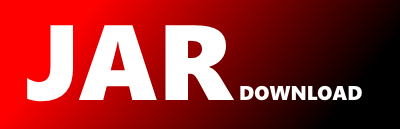
bpsim.util.BpsimAdapterFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package bpsim.util;
import bpsim.*;
import org.eclipse.emf.common.notify.Adapter;
import org.eclipse.emf.common.notify.Notifier;
import org.eclipse.emf.common.notify.impl.AdapterFactoryImpl;
import org.eclipse.emf.ecore.EObject;
/**
*
* The Adapter Factory for the model.
* It provides an adapter createXXX
method for each class of the model.
*
* @see bpsim.BpsimPackage
* @generated
*/
public class BpsimAdapterFactory extends AdapterFactoryImpl {
/**
* The cached model package.
*
*
* @generated
*/
protected static BpsimPackage modelPackage;
/**
* Creates an instance of the adapter factory.
*
*
* @generated
*/
public BpsimAdapterFactory() {
if (modelPackage == null) {
modelPackage = BpsimPackage.eINSTANCE;
}
}
/**
* Returns whether this factory is applicable for the type of the object.
*
* This implementation returns true
if the object is either the model's package or is an instance object of the model.
*
* @return whether this factory is applicable for the type of the object.
* @generated
*/
@Override
public boolean isFactoryForType(Object object) {
if (object == modelPackage) {
return true;
}
if (object instanceof EObject) {
return ((EObject)object).eClass().getEPackage() == modelPackage;
}
return false;
}
/**
* The switch that delegates to the createXXX
methods.
*
*
* @generated
*/
protected BpsimSwitch modelSwitch =
new BpsimSwitch() {
@Override
public Adapter caseBetaDistributionType(BetaDistributionType object) {
return createBetaDistributionTypeAdapter();
}
@Override
public Adapter caseBinomialDistributionType(BinomialDistributionType object) {
return createBinomialDistributionTypeAdapter();
}
@Override
public Adapter caseBooleanParameterType(BooleanParameterType object) {
return createBooleanParameterTypeAdapter();
}
@Override
public Adapter caseBPSimDataType(BPSimDataType object) {
return createBPSimDataTypeAdapter();
}
@Override
public Adapter caseCalendar(Calendar object) {
return createCalendarAdapter();
}
@Override
public Adapter caseConstantParameter(ConstantParameter object) {
return createConstantParameterAdapter();
}
@Override
public Adapter caseControlParameters(ControlParameters object) {
return createControlParametersAdapter();
}
@Override
public Adapter caseCostParameters(CostParameters object) {
return createCostParametersAdapter();
}
@Override
public Adapter caseDateTimeParameterType(DateTimeParameterType object) {
return createDateTimeParameterTypeAdapter();
}
@Override
public Adapter caseDistributionParameter(DistributionParameter object) {
return createDistributionParameterAdapter();
}
@Override
public Adapter caseDocumentRoot(DocumentRoot object) {
return createDocumentRootAdapter();
}
@Override
public Adapter caseDurationParameterType(DurationParameterType object) {
return createDurationParameterTypeAdapter();
}
@Override
public Adapter caseElementParameters(ElementParameters object) {
return createElementParametersAdapter();
}
@Override
public Adapter caseElementParametersType(ElementParametersType object) {
return createElementParametersTypeAdapter();
}
@Override
public Adapter caseEnumParameterType(EnumParameterType object) {
return createEnumParameterTypeAdapter();
}
@Override
public Adapter caseErlangDistributionType(ErlangDistributionType object) {
return createErlangDistributionTypeAdapter();
}
@Override
public Adapter caseExpressionParameterType(ExpressionParameterType object) {
return createExpressionParameterTypeAdapter();
}
@Override
public Adapter caseFloatingParameterType(FloatingParameterType object) {
return createFloatingParameterTypeAdapter();
}
@Override
public Adapter caseGammaDistributionType(GammaDistributionType object) {
return createGammaDistributionTypeAdapter();
}
@Override
public Adapter caseLogNormalDistributionType(LogNormalDistributionType object) {
return createLogNormalDistributionTypeAdapter();
}
@Override
public Adapter caseNegativeExponentialDistributionType(NegativeExponentialDistributionType object) {
return createNegativeExponentialDistributionTypeAdapter();
}
@Override
public Adapter caseNormalDistributionType(NormalDistributionType object) {
return createNormalDistributionTypeAdapter();
}
@Override
public Adapter caseNumericParameterType(NumericParameterType object) {
return createNumericParameterTypeAdapter();
}
@Override
public Adapter caseParameter(Parameter object) {
return createParameterAdapter();
}
@Override
public Adapter caseParameterValue(ParameterValue object) {
return createParameterValueAdapter();
}
@Override
public Adapter casePoissonDistributionType(PoissonDistributionType object) {
return createPoissonDistributionTypeAdapter();
}
@Override
public Adapter casePriorityParameters(PriorityParameters object) {
return createPriorityParametersAdapter();
}
@Override
public Adapter casePropertyParameters(PropertyParameters object) {
return createPropertyParametersAdapter();
}
@Override
public Adapter casePropertyType(PropertyType object) {
return createPropertyTypeAdapter();
}
@Override
public Adapter caseResourceParameters(ResourceParameters object) {
return createResourceParametersAdapter();
}
@Override
public Adapter caseScenario(Scenario object) {
return createScenarioAdapter();
}
@Override
public Adapter caseScenarioParameters(ScenarioParameters object) {
return createScenarioParametersAdapter();
}
@Override
public Adapter caseScenarioParametersType(ScenarioParametersType object) {
return createScenarioParametersTypeAdapter();
}
@Override
public Adapter caseStringParameterType(StringParameterType object) {
return createStringParameterTypeAdapter();
}
@Override
public Adapter caseTimeParameters(TimeParameters object) {
return createTimeParametersAdapter();
}
@Override
public Adapter caseTriangularDistributionType(TriangularDistributionType object) {
return createTriangularDistributionTypeAdapter();
}
@Override
public Adapter caseTruncatedNormalDistributionType(TruncatedNormalDistributionType object) {
return createTruncatedNormalDistributionTypeAdapter();
}
@Override
public Adapter caseUniformDistributionType(UniformDistributionType object) {
return createUniformDistributionTypeAdapter();
}
@Override
public Adapter caseUserDistributionDataPointType(UserDistributionDataPointType object) {
return createUserDistributionDataPointTypeAdapter();
}
@Override
public Adapter caseUserDistributionType(UserDistributionType object) {
return createUserDistributionTypeAdapter();
}
@Override
public Adapter caseVendorExtension(VendorExtension object) {
return createVendorExtensionAdapter();
}
@Override
public Adapter caseWeibullDistributionType(WeibullDistributionType object) {
return createWeibullDistributionTypeAdapter();
}
@Override
public Adapter defaultCase(EObject object) {
return createEObjectAdapter();
}
};
/**
* Creates an adapter for the target
.
*
*
* @param target the object to adapt.
* @return the adapter for the target
.
* @generated
*/
@Override
public Adapter createAdapter(Notifier target) {
return modelSwitch.doSwitch((EObject)target);
}
/**
* Creates a new adapter for an object of class '{@link bpsim.BetaDistributionType Beta Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.BetaDistributionType
* @generated
*/
public Adapter createBetaDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.BinomialDistributionType Binomial Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.BinomialDistributionType
* @generated
*/
public Adapter createBinomialDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.BooleanParameterType Boolean Parameter Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.BooleanParameterType
* @generated
*/
public Adapter createBooleanParameterTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.BPSimDataType BP Sim Data Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.BPSimDataType
* @generated
*/
public Adapter createBPSimDataTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.Calendar Calendar}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.Calendar
* @generated
*/
public Adapter createCalendarAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ConstantParameter Constant Parameter}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ConstantParameter
* @generated
*/
public Adapter createConstantParameterAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ControlParameters Control Parameters}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ControlParameters
* @generated
*/
public Adapter createControlParametersAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.CostParameters Cost Parameters}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.CostParameters
* @generated
*/
public Adapter createCostParametersAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.DateTimeParameterType Date Time Parameter Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.DateTimeParameterType
* @generated
*/
public Adapter createDateTimeParameterTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.DistributionParameter Distribution Parameter}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.DistributionParameter
* @generated
*/
public Adapter createDistributionParameterAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.DocumentRoot Document Root}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.DocumentRoot
* @generated
*/
public Adapter createDocumentRootAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.DurationParameterType Duration Parameter Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.DurationParameterType
* @generated
*/
public Adapter createDurationParameterTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ElementParameters Element Parameters}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ElementParameters
* @generated
*/
public Adapter createElementParametersAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ElementParametersType Element Parameters Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ElementParametersType
* @generated
*/
public Adapter createElementParametersTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.EnumParameterType Enum Parameter Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.EnumParameterType
* @generated
*/
public Adapter createEnumParameterTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ErlangDistributionType Erlang Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ErlangDistributionType
* @generated
*/
public Adapter createErlangDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ExpressionParameterType Expression Parameter Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ExpressionParameterType
* @generated
*/
public Adapter createExpressionParameterTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.FloatingParameterType Floating Parameter Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.FloatingParameterType
* @generated
*/
public Adapter createFloatingParameterTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.GammaDistributionType Gamma Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.GammaDistributionType
* @generated
*/
public Adapter createGammaDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.LogNormalDistributionType Log Normal Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.LogNormalDistributionType
* @generated
*/
public Adapter createLogNormalDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.NegativeExponentialDistributionType Negative Exponential Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.NegativeExponentialDistributionType
* @generated
*/
public Adapter createNegativeExponentialDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.NormalDistributionType Normal Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.NormalDistributionType
* @generated
*/
public Adapter createNormalDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.NumericParameterType Numeric Parameter Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.NumericParameterType
* @generated
*/
public Adapter createNumericParameterTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.Parameter Parameter}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.Parameter
* @generated
*/
public Adapter createParameterAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ParameterValue Parameter Value}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ParameterValue
* @generated
*/
public Adapter createParameterValueAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.PoissonDistributionType Poisson Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.PoissonDistributionType
* @generated
*/
public Adapter createPoissonDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.PriorityParameters Priority Parameters}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.PriorityParameters
* @generated
*/
public Adapter createPriorityParametersAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.PropertyParameters Property Parameters}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.PropertyParameters
* @generated
*/
public Adapter createPropertyParametersAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.PropertyType Property Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.PropertyType
* @generated
*/
public Adapter createPropertyTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ResourceParameters Resource Parameters}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ResourceParameters
* @generated
*/
public Adapter createResourceParametersAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.Scenario Scenario}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.Scenario
* @generated
*/
public Adapter createScenarioAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ScenarioParameters Scenario Parameters}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ScenarioParameters
* @generated
*/
public Adapter createScenarioParametersAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.ScenarioParametersType Scenario Parameters Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.ScenarioParametersType
* @generated
*/
public Adapter createScenarioParametersTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.StringParameterType String Parameter Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.StringParameterType
* @generated
*/
public Adapter createStringParameterTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.TimeParameters Time Parameters}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.TimeParameters
* @generated
*/
public Adapter createTimeParametersAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.TriangularDistributionType Triangular Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.TriangularDistributionType
* @generated
*/
public Adapter createTriangularDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.TruncatedNormalDistributionType Truncated Normal Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.TruncatedNormalDistributionType
* @generated
*/
public Adapter createTruncatedNormalDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.UniformDistributionType Uniform Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.UniformDistributionType
* @generated
*/
public Adapter createUniformDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.UserDistributionDataPointType User Distribution Data Point Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.UserDistributionDataPointType
* @generated
*/
public Adapter createUserDistributionDataPointTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.UserDistributionType User Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.UserDistributionType
* @generated
*/
public Adapter createUserDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.VendorExtension Vendor Extension}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.VendorExtension
* @generated
*/
public Adapter createVendorExtensionAdapter() {
return null;
}
/**
* Creates a new adapter for an object of class '{@link bpsim.WeibullDistributionType Weibull Distribution Type}'.
*
* This default implementation returns null so that we can easily ignore cases;
* it's useful to ignore a case when inheritance will catch all the cases anyway.
*
* @return the new adapter.
* @see bpsim.WeibullDistributionType
* @generated
*/
public Adapter createWeibullDistributionTypeAdapter() {
return null;
}
/**
* Creates a new adapter for the default case.
*
* This default implementation returns null.
*
* @return the new adapter.
* @generated
*/
public Adapter createEObjectAdapter() {
return null;
}
} //BpsimAdapterFactory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy