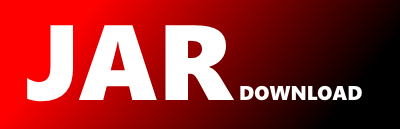
bpsim.impl.TimeParametersImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package bpsim.impl;
import bpsim.BpsimPackage;
import bpsim.Parameter;
import bpsim.TimeParameters;
import com.google.gwt.user.client.rpc.GwtTransient;
import org.eclipse.emf.common.notify.Notification;
import org.eclipse.emf.common.notify.NotificationChain;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.InternalEObject;
import org.eclipse.emf.ecore.impl.ENotificationImpl;
import org.eclipse.emf.ecore.impl.EObjectImpl;
/**
*
* An implementation of the model object 'Time Parameters'.
*
*
* The following features are implemented:
*
*
* - {@link bpsim.impl.TimeParametersImpl#getTransferTime Transfer Time}
* - {@link bpsim.impl.TimeParametersImpl#getQueueTime Queue Time}
* - {@link bpsim.impl.TimeParametersImpl#getWaitTime Wait Time}
* - {@link bpsim.impl.TimeParametersImpl#getSetUpTime Set Up Time}
* - {@link bpsim.impl.TimeParametersImpl#getProcessingTime Processing Time}
* - {@link bpsim.impl.TimeParametersImpl#getValidationTime Validation Time}
* - {@link bpsim.impl.TimeParametersImpl#getReworkTime Rework Time}
*
*
* @generated
*/
public class TimeParametersImpl extends EObjectImpl implements TimeParameters {
/**
* The cached value of the '{@link #getTransferTime() Transfer Time}' containment reference.
*
*
* @see #getTransferTime()
* @generated
* @ordered
*/
@GwtTransient
protected Parameter transferTime;
/**
* The cached value of the '{@link #getQueueTime() Queue Time}' containment reference.
*
*
* @see #getQueueTime()
* @generated
* @ordered
*/
@GwtTransient
protected Parameter queueTime;
/**
* The cached value of the '{@link #getWaitTime() Wait Time}' containment reference.
*
*
* @see #getWaitTime()
* @generated
* @ordered
*/
@GwtTransient
protected Parameter waitTime;
/**
* The cached value of the '{@link #getSetUpTime() Set Up Time}' containment reference.
*
*
* @see #getSetUpTime()
* @generated
* @ordered
*/
@GwtTransient
protected Parameter setUpTime;
/**
* The cached value of the '{@link #getProcessingTime() Processing Time}' containment reference.
*
*
* @see #getProcessingTime()
* @generated
* @ordered
*/
@GwtTransient
protected Parameter processingTime;
/**
* The cached value of the '{@link #getValidationTime() Validation Time}' containment reference.
*
*
* @see #getValidationTime()
* @generated
* @ordered
*/
@GwtTransient
protected Parameter validationTime;
/**
* The cached value of the '{@link #getReworkTime() Rework Time}' containment reference.
*
*
* @see #getReworkTime()
* @generated
* @ordered
*/
@GwtTransient
protected Parameter reworkTime;
/**
*
*
* @generated
*/
protected TimeParametersImpl() {
super();
}
/**
*
*
* @generated
*/
@Override
protected EClass eStaticClass() {
return BpsimPackage.Literals.TIME_PARAMETERS;
}
/**
*
*
* @generated
*/
@Override
public Parameter getTransferTime() {
return transferTime;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetTransferTime(Parameter newTransferTime, NotificationChain msgs) {
Parameter oldTransferTime = transferTime;
transferTime = newTransferTime;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__TRANSFER_TIME, oldTransferTime, newTransferTime);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setTransferTime(Parameter newTransferTime) {
if (newTransferTime != transferTime) {
NotificationChain msgs = null;
if (transferTime != null)
msgs = ((InternalEObject)transferTime).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__TRANSFER_TIME, null, msgs);
if (newTransferTime != null)
msgs = ((InternalEObject)newTransferTime).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__TRANSFER_TIME, null, msgs);
msgs = basicSetTransferTime(newTransferTime, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__TRANSFER_TIME, newTransferTime, newTransferTime));
}
/**
*
*
* @generated
*/
@Override
public Parameter getQueueTime() {
return queueTime;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetQueueTime(Parameter newQueueTime, NotificationChain msgs) {
Parameter oldQueueTime = queueTime;
queueTime = newQueueTime;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__QUEUE_TIME, oldQueueTime, newQueueTime);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setQueueTime(Parameter newQueueTime) {
if (newQueueTime != queueTime) {
NotificationChain msgs = null;
if (queueTime != null)
msgs = ((InternalEObject)queueTime).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__QUEUE_TIME, null, msgs);
if (newQueueTime != null)
msgs = ((InternalEObject)newQueueTime).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__QUEUE_TIME, null, msgs);
msgs = basicSetQueueTime(newQueueTime, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__QUEUE_TIME, newQueueTime, newQueueTime));
}
/**
*
*
* @generated
*/
@Override
public Parameter getWaitTime() {
return waitTime;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetWaitTime(Parameter newWaitTime, NotificationChain msgs) {
Parameter oldWaitTime = waitTime;
waitTime = newWaitTime;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__WAIT_TIME, oldWaitTime, newWaitTime);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setWaitTime(Parameter newWaitTime) {
if (newWaitTime != waitTime) {
NotificationChain msgs = null;
if (waitTime != null)
msgs = ((InternalEObject)waitTime).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__WAIT_TIME, null, msgs);
if (newWaitTime != null)
msgs = ((InternalEObject)newWaitTime).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__WAIT_TIME, null, msgs);
msgs = basicSetWaitTime(newWaitTime, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__WAIT_TIME, newWaitTime, newWaitTime));
}
/**
*
*
* @generated
*/
@Override
public Parameter getSetUpTime() {
return setUpTime;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetSetUpTime(Parameter newSetUpTime, NotificationChain msgs) {
Parameter oldSetUpTime = setUpTime;
setUpTime = newSetUpTime;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__SET_UP_TIME, oldSetUpTime, newSetUpTime);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setSetUpTime(Parameter newSetUpTime) {
if (newSetUpTime != setUpTime) {
NotificationChain msgs = null;
if (setUpTime != null)
msgs = ((InternalEObject)setUpTime).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__SET_UP_TIME, null, msgs);
if (newSetUpTime != null)
msgs = ((InternalEObject)newSetUpTime).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__SET_UP_TIME, null, msgs);
msgs = basicSetSetUpTime(newSetUpTime, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__SET_UP_TIME, newSetUpTime, newSetUpTime));
}
/**
*
*
* @generated
*/
@Override
public Parameter getProcessingTime() {
return processingTime;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetProcessingTime(Parameter newProcessingTime, NotificationChain msgs) {
Parameter oldProcessingTime = processingTime;
processingTime = newProcessingTime;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__PROCESSING_TIME, oldProcessingTime, newProcessingTime);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setProcessingTime(Parameter newProcessingTime) {
if (newProcessingTime != processingTime) {
NotificationChain msgs = null;
if (processingTime != null)
msgs = ((InternalEObject)processingTime).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__PROCESSING_TIME, null, msgs);
if (newProcessingTime != null)
msgs = ((InternalEObject)newProcessingTime).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__PROCESSING_TIME, null, msgs);
msgs = basicSetProcessingTime(newProcessingTime, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__PROCESSING_TIME, newProcessingTime, newProcessingTime));
}
/**
*
*
* @generated
*/
@Override
public Parameter getValidationTime() {
return validationTime;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetValidationTime(Parameter newValidationTime, NotificationChain msgs) {
Parameter oldValidationTime = validationTime;
validationTime = newValidationTime;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__VALIDATION_TIME, oldValidationTime, newValidationTime);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setValidationTime(Parameter newValidationTime) {
if (newValidationTime != validationTime) {
NotificationChain msgs = null;
if (validationTime != null)
msgs = ((InternalEObject)validationTime).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__VALIDATION_TIME, null, msgs);
if (newValidationTime != null)
msgs = ((InternalEObject)newValidationTime).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__VALIDATION_TIME, null, msgs);
msgs = basicSetValidationTime(newValidationTime, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__VALIDATION_TIME, newValidationTime, newValidationTime));
}
/**
*
*
* @generated
*/
@Override
public Parameter getReworkTime() {
return reworkTime;
}
/**
*
*
* @generated
*/
public NotificationChain basicSetReworkTime(Parameter newReworkTime, NotificationChain msgs) {
Parameter oldReworkTime = reworkTime;
reworkTime = newReworkTime;
if (eNotificationRequired()) {
ENotificationImpl notification = new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__REWORK_TIME, oldReworkTime, newReworkTime);
if (msgs == null) msgs = notification; else msgs.add(notification);
}
return msgs;
}
/**
*
*
* @generated
*/
@Override
public void setReworkTime(Parameter newReworkTime) {
if (newReworkTime != reworkTime) {
NotificationChain msgs = null;
if (reworkTime != null)
msgs = ((InternalEObject)reworkTime).eInverseRemove(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__REWORK_TIME, null, msgs);
if (newReworkTime != null)
msgs = ((InternalEObject)newReworkTime).eInverseAdd(this, EOPPOSITE_FEATURE_BASE - BpsimPackage.TIME_PARAMETERS__REWORK_TIME, null, msgs);
msgs = basicSetReworkTime(newReworkTime, msgs);
if (msgs != null) msgs.dispatch();
}
else if (eNotificationRequired())
eNotify(new ENotificationImpl(this, Notification.SET, BpsimPackage.TIME_PARAMETERS__REWORK_TIME, newReworkTime, newReworkTime));
}
/**
*
*
* @generated
*/
@Override
public NotificationChain eInverseRemove(InternalEObject otherEnd, int featureID, NotificationChain msgs) {
switch (featureID) {
case BpsimPackage.TIME_PARAMETERS__TRANSFER_TIME:
return basicSetTransferTime(null, msgs);
case BpsimPackage.TIME_PARAMETERS__QUEUE_TIME:
return basicSetQueueTime(null, msgs);
case BpsimPackage.TIME_PARAMETERS__WAIT_TIME:
return basicSetWaitTime(null, msgs);
case BpsimPackage.TIME_PARAMETERS__SET_UP_TIME:
return basicSetSetUpTime(null, msgs);
case BpsimPackage.TIME_PARAMETERS__PROCESSING_TIME:
return basicSetProcessingTime(null, msgs);
case BpsimPackage.TIME_PARAMETERS__VALIDATION_TIME:
return basicSetValidationTime(null, msgs);
case BpsimPackage.TIME_PARAMETERS__REWORK_TIME:
return basicSetReworkTime(null, msgs);
}
return super.eInverseRemove(otherEnd, featureID, msgs);
}
/**
*
*
* @generated
*/
@Override
public Object eGet(int featureID, boolean resolve, boolean coreType) {
switch (featureID) {
case BpsimPackage.TIME_PARAMETERS__TRANSFER_TIME:
return getTransferTime();
case BpsimPackage.TIME_PARAMETERS__QUEUE_TIME:
return getQueueTime();
case BpsimPackage.TIME_PARAMETERS__WAIT_TIME:
return getWaitTime();
case BpsimPackage.TIME_PARAMETERS__SET_UP_TIME:
return getSetUpTime();
case BpsimPackage.TIME_PARAMETERS__PROCESSING_TIME:
return getProcessingTime();
case BpsimPackage.TIME_PARAMETERS__VALIDATION_TIME:
return getValidationTime();
case BpsimPackage.TIME_PARAMETERS__REWORK_TIME:
return getReworkTime();
}
return super.eGet(featureID, resolve, coreType);
}
/**
*
*
* @generated
*/
@Override
public void eSet(int featureID, Object newValue) {
switch (featureID) {
case BpsimPackage.TIME_PARAMETERS__TRANSFER_TIME:
setTransferTime((Parameter)newValue);
return;
case BpsimPackage.TIME_PARAMETERS__QUEUE_TIME:
setQueueTime((Parameter)newValue);
return;
case BpsimPackage.TIME_PARAMETERS__WAIT_TIME:
setWaitTime((Parameter)newValue);
return;
case BpsimPackage.TIME_PARAMETERS__SET_UP_TIME:
setSetUpTime((Parameter)newValue);
return;
case BpsimPackage.TIME_PARAMETERS__PROCESSING_TIME:
setProcessingTime((Parameter)newValue);
return;
case BpsimPackage.TIME_PARAMETERS__VALIDATION_TIME:
setValidationTime((Parameter)newValue);
return;
case BpsimPackage.TIME_PARAMETERS__REWORK_TIME:
setReworkTime((Parameter)newValue);
return;
}
super.eSet(featureID, newValue);
}
/**
*
*
* @generated
*/
@Override
public void eUnset(int featureID) {
switch (featureID) {
case BpsimPackage.TIME_PARAMETERS__TRANSFER_TIME:
setTransferTime((Parameter)null);
return;
case BpsimPackage.TIME_PARAMETERS__QUEUE_TIME:
setQueueTime((Parameter)null);
return;
case BpsimPackage.TIME_PARAMETERS__WAIT_TIME:
setWaitTime((Parameter)null);
return;
case BpsimPackage.TIME_PARAMETERS__SET_UP_TIME:
setSetUpTime((Parameter)null);
return;
case BpsimPackage.TIME_PARAMETERS__PROCESSING_TIME:
setProcessingTime((Parameter)null);
return;
case BpsimPackage.TIME_PARAMETERS__VALIDATION_TIME:
setValidationTime((Parameter)null);
return;
case BpsimPackage.TIME_PARAMETERS__REWORK_TIME:
setReworkTime((Parameter)null);
return;
}
super.eUnset(featureID);
}
/**
*
*
* @generated
*/
@Override
public boolean eIsSet(int featureID) {
switch (featureID) {
case BpsimPackage.TIME_PARAMETERS__TRANSFER_TIME:
return transferTime != null;
case BpsimPackage.TIME_PARAMETERS__QUEUE_TIME:
return queueTime != null;
case BpsimPackage.TIME_PARAMETERS__WAIT_TIME:
return waitTime != null;
case BpsimPackage.TIME_PARAMETERS__SET_UP_TIME:
return setUpTime != null;
case BpsimPackage.TIME_PARAMETERS__PROCESSING_TIME:
return processingTime != null;
case BpsimPackage.TIME_PARAMETERS__VALIDATION_TIME:
return validationTime != null;
case BpsimPackage.TIME_PARAMETERS__REWORK_TIME:
return reworkTime != null;
}
return super.eIsSet(featureID);
}
} //TimeParametersImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy