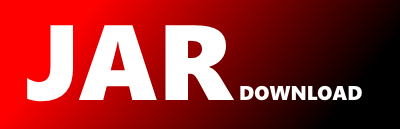
bpsim.Scenario Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package bpsim;
import javax.xml.datatype.XMLGregorianCalendar;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EObject;
/**
*
* A representation of the model object 'Scenario'.
*
*
*
* The following features are supported:
*
*
* - {@link bpsim.Scenario#getScenarioParameters Scenario Parameters}
* - {@link bpsim.Scenario#getElementParameters Element Parameters}
* - {@link bpsim.Scenario#getCalendar Calendar}
* - {@link bpsim.Scenario#getVendorExtension Vendor Extension}
* - {@link bpsim.Scenario#getAuthor Author}
* - {@link bpsim.Scenario#getCreated Created}
* - {@link bpsim.Scenario#getDescription Description}
* - {@link bpsim.Scenario#getId Id}
* - {@link bpsim.Scenario#getInherits Inherits}
* - {@link bpsim.Scenario#getModified Modified}
* - {@link bpsim.Scenario#getName Name}
* - {@link bpsim.Scenario#getResult Result}
* - {@link bpsim.Scenario#getVendor Vendor}
* - {@link bpsim.Scenario#getVersion Version}
*
*
* @see bpsim.BpsimPackage#getScenario()
* @model extendedMetaData="name='Scenario' kind='elementOnly'"
* @generated
*/
public interface Scenario extends EObject {
/**
* Returns the value of the 'Scenario Parameters' containment reference.
*
*
* @return the value of the 'Scenario Parameters' containment reference.
* @see #setScenarioParameters(ScenarioParameters)
* @see bpsim.BpsimPackage#getScenario_ScenarioParameters()
* @model containment="true"
* extendedMetaData="kind='element' name='ScenarioParameters' namespace='##targetNamespace'"
* @generated
*/
ScenarioParameters getScenarioParameters();
/**
* Sets the value of the '{@link bpsim.Scenario#getScenarioParameters Scenario Parameters}' containment reference.
*
*
* @param value the new value of the 'Scenario Parameters' containment reference.
* @see #getScenarioParameters()
* @generated
*/
void setScenarioParameters(ScenarioParameters value);
/**
* Returns the value of the 'Element Parameters' containment reference list.
* The list contents are of type {@link bpsim.ElementParameters}.
*
*
* @return the value of the 'Element Parameters' containment reference list.
* @see bpsim.BpsimPackage#getScenario_ElementParameters()
* @model containment="true"
* extendedMetaData="kind='element' name='ElementParameters' namespace='##targetNamespace'"
* @generated
*/
EList getElementParameters();
/**
* Returns the value of the 'Calendar' containment reference list.
* The list contents are of type {@link bpsim.Calendar}.
*
*
* @return the value of the 'Calendar' containment reference list.
* @see bpsim.BpsimPackage#getScenario_Calendar()
* @model containment="true"
* extendedMetaData="kind='element' name='Calendar' namespace='##targetNamespace'"
* @generated
*/
EList getCalendar();
/**
* Returns the value of the 'Vendor Extension' containment reference list.
* The list contents are of type {@link bpsim.VendorExtension}.
*
*
* @return the value of the 'Vendor Extension' containment reference list.
* @see bpsim.BpsimPackage#getScenario_VendorExtension()
* @model containment="true"
* extendedMetaData="kind='element' name='VendorExtension' namespace='##targetNamespace'"
* @generated
*/
EList getVendorExtension();
/**
* Returns the value of the 'Author' attribute.
*
*
* @return the value of the 'Author' attribute.
* @see #setAuthor(String)
* @see bpsim.BpsimPackage#getScenario_Author()
* @model dataType="org.eclipse.emf.ecore.xml.type.String"
* extendedMetaData="kind='attribute' name='author'"
* @generated
*/
String getAuthor();
/**
* Sets the value of the '{@link bpsim.Scenario#getAuthor Author}' attribute.
*
*
* @param value the new value of the 'Author' attribute.
* @see #getAuthor()
* @generated
*/
void setAuthor(String value);
/**
* Returns the value of the 'Created' attribute.
*
*
* @return the value of the 'Created' attribute.
* @see #setCreated(XMLGregorianCalendar)
* @see bpsim.BpsimPackage#getScenario_Created()
* @model dataType="org.eclipse.emf.ecore.xml.type.DateTime"
* extendedMetaData="kind='attribute' name='created'"
* @generated
*/
XMLGregorianCalendar getCreated();
/**
* Sets the value of the '{@link bpsim.Scenario#getCreated Created}' attribute.
*
*
* @param value the new value of the 'Created' attribute.
* @see #getCreated()
* @generated
*/
void setCreated(XMLGregorianCalendar value);
/**
* Returns the value of the 'Description' attribute.
*
*
* @return the value of the 'Description' attribute.
* @see #setDescription(String)
* @see bpsim.BpsimPackage#getScenario_Description()
* @model dataType="org.eclipse.emf.ecore.xml.type.String"
* extendedMetaData="kind='attribute' name='description'"
* @generated
*/
String getDescription();
/**
* Sets the value of the '{@link bpsim.Scenario#getDescription Description}' attribute.
*
*
* @param value the new value of the 'Description' attribute.
* @see #getDescription()
* @generated
*/
void setDescription(String value);
/**
* Returns the value of the 'Id' attribute.
*
*
* @return the value of the 'Id' attribute.
* @see #setId(String)
* @see bpsim.BpsimPackage#getScenario_Id()
* @model id="true" dataType="org.eclipse.emf.ecore.xml.type.ID" required="true"
* extendedMetaData="kind='attribute' name='id'"
* @generated
*/
String getId();
/**
* Sets the value of the '{@link bpsim.Scenario#getId Id}' attribute.
*
*
* @param value the new value of the 'Id' attribute.
* @see #getId()
* @generated
*/
void setId(String value);
/**
* Returns the value of the 'Inherits' attribute.
*
*
* @return the value of the 'Inherits' attribute.
* @see #setInherits(String)
* @see bpsim.BpsimPackage#getScenario_Inherits()
* @model dataType="org.eclipse.emf.ecore.xml.type.IDREF"
* extendedMetaData="kind='attribute' name='inherits'"
* @generated
*/
String getInherits();
/**
* Sets the value of the '{@link bpsim.Scenario#getInherits Inherits}' attribute.
*
*
* @param value the new value of the 'Inherits' attribute.
* @see #getInherits()
* @generated
*/
void setInherits(String value);
/**
* Returns the value of the 'Modified' attribute.
*
*
* @return the value of the 'Modified' attribute.
* @see #setModified(XMLGregorianCalendar)
* @see bpsim.BpsimPackage#getScenario_Modified()
* @model dataType="org.eclipse.emf.ecore.xml.type.DateTime"
* extendedMetaData="kind='attribute' name='modified'"
* @generated
*/
XMLGregorianCalendar getModified();
/**
* Sets the value of the '{@link bpsim.Scenario#getModified Modified}' attribute.
*
*
* @param value the new value of the 'Modified' attribute.
* @see #getModified()
* @generated
*/
void setModified(XMLGregorianCalendar value);
/**
* Returns the value of the 'Name' attribute.
*
*
* @return the value of the 'Name' attribute.
* @see #setName(String)
* @see bpsim.BpsimPackage#getScenario_Name()
* @model dataType="org.eclipse.emf.ecore.xml.type.String"
* extendedMetaData="kind='attribute' name='name'"
* @generated
*/
String getName();
/**
* Sets the value of the '{@link bpsim.Scenario#getName Name}' attribute.
*
*
* @param value the new value of the 'Name' attribute.
* @see #getName()
* @generated
*/
void setName(String value);
/**
* Returns the value of the 'Result' attribute.
*
*
* @return the value of the 'Result' attribute.
* @see #setResult(String)
* @see bpsim.BpsimPackage#getScenario_Result()
* @model dataType="org.eclipse.emf.ecore.xml.type.IDREF"
* extendedMetaData="kind='attribute' name='result'"
* @generated
*/
String getResult();
/**
* Sets the value of the '{@link bpsim.Scenario#getResult Result}' attribute.
*
*
* @param value the new value of the 'Result' attribute.
* @see #getResult()
* @generated
*/
void setResult(String value);
/**
* Returns the value of the 'Vendor' attribute.
*
*
* @return the value of the 'Vendor' attribute.
* @see #setVendor(String)
* @see bpsim.BpsimPackage#getScenario_Vendor()
* @model dataType="org.eclipse.emf.ecore.xml.type.String"
* extendedMetaData="kind='attribute' name='vendor'"
* @generated
*/
String getVendor();
/**
* Sets the value of the '{@link bpsim.Scenario#getVendor Vendor}' attribute.
*
*
* @param value the new value of the 'Vendor' attribute.
* @see #getVendor()
* @generated
*/
void setVendor(String value);
/**
* Returns the value of the 'Version' attribute.
*
*
* @return the value of the 'Version' attribute.
* @see #setVersion(String)
* @see bpsim.BpsimPackage#getScenario_Version()
* @model dataType="org.eclipse.emf.ecore.xml.type.String"
* extendedMetaData="kind='attribute' name='version'"
* @generated
*/
String getVersion();
/**
* Sets the value of the '{@link bpsim.Scenario#getVersion Version}' attribute.
*
*
* @param value the new value of the 'Version' attribute.
* @see #getVersion()
* @generated
*/
void setVersion(String value);
} // Scenario
© 2015 - 2025 Weber Informatics LLC | Privacy Policy