchemaLocation();
/**
* Returns the value of the 'Global' containment reference.
*
*
* @return the value of the 'Global' containment reference.
* @see #setGlobal(GlobalType)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_Global()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='global' namespace='##targetNamespace'"
* @generated
*/
GlobalType getGlobal();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getGlobal Global}' containment reference.
*
*
* @param value the new value of the 'Global' containment reference.
* @see #getGlobal()
* @generated
*/
void setGlobal(GlobalType value);
/**
* Returns the value of the 'Import' containment reference.
*
*
* @return the value of the 'Import' containment reference.
* @see #setImport(ImportType)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_Import()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='import' namespace='##targetNamespace'"
* @generated
*/
ImportType getImport();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getImport Import}' containment reference.
*
*
* @param value the new value of the 'Import' containment reference.
* @see #getImport()
* @generated
*/
void setImport(ImportType value);
/**
* Returns the value of the 'Meta Data' containment reference.
*
*
* @return the value of the 'Meta Data' containment reference.
* @see #setMetaData(MetaDataType)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_MetaData()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='metaData' namespace='##targetNamespace'"
* @generated
*/
MetaDataType getMetaData();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getMetaData Meta Data}' containment reference.
*
*
* @param value the new value of the 'Meta Data' containment reference.
* @see #getMetaData()
* @generated
*/
void setMetaData(MetaDataType value);
/**
* Returns the value of the 'On Entry Script' containment reference.
*
*
* @return the value of the 'On Entry Script' containment reference.
* @see #setOnEntryScript(OnEntryScriptType)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_OnEntryScript()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='onEntry-script' namespace='##targetNamespace'"
* @generated
*/
OnEntryScriptType getOnEntryScript();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getOnEntryScript On Entry Script}' containment reference.
*
*
* @param value the new value of the 'On Entry Script' containment reference.
* @see #getOnEntryScript()
* @generated
*/
void setOnEntryScript(OnEntryScriptType value);
/**
* Returns the value of the 'On Exit Script' containment reference.
*
*
* @return the value of the 'On Exit Script' containment reference.
* @see #setOnExitScript(OnExitScriptType)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_OnExitScript()
* @model containment="true" upper="-2" transient="true" volatile="true" derived="true"
* extendedMetaData="kind='element' name='onExit-script' namespace='##targetNamespace'"
* @generated
*/
OnExitScriptType getOnExitScript();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getOnExitScript On Exit Script}' containment reference.
*
*
* @param value the new value of the 'On Exit Script' containment reference.
* @see #getOnExitScript()
* @generated
*/
void setOnExitScript(OnExitScriptType value);
/**
* Returns the value of the 'Package Name' attribute.
*
*
* @return the value of the 'Package Name' attribute.
* @see #setPackageName(String)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_PackageName()
* @model dataType="org.jboss.drools.PackageNameType"
* extendedMetaData="kind='attribute' name='packageName' namespace='##targetNamespace'"
* @generated
*/
String getPackageName();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getPackageName Package Name}' attribute.
*
*
* @param value the new value of the 'Package Name' attribute.
* @see #getPackageName()
* @generated
*/
void setPackageName(String value);
/**
* Returns the value of the 'Priority' attribute.
*
*
* @return the value of the 'Priority' attribute.
* @see #setPriority(BigInteger)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_Priority()
* @model dataType="org.jboss.drools.PriorityType"
* extendedMetaData="kind='attribute' name='priority' namespace='##targetNamespace'"
* @generated
*/
BigInteger getPriority();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getPriority Priority}' attribute.
*
*
* @param value the new value of the 'Priority' attribute.
* @see #getPriority()
* @generated
*/
void setPriority(BigInteger value);
/**
* Returns the value of the 'Rule Flow Group' attribute.
*
*
* @return the value of the 'Rule Flow Group' attribute.
* @see #setRuleFlowGroup(String)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_RuleFlowGroup()
* @model dataType="org.jboss.drools.RuleFlowGroupType"
* extendedMetaData="kind='attribute' name='ruleFlowGroup' namespace='##targetNamespace'"
* @generated
*/
String getRuleFlowGroup();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getRuleFlowGroup Rule Flow Group}' attribute.
*
*
* @param value the new value of the 'Rule Flow Group' attribute.
* @see #getRuleFlowGroup()
* @generated
*/
void setRuleFlowGroup(String value);
/**
* Returns the value of the 'Task Name' attribute.
*
*
* @return the value of the 'Task Name' attribute.
* @see #setTaskName(String)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_TaskName()
* @model dataType="org.jboss.drools.TaskNameType"
* extendedMetaData="kind='attribute' name='taskName' namespace='##targetNamespace'"
* @generated
*/
String getTaskName();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getTaskName Task Name}' attribute.
*
*
* @param value the new value of the 'Task Name' attribute.
* @see #getTaskName()
* @generated
*/
void setTaskName(String value);
/**
* Returns the value of the 'Version' attribute.
*
*
* @return the value of the 'Version' attribute.
* @see #setVersion(String)
* @see org.jboss.drools.DroolsPackage#getDocumentRoot_Version()
* @model dataType="org.jboss.drools.VersionType"
* extendedMetaData="kind='attribute' name='version' namespace='##targetNamespace'"
* @generated
*/
String getVersion();
/**
* Sets the value of the '{@link org.jboss.drools.DocumentRoot#getVersion Version}' attribute.
*
*
* @param value the new value of the 'Version' attribute.
* @see #getVersion()
* @generated
*/
void setVersion(String value);
} // DocumentRoot
© 2015 - 2025 Weber Informatics LLC | Privacy Policy