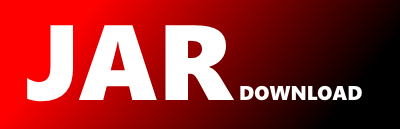
bpsim.impl.ScenarioParametersImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package bpsim.impl;
import bpsim.BpsimPackage;
import bpsim.Parameter;
import bpsim.PropertyParameters;
import bpsim.ScenarioParameters;
import bpsim.TimeUnit;
import com.google.gwt.user.client.rpc.GwtTransient;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.EObjectImpl;
/**
*
* An implementation of the model object 'Scenario Parameters'.
*
*
* The following features are implemented:
*
*
* - {@link bpsim.impl.ScenarioParametersImpl#getStart Start}
* - {@link bpsim.impl.ScenarioParametersImpl#getDuration Duration}
* - {@link bpsim.impl.ScenarioParametersImpl#getPropertyParameters Property Parameters}
* - {@link bpsim.impl.ScenarioParametersImpl#getBaseCurrencyUnit Base Currency Unit}
* - {@link bpsim.impl.ScenarioParametersImpl#getBaseTimeUnit Base Time Unit}
* - {@link bpsim.impl.ScenarioParametersImpl#getReplication Replication}
* - {@link bpsim.impl.ScenarioParametersImpl#getSeed Seed}
*
*
* @generated
*/
public class ScenarioParametersImpl extends EObjectImpl implements ScenarioParameters {
/**
* The cached value of the '{@link #getStart() Start}' containment reference.
*
*
* @see #getStart()
* @generated
* @ordered
*/
@GwtTransient
protected Parameter start;
/**
* The cached value of the '{@link #getDuration() Duration}' containment reference.
*
*
* @see #getDuration()
* @generated
* @ordered
*/
@GwtTransient
protected Parameter duration;
/**
* The cached value of the '{@link #getPropertyParameters() Property Parameters}' containment reference.
*
*
* @see #getPropertyParameters()
* @generated
* @ordered
*/
@GwtTransient
protected PropertyParameters propertyParameters;
/**
* The default value of the '{@link #getBaseCurrencyUnit() Base Currency Unit}' attribute.
*
*
* @see #getBaseCurrencyUnit()
* @generated
* @ordered
*/
protected static final String BASE_CURRENCY_UNIT_EDEFAULT = null;
/**
* The cached value of the '{@link #getBaseCurrencyUnit() Base Currency Unit}' attribute.
*
*
* @see #getBaseCurrencyUnit()
* @generated
* @ordered
*/
@GwtTransient
protected String baseCurrencyUnit = BASE_CURRENCY_UNIT_EDEFAULT;
/**
* The default value of the '{@link #getBaseTimeUnit() Base Time Unit}' attribute.
*
*
* @see #getBaseTimeUnit()
* @generated
* @ordered
*/
protected static final TimeUnit BASE_TIME_UNIT_EDEFAULT = TimeUnit.MS;
/**
* The cached value of the '{@link #getBaseTimeUnit() Base Time Unit}' attribute.
*
*
* @see #getBaseTimeUnit()
* @generated
* @ordered
*/
@GwtTransient
protected TimeUnit baseTimeUnit = BASE_TIME_UNIT_EDEFAULT;
/**
* This is true if the Base Time Unit attribute has been set.
*
*
* @generated
* @ordered
*/
@GwtTransient
protected boolean baseTimeUnitESet;
/**
* The default value of the '{@link #getReplication() Replication}' attribute.
*
*
* @see #getReplication()
* @generated
* @ordered
*/
protected static final int REPLICATION_EDEFAULT = 0;
/**
* The cached value of the '{@link #getReplication() Replication}' attribute.
*
*
* @see #getReplication()
* @generated
* @ordered
*/
@GwtTransient
protected int replication = REPLICATION_EDEFAULT;
/**
* This is true if the Replication attribute has been set.
*
*
* @generated
* @ordered
*/
@GwtTransient
protected boolean replicationESet;
/**
* The default value of the '{@link #getSeed() Seed}' attribute.
*
*
* @see #getSeed()
* @generated
* @ordered
*/
protected static final long SEED_EDEFAULT = 0L;
/**
* The cached value of the '{@link #getSeed() Seed}' attribute.
*
*
* @see #getSeed()
* @generated
* @ordered
*/
@GwtTransient
protected long seed = SEED_EDEFAULT;
/**
* This is true if the Seed attribute has been set.
*
*
* @generated
* @ordered
*/
@GwtTransient
protected boolean seedESet;
/**
*
*
* @generated
*/
protected ScenarioParametersImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return BpsimPackage.Literals.SCENARIO_PARAMETERS;
}
/**
*
*
* @generated
*/
@Override
public Parameter getStart() {
return start;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetStart(Parameter newStart, NotificationChain msgs) {
Parameter oldStart = start;
start = newStart;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__START, oldStart, newStart);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setStart(Parameter newStart) {
if (newStart != start) {
NotificationChain msgs = null;
if (start != null)
msgs = ((InternalEObject)start).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.SCENARIO_PARAMETERS__START, null, msgs);
if (newStart != null)
msgs = ((InternalEObject)newStart).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.SCENARIO_PARAMETERS__START, null, msgs);
msgs = basicSetStart(newStart, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__START, newStart, newStart));
}
/**
*
*
* @generated
*/
@Override
public Parameter getDuration() {
return duration;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetDuration(Parameter newDuration, NotificationChain msgs) {
Parameter oldDuration = duration;
duration = newDuration;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__DURATION, oldDuration, newDuration);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setDuration(Parameter newDuration) {
if (newDuration != duration) {
NotificationChain msgs = null;
if (duration != null)
msgs = ((InternalEObject)duration).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.SCENARIO_PARAMETERS__DURATION, null, msgs);
if (newDuration != null)
msgs = ((InternalEObject)newDuration).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.SCENARIO_PARAMETERS__DURATION, null, msgs);
msgs = basicSetDuration(newDuration, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__DURATION, newDuration, newDuration));
}
/**
*
*
* @generated
*/
@Override
public PropertyParameters getPropertyParameters() {
return propertyParameters;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetPropertyParameters(PropertyParameters newPropertyParameters, NotificationChain msgs) {
PropertyParameters oldPropertyParameters = propertyParameters;
propertyParameters = newPropertyParameters;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__PROPERTY_PARAMETERS, oldPropertyParameters, newPropertyParameters);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setPropertyParameters(PropertyParameters newPropertyParameters) {
if (newPropertyParameters != propertyParameters) {
NotificationChain msgs = null;
if (propertyParameters != null)
msgs = ((InternalEObject)propertyParameters).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.SCENARIO_PARAMETERS__PROPERTY_PARAMETERS, null, msgs);
if (newPropertyParameters != null)
msgs = ((InternalEObject)newPropertyParameters).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.SCENARIO_PARAMETERS__PROPERTY_PARAMETERS, null, msgs);
msgs = basicSetPropertyParameters(newPropertyParameters, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__PROPERTY_PARAMETERS, newPropertyParameters, newPropertyParameters));
}
/**
*
*
* @generated
*/
@Override
public String getBaseCurrencyUnit() {
return baseCurrencyUnit;
}
/**
*
*
* @generated
*/
@Override
public void setBaseCurrencyUnit(String newBaseCurrencyUnit) {
String oldBaseCurrencyUnit = baseCurrencyUnit;
baseCurrencyUnit = newBaseCurrencyUnit;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__BASE_CURRENCY_UNIT, oldBaseCurrencyUnit, baseCurrencyUnit));
}
/**
*
*
* @generated
*/
@Override
public TimeUnit getBaseTimeUnit() {
return baseTimeUnit;
}
/**
*
*
* @generated
*/
@Override
public void setBaseTimeUnit(TimeUnit newBaseTimeUnit) {
TimeUnit oldBaseTimeUnit = baseTimeUnit;
baseTimeUnit = newBaseTimeUnit == null ? BASE_TIME_UNIT_EDEFAULT : newBaseTimeUnit;
boolean oldBaseTimeUnitESet = baseTimeUnitESet;
baseTimeUnitESet = true;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__BASE_TIME_UNIT, oldBaseTimeUnit, baseTimeUnit, !oldBaseTimeUnitESet));
}
/**
*
*
* @generated
*/
@Override
public void unsetBaseTimeUnit() {
TimeUnit oldBaseTimeUnit = baseTimeUnit;
boolean oldBaseTimeUnitESet = baseTimeUnitESet;
baseTimeUnit = BASE_TIME_UNIT_EDEFAULT;
baseTimeUnitESet = false;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.UNSET, BpsimPackage.SCENARIO_PARAMETERS__BASE_TIME_UNIT, oldBaseTimeUnit, BASE_TIME_UNIT_EDEFAULT, oldBaseTimeUnitESet));
}
/**
*
*
* @generated
*/
@Override
public boolean isSetBaseTimeUnit() {
return baseTimeUnitESet;
}
/**
*
*
* @generated
*/
@Override
public int getReplication() {
return replication;
}
/**
*
*
* @generated
*/
@Override
public void setReplication(int newReplication) {
int oldReplication = replication;
replication = newReplication;
boolean oldReplicationESet = replicationESet;
replicationESet = true;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__REPLICATION, oldReplication, replication, !oldReplicationESet));
}
/**
*
*
* @generated
*/
@Override
public void unsetReplication() {
int oldReplication = replication;
boolean oldReplicationESet = replicationESet;
replication = REPLICATION_EDEFAULT;
replicationESet = false;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.UNSET, BpsimPackage.SCENARIO_PARAMETERS__REPLICATION, oldReplication, REPLICATION_EDEFAULT, oldReplicationESet));
}
/**
*
*
* @generated
*/
@Override
public boolean isSetReplication() {
return replicationESet;
}
/**
*
*
* @generated
*/
@Override
public long getSeed() {
return seed;
}
/**
*
*
* @generated
*/
@Override
public void setSeed(long newSeed) {
long oldSeed = seed;
seed = newSeed;
boolean oldSeedESet = seedESet;
seedESet = true;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.SCENARIO_PARAMETERS__SEED, oldSeed, seed, !oldSeedESet));
}
/**
*
*
* @generated
*/
@Override
public void unsetSeed() {
long oldSeed = seed;
boolean oldSeedESet = seedESet;
seed = SEED_EDEFAULT;
seedESet = false;
if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.UNSET, BpsimPackage.SCENARIO_PARAMETERS__SEED, oldSeed, SEED_EDEFAULT, oldSeedESet));
}
/**
*
*
* @generated
*/
@Override
public boolean isSetSeed() {
return seedESet;
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs) {
switch (featureID) {
case BpsimPackage.SCENARIO_PARAMETERS__START:
return basicSetStart(null, msgs);
case BpsimPackage.SCENARIO_PARAMETERS__DURATION:
return basicSetDuration(null, msgs);
case BpsimPackage.SCENARIO_PARAMETERS__PROPERTY_PARAMETERS:
return basicSetPropertyParameters(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType) {
switch (featureID) {
case BpsimPackage.SCENARIO_PARAMETERS__START:
return getStart();
case BpsimPackage.SCENARIO_PARAMETERS__DURATION:
return getDuration();
case BpsimPackage.SCENARIO_PARAMETERS__PROPERTY_PARAMETERS:
return getPropertyParameters();
case BpsimPackage.SCENARIO_PARAMETERS__BASE_CURRENCY_UNIT:
return getBaseCurrencyUnit();
case BpsimPackage.SCENARIO_PARAMETERS__BASE_TIME_UNIT:
return getBaseTimeUnit();
case BpsimPackage.SCENARIO_PARAMETERS__REPLICATION:
return getReplication();
case BpsimPackage.SCENARIO_PARAMETERS__SEED:
return getSeed();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue) {
switch (featureID) {
case BpsimPackage.SCENARIO_PARAMETERS__START:
setStart((Parameter)newValue);
return;
case BpsimPackage.SCENARIO_PARAMETERS__DURATION:
setDuration((Parameter)newValue);
return;
case BpsimPackage.SCENARIO_PARAMETERS__PROPERTY_PARAMETERS:
setPropertyParameters((PropertyParameters)newValue);
return;
case BpsimPackage.SCENARIO_PARAMETERS__BASE_CURRENCY_UNIT:
setBaseCurrencyUnit((String)newValue);
return;
case BpsimPackage.SCENARIO_PARAMETERS__BASE_TIME_UNIT:
setBaseTimeUnit((TimeUnit)newValue);
return;
case BpsimPackage.SCENARIO_PARAMETERS__REPLICATION:
setReplication((Integer)newValue);
return;
case BpsimPackage.SCENARIO_PARAMETERS__SEED:
setSeed((Long)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID) {
switch (featureID) {
case BpsimPackage.SCENARIO_PARAMETERS__START:
setStart((Parameter)null);
return;
case BpsimPackage.SCENARIO_PARAMETERS__DURATION:
setDuration((Parameter)null);
return;
case BpsimPackage.SCENARIO_PARAMETERS__PROPERTY_PARAMETERS:
setPropertyParameters((PropertyParameters)null);
return;
case BpsimPackage.SCENARIO_PARAMETERS__BASE_CURRENCY_UNIT:
setBaseCurrencyUnit(BASE_CURRENCY_UNIT_EDEFAULT);
return;
case BpsimPackage.SCENARIO_PARAMETERS__BASE_TIME_UNIT:
unsetBaseTimeUnit();
return;
case BpsimPackage.SCENARIO_PARAMETERS__REPLICATION:
unsetReplication();
return;
case BpsimPackage.SCENARIO_PARAMETERS__SEED:
unsetSeed();
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID) {
switch (featureID) {
case BpsimPackage.SCENARIO_PARAMETERS__START:
return start != null;
case BpsimPackage.SCENARIO_PARAMETERS__DURATION:
return duration != null;
case BpsimPackage.SCENARIO_PARAMETERS__PROPERTY_PARAMETERS:
return propertyParameters != null;
case BpsimPackage.SCENARIO_PARAMETERS__BASE_CURRENCY_UNIT:
return BASE_CURRENCY_UNIT_EDEFAULT == null ? baseCurrencyUnit != null : !BASE_CURRENCY_UNIT_EDEFAULT.equals(baseCurrencyUnit);
case BpsimPackage.SCENARIO_PARAMETERS__BASE_TIME_UNIT:
return isSetBaseTimeUnit();
case BpsimPackage.SCENARIO_PARAMETERS__REPLICATION:
return isSetReplication();
case BpsimPackage.SCENARIO_PARAMETERS__SEED:
return isSetSeed();
}
return super.eIsSet(featureID);
}
/**
*
*
* @generated
*/
@Override
public String toString() {
if (eIsProxy()) return super.toString();
StringBuilder result = new StringBuilder(super.toString());
result.append(" (baseCurrencyUnit: ");
result.append(baseCurrencyUnit);
result.append(", baseTimeUnit: ");
if (baseTimeUnitESet) result.append(baseTimeUnit); else result.append("");
result.append(", replication: ");
if (replicationESet) result.append(replication); else result.append("");
result.append(", seed: ");
if (seedESet) result.append(seed); else result.append("");
result.append(')');
return result.toString();
}
} //ScenarioParametersImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy