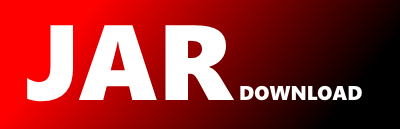
bpsim.ElementParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package bpsim;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EObject;
/**
*
* A representation of the model object 'Element Parameters'.
*
*
*
* The following features are supported:
*
*
* - {@link bpsim.ElementParameters#getTimeParameters Time Parameters}
* - {@link bpsim.ElementParameters#getControlParameters Control Parameters}
* - {@link bpsim.ElementParameters#getResourceParameters Resource Parameters}
* - {@link bpsim.ElementParameters#getPriorityParameters Priority Parameters}
* - {@link bpsim.ElementParameters#getCostParameters Cost Parameters}
* - {@link bpsim.ElementParameters#getPropertyParameters Property Parameters}
* - {@link bpsim.ElementParameters#getVendorExtension Vendor Extension}
* - {@link bpsim.ElementParameters#getElementRef Element Ref}
* - {@link bpsim.ElementParameters#getId Id}
*
*
* @see bpsim.BpsimPackage#getElementParameters()
* @model extendedMetaData="name='ElementParameters' kind='elementOnly'"
* @generated
*/
public interface ElementParameters extends EObject {
/**
* Returns the value of the 'Time Parameters' containment reference.
*
*
* @return the value of the 'Time Parameters' containment reference.
* @see #setTimeParameters(TimeParameters)
* @see bpsim.BpsimPackage#getElementParameters_TimeParameters()
* @model containment="true"
* extendedMetaData="kind='element' name='TimeParameters' namespace='##targetNamespace'"
* @generated
*/
TimeParameters getTimeParameters();
/**
* Sets the value of the '{@link bpsim.ElementParameters#getTimeParameters Time Parameters}' containment reference.
*
*
* @param value the new value of the 'Time Parameters' containment reference.
* @see #getTimeParameters()
* @generated
*/
void setTimeParameters(TimeParameters value);
/**
* Returns the value of the 'Control Parameters' containment reference.
*
*
* @return the value of the 'Control Parameters' containment reference.
* @see #setControlParameters(ControlParameters)
* @see bpsim.BpsimPackage#getElementParameters_ControlParameters()
* @model containment="true"
* extendedMetaData="kind='element' name='ControlParameters' namespace='##targetNamespace'"
* @generated
*/
ControlParameters getControlParameters();
/**
* Sets the value of the '{@link bpsim.ElementParameters#getControlParameters Control Parameters}' containment reference.
*
*
* @param value the new value of the 'Control Parameters' containment reference.
* @see #getControlParameters()
* @generated
*/
void setControlParameters(ControlParameters value);
/**
* Returns the value of the 'Resource Parameters' containment reference.
*
*
* @return the value of the 'Resource Parameters' containment reference.
* @see #setResourceParameters(ResourceParameters)
* @see bpsim.BpsimPackage#getElementParameters_ResourceParameters()
* @model containment="true"
* extendedMetaData="kind='element' name='ResourceParameters' namespace='##targetNamespace'"
* @generated
*/
ResourceParameters getResourceParameters();
/**
* Sets the value of the '{@link bpsim.ElementParameters#getResourceParameters Resource Parameters}' containment reference.
*
*
* @param value the new value of the 'Resource Parameters' containment reference.
* @see #getResourceParameters()
* @generated
*/
void setResourceParameters(ResourceParameters value);
/**
* Returns the value of the 'Priority Parameters' containment reference.
*
*
* @return the value of the 'Priority Parameters' containment reference.
* @see #setPriorityParameters(PriorityParameters)
* @see bpsim.BpsimPackage#getElementParameters_PriorityParameters()
* @model containment="true"
* extendedMetaData="kind='element' name='PriorityParameters' namespace='##targetNamespace'"
* @generated
*/
PriorityParameters getPriorityParameters();
/**
* Sets the value of the '{@link bpsim.ElementParameters#getPriorityParameters Priority Parameters}' containment reference.
*
*
* @param value the new value of the 'Priority Parameters' containment reference.
* @see #getPriorityParameters()
* @generated
*/
void setPriorityParameters(PriorityParameters value);
/**
* Returns the value of the 'Cost Parameters' containment reference.
*
*
* @return the value of the 'Cost Parameters' containment reference.
* @see #setCostParameters(CostParameters)
* @see bpsim.BpsimPackage#getElementParameters_CostParameters()
* @model containment="true"
* extendedMetaData="kind='element' name='CostParameters' namespace='##targetNamespace'"
* @generated
*/
CostParameters getCostParameters();
/**
* Sets the value of the '{@link bpsim.ElementParameters#getCostParameters Cost Parameters}' containment reference.
*
*
* @param value the new value of the 'Cost Parameters' containment reference.
* @see #getCostParameters()
* @generated
*/
void setCostParameters(CostParameters value);
/**
* Returns the value of the 'Property Parameters' containment reference.
*
*
* @return the value of the 'Property Parameters' containment reference.
* @see #setPropertyParameters(PropertyParameters)
* @see bpsim.BpsimPackage#getElementParameters_PropertyParameters()
* @model containment="true"
* extendedMetaData="kind='element' name='PropertyParameters' namespace='##targetNamespace'"
* @generated
*/
PropertyParameters getPropertyParameters();
/**
* Sets the value of the '{@link bpsim.ElementParameters#getPropertyParameters Property Parameters}' containment reference.
*
*
* @param value the new value of the 'Property Parameters' containment reference.
* @see #getPropertyParameters()
* @generated
*/
void setPropertyParameters(PropertyParameters value);
/**
* Returns the value of the 'Vendor Extension' containment reference list.
* The list contents are of type {@link bpsim.VendorExtension}.
*
*
* @return the value of the 'Vendor Extension' containment reference list.
* @see bpsim.BpsimPackage#getElementParameters_VendorExtension()
* @model containment="true"
* extendedMetaData="kind='element' name='VendorExtension' namespace='##targetNamespace'"
* @generated
*/
EList getVendorExtension();
/**
* Returns the value of the 'Element Ref' attribute.
*
*
* @return the value of the 'Element Ref' attribute.
* @see #setElementRef(String)
* @see bpsim.BpsimPackage#getElementParameters_ElementRef()
* @model dataType="org.eclipse.emf.ecore.xml.type.ID"
* extendedMetaData="kind='attribute' name='elementRef'"
* @generated
*/
String getElementRef();
/**
* Sets the value of the '{@link bpsim.ElementParameters#getElementRef Element Ref}' attribute.
*
*
* @param value the new value of the 'Element Ref' attribute.
* @see #getElementRef()
* @generated
*/
void setElementRef(String value);
/**
* Returns the value of the 'Id' attribute.
*
*
* @return the value of the 'Id' attribute.
* @see #setId(String)
* @see bpsim.BpsimPackage#getElementParameters_Id()
* @model id="true" dataType="org.eclipse.emf.ecore.xml.type.ID"
* extendedMetaData="kind='attribute' name='id'"
* @generated
*/
String getId();
/**
* Sets the value of the '{@link bpsim.ElementParameters#getId Id}' attribute.
*
*
* @param value the new value of the 'Id' attribute.
* @see #getId()
* @generated
*/
void setId(String value);
} // ElementParameters
© 2015 - 2025 Weber Informatics LLC | Privacy Policy