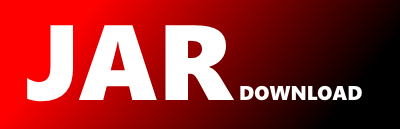
bpsim.ScenarioParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package bpsim;
import org.eclipse.emf.ecore.EObject;
/**
*
* A representation of the model object 'Scenario Parameters'.
*
*
*
* The following features are supported:
*
*
* - {@link bpsim.ScenarioParameters#getStart Start}
* - {@link bpsim.ScenarioParameters#getDuration Duration}
* - {@link bpsim.ScenarioParameters#getPropertyParameters Property Parameters}
* - {@link bpsim.ScenarioParameters#getBaseCurrencyUnit Base Currency Unit}
* - {@link bpsim.ScenarioParameters#getBaseTimeUnit Base Time Unit}
* - {@link bpsim.ScenarioParameters#getReplication Replication}
* - {@link bpsim.ScenarioParameters#getSeed Seed}
*
*
* @see bpsim.BpsimPackage#getScenarioParameters()
* @model extendedMetaData="name='ScenarioParameters' kind='elementOnly'"
* @generated
*/
public interface ScenarioParameters extends EObject {
/**
* Returns the value of the 'Start' containment reference.
*
*
* @return the value of the 'Start' containment reference.
* @see #setStart(Parameter)
* @see bpsim.BpsimPackage#getScenarioParameters_Start()
* @model containment="true"
* extendedMetaData="kind='element' name='Start' namespace='##targetNamespace'"
* @generated
*/
Parameter getStart();
/**
* Sets the value of the '{@link bpsim.ScenarioParameters#getStart Start}' containment reference.
*
*
* @param value the new value of the 'Start' containment reference.
* @see #getStart()
* @generated
*/
void setStart(Parameter value);
/**
* Returns the value of the 'Duration' containment reference.
*
*
* @return the value of the 'Duration' containment reference.
* @see #setDuration(Parameter)
* @see bpsim.BpsimPackage#getScenarioParameters_Duration()
* @model containment="true"
* extendedMetaData="kind='element' name='Duration' namespace='##targetNamespace'"
* @generated
*/
Parameter getDuration();
/**
* Sets the value of the '{@link bpsim.ScenarioParameters#getDuration Duration}' containment reference.
*
*
* @param value the new value of the 'Duration' containment reference.
* @see #getDuration()
* @generated
*/
void setDuration(Parameter value);
/**
* Returns the value of the 'Property Parameters' containment reference.
*
*
* @return the value of the 'Property Parameters' containment reference.
* @see #setPropertyParameters(PropertyParameters)
* @see bpsim.BpsimPackage#getScenarioParameters_PropertyParameters()
* @model containment="true"
* extendedMetaData="kind='element' name='PropertyParameters' namespace='##targetNamespace'"
* @generated
*/
PropertyParameters getPropertyParameters();
/**
* Sets the value of the '{@link bpsim.ScenarioParameters#getPropertyParameters Property Parameters}' containment reference.
*
*
* @param value the new value of the 'Property Parameters' containment reference.
* @see #getPropertyParameters()
* @generated
*/
void setPropertyParameters(PropertyParameters value);
/**
* Returns the value of the 'Base Currency Unit' attribute.
*
*
* @return the value of the 'Base Currency Unit' attribute.
* @see #setBaseCurrencyUnit(String)
* @see bpsim.BpsimPackage#getScenarioParameters_BaseCurrencyUnit()
* @model dataType="org.eclipse.emf.ecore.xml.type.String"
* extendedMetaData="kind='attribute' name='baseCurrencyUnit'"
* @generated
*/
String getBaseCurrencyUnit();
/**
* Sets the value of the '{@link bpsim.ScenarioParameters#getBaseCurrencyUnit Base Currency Unit}' attribute.
*
*
* @param value the new value of the 'Base Currency Unit' attribute.
* @see #getBaseCurrencyUnit()
* @generated
*/
void setBaseCurrencyUnit(String value);
/**
* Returns the value of the 'Base Time Unit' attribute.
* The literals are from the enumeration {@link bpsim.TimeUnit}.
*
*
* @return the value of the 'Base Time Unit' attribute.
* @see bpsim.TimeUnit
* @see #isSetBaseTimeUnit()
* @see #unsetBaseTimeUnit()
* @see #setBaseTimeUnit(TimeUnit)
* @see bpsim.BpsimPackage#getScenarioParameters_BaseTimeUnit()
* @model unsettable="true"
* extendedMetaData="kind='attribute' name='baseTimeUnit'"
* @generated
*/
TimeUnit getBaseTimeUnit();
/**
* Sets the value of the '{@link bpsim.ScenarioParameters#getBaseTimeUnit Base Time Unit}' attribute.
*
*
* @param value the new value of the 'Base Time Unit' attribute.
* @see bpsim.TimeUnit
* @see #isSetBaseTimeUnit()
* @see #unsetBaseTimeUnit()
* @see #getBaseTimeUnit()
* @generated
*/
void setBaseTimeUnit(TimeUnit value);
/**
* Unsets the value of the '{@link bpsim.ScenarioParameters#getBaseTimeUnit Base Time Unit}' attribute.
*
*
* @see #isSetBaseTimeUnit()
* @see #getBaseTimeUnit()
* @see #setBaseTimeUnit(TimeUnit)
* @generated
*/
void unsetBaseTimeUnit();
/**
* Returns whether the value of the '{@link bpsim.ScenarioParameters#getBaseTimeUnit Base Time Unit}' attribute is set.
*
*
* @return whether the value of the 'Base Time Unit' attribute is set.
* @see #unsetBaseTimeUnit()
* @see #getBaseTimeUnit()
* @see #setBaseTimeUnit(TimeUnit)
* @generated
*/
boolean isSetBaseTimeUnit();
/**
* Returns the value of the 'Replication' attribute.
*
*
* @return the value of the 'Replication' attribute.
* @see #isSetReplication()
* @see #unsetReplication()
* @see #setReplication(int)
* @see bpsim.BpsimPackage#getScenarioParameters_Replication()
* @model unsettable="true" dataType="org.eclipse.emf.ecore.xml.type.Int"
* extendedMetaData="kind='attribute' name='replication'"
* @generated
*/
int getReplication();
/**
* Sets the value of the '{@link bpsim.ScenarioParameters#getReplication Replication}' attribute.
*
*
* @param value the new value of the 'Replication' attribute.
* @see #isSetReplication()
* @see #unsetReplication()
* @see #getReplication()
* @generated
*/
void setReplication(int value);
/**
* Unsets the value of the '{@link bpsim.ScenarioParameters#getReplication Replication}' attribute.
*
*
* @see #isSetReplication()
* @see #getReplication()
* @see #setReplication(int)
* @generated
*/
void unsetReplication();
/**
* Returns whether the value of the '{@link bpsim.ScenarioParameters#getReplication Replication}' attribute is set.
*
*
* @return whether the value of the 'Replication' attribute is set.
* @see #unsetReplication()
* @see #getReplication()
* @see #setReplication(int)
* @generated
*/
boolean isSetReplication();
/**
* Returns the value of the 'Seed' attribute.
*
*
* @return the value of the 'Seed' attribute.
* @see #isSetSeed()
* @see #unsetSeed()
* @see #setSeed(long)
* @see bpsim.BpsimPackage#getScenarioParameters_Seed()
* @model unsettable="true" dataType="org.eclipse.emf.ecore.xml.type.Long"
* extendedMetaData="kind='attribute' name='seed'"
* @generated
*/
long getSeed();
/**
* Sets the value of the '{@link bpsim.ScenarioParameters#getSeed Seed}' attribute.
*
*
* @param value the new value of the 'Seed' attribute.
* @see #isSetSeed()
* @see #unsetSeed()
* @see #getSeed()
* @generated
*/
void setSeed(long value);
/**
* Unsets the value of the '{@link bpsim.ScenarioParameters#getSeed Seed}' attribute.
*
*
* @see #isSetSeed()
* @see #getSeed()
* @see #setSeed(long)
* @generated
*/
void unsetSeed();
/**
* Returns whether the value of the '{@link bpsim.ScenarioParameters#getSeed Seed}' attribute is set.
*
*
* @return whether the value of the 'Seed' attribute is set.
* @see #unsetSeed()
* @see #getSeed()
* @see #setSeed(long)
* @generated
*/
boolean isSetSeed();
} // ScenarioParameters
© 2015 - 2025 Weber Informatics LLC | Privacy Policy