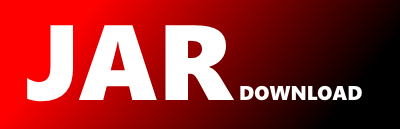
org.eclipse.bpmn2.Activity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*
*
* Copyright (c) 2010 SAP AG.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Reiner Hille-Doering (SAP AG) - initial API and implementation and/or initial documentation
*
*
*/
package org.eclipse.bpmn2;
import org.eclipse.emf.common.util.EList;
/**
*
* A representation of the model object 'Activity'.
*
*
*
* The following features are supported:
*
*
* - {@link org.eclipse.bpmn2.Activity#getIoSpecification Io Specification}
* - {@link org.eclipse.bpmn2.Activity#getBoundaryEventRefs Boundary Event Refs}
* - {@link org.eclipse.bpmn2.Activity#getProperties Properties}
* - {@link org.eclipse.bpmn2.Activity#getDataInputAssociations Data Input Associations}
* - {@link org.eclipse.bpmn2.Activity#getDataOutputAssociations Data Output Associations}
* - {@link org.eclipse.bpmn2.Activity#getResources Resources}
* - {@link org.eclipse.bpmn2.Activity#getLoopCharacteristics Loop Characteristics}
* - {@link org.eclipse.bpmn2.Activity#getCompletionQuantity Completion Quantity}
* - {@link org.eclipse.bpmn2.Activity#getDefault Default}
* - {@link org.eclipse.bpmn2.Activity#isIsForCompensation Is For Compensation}
* - {@link org.eclipse.bpmn2.Activity#getStartQuantity Start Quantity}
*
*
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity()
* @model extendedMetaData="name='tActivity' kind='elementOnly' abstract='true'"
* @generated
*/
public interface Activity extends FlowNode {
/**
* Returns the value of the 'Io Specification' containment reference.
*
*
* @return the value of the 'Io Specification' containment reference.
* @see #setIoSpecification(InputOutputSpecification)
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_IoSpecification()
* @model containment="true" ordered="false"
* extendedMetaData="kind='element' name='ioSpecification' namespace='http://www.omg.org/spec/BPMN/20100524/MODEL'"
* @generated
*/
InputOutputSpecification getIoSpecification();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.Activity#getIoSpecification Io Specification}' containment reference.
*
*
* @param value the new value of the 'Io Specification' containment reference.
* @see #getIoSpecification()
* @generated
*/
void setIoSpecification(InputOutputSpecification value);
/**
* Returns the value of the 'Boundary Event Refs' reference list.
* The list contents are of type {@link org.eclipse.bpmn2.BoundaryEvent}.
* It is bidirectional and its opposite is '{@link org.eclipse.bpmn2.BoundaryEvent#getAttachedToRef Attached To Ref}'.
*
*
* @return the value of the 'Boundary Event Refs' reference list.
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_BoundaryEventRefs()
* @see org.eclipse.bpmn2.BoundaryEvent#getAttachedToRef
* @model opposite="attachedToRef" resolveProxies="false" transient="true" derived="true" ordered="false"
* @generated
*/
EList getBoundaryEventRefs();
/**
* Returns the value of the 'Properties' containment reference list.
* The list contents are of type {@link org.eclipse.bpmn2.Property}.
*
*
* @return the value of the 'Properties' containment reference list.
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_Properties()
* @model containment="true" ordered="false"
* extendedMetaData="kind='element' name='property' namespace='http://www.omg.org/spec/BPMN/20100524/MODEL'"
* @generated
*/
EList getProperties();
/**
* Returns the value of the 'Data Input Associations' containment reference list.
* The list contents are of type {@link org.eclipse.bpmn2.DataInputAssociation}.
*
*
* @return the value of the 'Data Input Associations' containment reference list.
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_DataInputAssociations()
* @model containment="true" ordered="false"
* extendedMetaData="kind='element' name='dataInputAssociation' namespace='http://www.omg.org/spec/BPMN/20100524/MODEL'"
* @generated
*/
EList getDataInputAssociations();
/**
* Returns the value of the 'Data Output Associations' containment reference list.
* The list contents are of type {@link org.eclipse.bpmn2.DataOutputAssociation}.
*
*
* @return the value of the 'Data Output Associations' containment reference list.
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_DataOutputAssociations()
* @model containment="true" ordered="false"
* extendedMetaData="kind='element' name='dataOutputAssociation' namespace='http://www.omg.org/spec/BPMN/20100524/MODEL'"
* @generated
*/
EList getDataOutputAssociations();
/**
* Returns the value of the 'Resources' containment reference list.
* The list contents are of type {@link org.eclipse.bpmn2.ResourceRole}.
*
*
* @return the value of the 'Resources' containment reference list.
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_Resources()
* @model containment="true" ordered="false"
* extendedMetaData="kind='element' name='resourceRole' namespace='http://www.omg.org/spec/BPMN/20100524/MODEL' group='http://www.omg.org/spec/BPMN/20100524/MODEL#resourceRole'"
* @generated
*/
EList getResources();
/**
* Returns the value of the 'Loop Characteristics' containment reference.
*
*
* @return the value of the 'Loop Characteristics' containment reference.
* @see #setLoopCharacteristics(LoopCharacteristics)
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_LoopCharacteristics()
* @model containment="true" ordered="false"
* extendedMetaData="kind='element' name='loopCharacteristics' namespace='http://www.omg.org/spec/BPMN/20100524/MODEL' group='http://www.omg.org/spec/BPMN/20100524/MODEL#loopCharacteristics'"
* @generated
*/
LoopCharacteristics getLoopCharacteristics();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.Activity#getLoopCharacteristics Loop Characteristics}' containment reference.
*
*
* @param value the new value of the 'Loop Characteristics' containment reference.
* @see #getLoopCharacteristics()
* @generated
*/
void setLoopCharacteristics(LoopCharacteristics value);
/**
* Returns the value of the 'Completion Quantity' attribute.
* The default value is "1"
.
*
*
* @return the value of the 'Completion Quantity' attribute.
* @see #setCompletionQuantity(int)
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_CompletionQuantity()
* @model default="1" required="true" ordered="false"
* extendedMetaData="kind='attribute' name='completionQuantity'"
* @generated
*/
int getCompletionQuantity();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.Activity#getCompletionQuantity Completion Quantity}' attribute.
*
*
* @param value the new value of the 'Completion Quantity' attribute.
* @see #getCompletionQuantity()
* @generated
*/
void setCompletionQuantity(int value);
/**
* Returns the value of the 'Default' reference.
*
*
* @return the value of the 'Default' reference.
* @see #setDefault(SequenceFlow)
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_Default()
* @model resolveProxies="false" ordered="false"
* extendedMetaData="kind='attribute' name='default'"
* @generated
*/
SequenceFlow getDefault();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.Activity#getDefault Default}' reference.
*
*
* @param value the new value of the 'Default' reference.
* @see #getDefault()
* @generated
*/
void setDefault(SequenceFlow value);
/**
* Returns the value of the 'Is For Compensation' attribute.
* The default value is "false"
.
*
*
* @return the value of the 'Is For Compensation' attribute.
* @see #setIsForCompensation(boolean)
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_IsForCompensation()
* @model default="false" required="true" ordered="false"
* extendedMetaData="kind='attribute' name='isForCompensation'"
* @generated
*/
boolean isIsForCompensation();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.Activity#isIsForCompensation Is For Compensation}' attribute.
*
*
* @param value the new value of the 'Is For Compensation' attribute.
* @see #isIsForCompensation()
* @generated
*/
void setIsForCompensation(boolean value);
/**
* Returns the value of the 'Start Quantity' attribute.
* The default value is "1"
.
*
*
* @return the value of the 'Start Quantity' attribute.
* @see #setStartQuantity(int)
* @see org.eclipse.bpmn2.Bpmn2Package#getActivity_StartQuantity()
* @model default="1" required="true" ordered="false"
* extendedMetaData="kind='attribute' name='startQuantity'"
* @generated
*/
int getStartQuantity();
/**
* Sets the value of the '{@link org.eclipse.bpmn2.Activity#getStartQuantity Start Quantity}' attribute.
*
*
* @param value the new value of the 'Start Quantity' attribute.
* @see #getStartQuantity()
* @generated
*/
void setStartQuantity(int value);
} // Activity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy