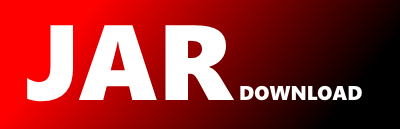
org.jboss.drools.util.DroolsSwitch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package org.jboss.drools.util;
import java.util.List;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EObject;
import org.jboss.drools.*;
/**
*
* The Switch for the model's inheritance hierarchy.
* It supports the call {@link #doSwitch(EObject) doSwitch(object)}
* to invoke the caseXXX
method for each class of the model,
* starting with the actual class of the object
* and proceeding up the inheritance hierarchy
* until a non-null result is returned,
* which is the result of the switch.
*
* @see org.jboss.drools.DroolsPackage
* @generated
*/
public class DroolsSwitch {
/**
* The cached model package
*
*
* @generated
*/
protected static DroolsPackage modelPackage;
/**
* Creates an instance of the switch.
*
*
* @generated
*/
public DroolsSwitch() {
if (modelPackage == null) {
modelPackage = DroolsPackage.eINSTANCE;
}
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
public T doSwitch(EObject theEObject) {
return doSwitch(theEObject.eClass(), theEObject);
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
protected T doSwitch(EClass theEClass, EObject theEObject) {
if (theEClass.eContainer() == modelPackage) {
return doSwitch(theEClass.getClassifierID(), theEObject);
}
else {
List eSuperTypes = theEClass.getESuperTypes();
return
eSuperTypes.isEmpty() ?
defaultCase(theEObject) :
doSwitch(eSuperTypes.get(0), theEObject);
}
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
protected T doSwitch(int classifierID, EObject theEObject) {
switch (classifierID) {
case DroolsPackage.DOCUMENT_ROOT: {
DocumentRoot documentRoot = (DocumentRoot)theEObject;
T result = caseDocumentRoot(documentRoot);
if (result == null) result = defaultCase(theEObject);
return result;
}
case DroolsPackage.GLOBAL_TYPE: {
GlobalType globalType = (GlobalType)theEObject;
T result = caseGlobalType(globalType);
if (result == null) result = defaultCase(theEObject);
return result;
}
case DroolsPackage.IMPORT_TYPE: {
ImportType importType = (ImportType)theEObject;
T result = caseImportType(importType);
if (result == null) result = defaultCase(theEObject);
return result;
}
case DroolsPackage.META_DATA_TYPE: {
MetaDataType metaDataType = (MetaDataType)theEObject;
T result = caseMetaDataType(metaDataType);
if (result == null) result = defaultCase(theEObject);
return result;
}
case DroolsPackage.ON_ENTRY_SCRIPT_TYPE: {
OnEntryScriptType onEntryScriptType = (OnEntryScriptType)theEObject;
T result = caseOnEntryScriptType(onEntryScriptType);
if (result == null) result = defaultCase(theEObject);
return result;
}
case DroolsPackage.ON_EXIT_SCRIPT_TYPE: {
OnExitScriptType onExitScriptType = (OnExitScriptType)theEObject;
T result = caseOnExitScriptType(onExitScriptType);
if (result == null) result = defaultCase(theEObject);
return result;
}
default: return defaultCase(theEObject);
}
}
/**
* Returns the result of interpreting the object as an instance of 'Document Root'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Document Root'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseDocumentRoot(DocumentRoot object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Global Type'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Global Type'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseGlobalType(GlobalType object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Import Type'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Import Type'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseImportType(ImportType object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Meta Data Type'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'Meta Data Type'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseMetaDataType(MetaDataType object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'On Entry Script Type'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'On Entry Script Type'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseOnEntryScriptType(OnEntryScriptType object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'On Exit Script Type'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'On Exit Script Type'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseOnExitScriptType(OnExitScriptType object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EObject'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch, but this is the last case anyway.
*
* @param object the target of the switch.
* @return the result of interpreting the object as an instance of 'EObject'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject)
* @generated
*/
public T defaultCase(EObject object) {
return null;
}
} //DroolsSwitch
© 2015 - 2025 Weber Informatics LLC | Privacy Policy