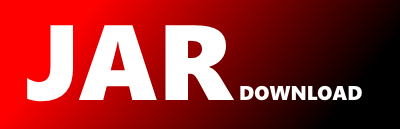
org.jboss.drools.DroolsPackage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Show all versions of kie-wb-common-stunner-bpmn-emf Show documentation
Kie Workbench - Common - Stunner - BPMN Definition Set - GWT Support for Eclipse EMF/XMI
/**
*/
package org.jboss.drools;
import org.eclipse.emf.ecore.EAttribute;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EDataType;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.EReference;
/**
*
* The Package for the model.
* It contains accessors for the meta objects to represent
*
* - each class,
* - each feature of each class,
* - each enum,
* - and each data type
*
*
* @see org.jboss.drools.DroolsFactory
* @model kind="package"
* @generated
*/
public interface DroolsPackage extends EPackage {
/**
* The package name.
*
*
* @generated
*/
String eNAME = "drools";
/**
* The package namespace URI.
*
*
* @generated
*/
String eNS_URI = "http://www.jboss.org/drools";
/**
* The package namespace name.
*
*
* @generated
*/
String eNS_PREFIX = "drools";
/**
* The singleton instance of the package.
*
*
* @generated
*/
DroolsPackage eINSTANCE = org.jboss.drools.impl.DroolsPackageImpl.init();
/**
* The meta object id for the '{@link org.jboss.drools.impl.DocumentRootImpl Document Root}' class.
*
*
* @see org.jboss.drools.impl.DocumentRootImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getDocumentRoot()
* @generated
*/
int DOCUMENT_ROOT = 0;
/**
* The feature id for the 'Mixed' attribute list.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__MIXED = 0;
/**
* The feature id for the 'XMLNS Prefix Map' map.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__XMLNS_PREFIX_MAP = 1;
/**
* The feature id for the 'XSI Schema Location' map.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__XSI_SCHEMA_LOCATION = 2;
/**
* The feature id for the 'Global' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__GLOBAL = 3;
/**
* The feature id for the 'Import' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__IMPORT = 4;
/**
* The feature id for the 'Meta Data' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__META_DATA = 5;
/**
* The feature id for the 'On Entry Script' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__ON_ENTRY_SCRIPT = 6;
/**
* The feature id for the 'On Exit Script' containment reference.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__ON_EXIT_SCRIPT = 7;
/**
* The feature id for the 'Package Name' attribute.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__PACKAGE_NAME = 8;
/**
* The feature id for the 'Priority' attribute.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__PRIORITY = 9;
/**
* The feature id for the 'Rule Flow Group' attribute.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__RULE_FLOW_GROUP = 10;
/**
* The feature id for the 'Task Name' attribute.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__TASK_NAME = 11;
/**
* The feature id for the 'Version' attribute.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT__VERSION = 12;
/**
* The number of structural features of the 'Document Root' class.
*
*
* @generated
* @ordered
*/
int DOCUMENT_ROOT_FEATURE_COUNT = 13;
/**
* The meta object id for the '{@link org.jboss.drools.impl.GlobalTypeImpl Global Type}' class.
*
*
* @see org.jboss.drools.impl.GlobalTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getGlobalType()
* @generated
*/
int GLOBAL_TYPE = 1;
/**
* The feature id for the 'Identifier' attribute.
*
*
* @generated
* @ordered
*/
int GLOBAL_TYPE__IDENTIFIER = 0;
/**
* The feature id for the 'Type' attribute.
*
*
* @generated
* @ordered
*/
int GLOBAL_TYPE__TYPE = 1;
/**
* The number of structural features of the 'Global Type' class.
*
*
* @generated
* @ordered
*/
int GLOBAL_TYPE_FEATURE_COUNT = 2;
/**
* The meta object id for the '{@link org.jboss.drools.impl.ImportTypeImpl Import Type}' class.
*
*
* @see org.jboss.drools.impl.ImportTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getImportType()
* @generated
*/
int IMPORT_TYPE = 2;
/**
* The feature id for the 'Name' attribute.
*
*
* @generated
* @ordered
*/
int IMPORT_TYPE__NAME = 0;
/**
* The number of structural features of the 'Import Type' class.
*
*
* @generated
* @ordered
*/
int IMPORT_TYPE_FEATURE_COUNT = 1;
/**
* The meta object id for the '{@link org.jboss.drools.impl.MetaDataTypeImpl Meta Data Type}' class.
*
*
* @see org.jboss.drools.impl.MetaDataTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getMetaDataType()
* @generated
*/
int META_DATA_TYPE = 3;
/**
* The feature id for the 'Meta Value' attribute.
*
*
* @generated
* @ordered
*/
int META_DATA_TYPE__META_VALUE = 0;
/**
* The feature id for the 'Name' attribute.
*
*
* @generated
* @ordered
*/
int META_DATA_TYPE__NAME = 1;
/**
* The number of structural features of the 'Meta Data Type' class.
*
*
* @generated
* @ordered
*/
int META_DATA_TYPE_FEATURE_COUNT = 2;
/**
* The meta object id for the '{@link org.jboss.drools.impl.OnEntryScriptTypeImpl On Entry Script Type}' class.
*
*
* @see org.jboss.drools.impl.OnEntryScriptTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getOnEntryScriptType()
* @generated
*/
int ON_ENTRY_SCRIPT_TYPE = 4;
/**
* The feature id for the 'Script' attribute.
*
*
* @generated
* @ordered
*/
int ON_ENTRY_SCRIPT_TYPE__SCRIPT = 0;
/**
* The feature id for the 'Script Format' attribute.
*
*
* @generated
* @ordered
*/
int ON_ENTRY_SCRIPT_TYPE__SCRIPT_FORMAT = 1;
/**
* The number of structural features of the 'On Entry Script Type' class.
*
*
* @generated
* @ordered
*/
int ON_ENTRY_SCRIPT_TYPE_FEATURE_COUNT = 2;
/**
* The meta object id for the '{@link org.jboss.drools.impl.OnExitScriptTypeImpl On Exit Script Type}' class.
*
*
* @see org.jboss.drools.impl.OnExitScriptTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getOnExitScriptType()
* @generated
*/
int ON_EXIT_SCRIPT_TYPE = 5;
/**
* The feature id for the 'Script' attribute.
*
*
* @generated
* @ordered
*/
int ON_EXIT_SCRIPT_TYPE__SCRIPT = 0;
/**
* The feature id for the 'Script Format' attribute.
*
*
* @generated
* @ordered
*/
int ON_EXIT_SCRIPT_TYPE__SCRIPT_FORMAT = 1;
/**
* The number of structural features of the 'On Exit Script Type' class.
*
*
* @generated
* @ordered
*/
int ON_EXIT_SCRIPT_TYPE_FEATURE_COUNT = 2;
/**
* The meta object id for the 'Package Name Type' data type.
*
*
* @see java.lang.String
* @see org.jboss.drools.impl.DroolsPackageImpl#getPackageNameType()
* @generated
*/
int PACKAGE_NAME_TYPE = 6;
/**
* The meta object id for the 'Priority Type' data type.
*
*
* @see java.math.BigInteger
* @see org.jboss.drools.impl.DroolsPackageImpl#getPriorityType()
* @generated
*/
int PRIORITY_TYPE = 7;
/**
* The meta object id for the 'Rule Flow Group Type' data type.
*
*
* @see java.lang.String
* @see org.jboss.drools.impl.DroolsPackageImpl#getRuleFlowGroupType()
* @generated
*/
int RULE_FLOW_GROUP_TYPE = 8;
/**
* The meta object id for the 'Task Name Type' data type.
*
*
* @see java.lang.String
* @see org.jboss.drools.impl.DroolsPackageImpl#getTaskNameType()
* @generated
*/
int TASK_NAME_TYPE = 9;
/**
* The meta object id for the 'Version Type' data type.
*
*
* @see java.lang.String
* @see org.jboss.drools.impl.DroolsPackageImpl#getVersionType()
* @generated
*/
int VERSION_TYPE = 10;
/**
* Returns the meta object for class '{@link org.jboss.drools.DocumentRoot Document Root}'.
*
*
* @return the meta object for class 'Document Root'.
* @see org.jboss.drools.DocumentRoot
* @generated
*/
EClass getDocumentRoot();
/**
* Returns the meta object for the attribute list '{@link org.jboss.drools.DocumentRoot#getMixed Mixed}'.
*
*
* @return the meta object for the attribute list 'Mixed'.
* @see org.jboss.drools.DocumentRoot#getMixed()
* @see #getDocumentRoot()
* @generated
*/
EAttribute getDocumentRoot_Mixed();
/**
* Returns the meta object for the map '{@link org.jboss.drools.DocumentRoot#getXMLNSPrefixMap XMLNS Prefix Map}'.
*
*
* @return the meta object for the map 'XMLNS Prefix Map'.
* @see org.jboss.drools.DocumentRoot#getXMLNSPrefixMap()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_XMLNSPrefixMap();
/**
* Returns the meta object for the map '{@link org.jboss.drools.DocumentRoot#getXSISchemaLocation XSI Schema Location}'.
*
*
* @return the meta object for the map 'XSI Schema Location'.
* @see org.jboss.drools.DocumentRoot#getXSISchemaLocation()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_XSISchemaLocation();
/**
* Returns the meta object for the containment reference '{@link org.jboss.drools.DocumentRoot#getGlobal Global}'.
*
*
* @return the meta object for the containment reference 'Global'.
* @see org.jboss.drools.DocumentRoot#getGlobal()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_Global();
/**
* Returns the meta object for the containment reference '{@link org.jboss.drools.DocumentRoot#getImport Import}'.
*
*
* @return the meta object for the containment reference 'Import'.
* @see org.jboss.drools.DocumentRoot#getImport()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_Import();
/**
* Returns the meta object for the containment reference '{@link org.jboss.drools.DocumentRoot#getMetaData Meta Data}'.
*
*
* @return the meta object for the containment reference 'Meta Data'.
* @see org.jboss.drools.DocumentRoot#getMetaData()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_MetaData();
/**
* Returns the meta object for the containment reference '{@link org.jboss.drools.DocumentRoot#getOnEntryScript On Entry Script}'.
*
*
* @return the meta object for the containment reference 'On Entry Script'.
* @see org.jboss.drools.DocumentRoot#getOnEntryScript()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_OnEntryScript();
/**
* Returns the meta object for the containment reference '{@link org.jboss.drools.DocumentRoot#getOnExitScript On Exit Script}'.
*
*
* @return the meta object for the containment reference 'On Exit Script'.
* @see org.jboss.drools.DocumentRoot#getOnExitScript()
* @see #getDocumentRoot()
* @generated
*/
EReference getDocumentRoot_OnExitScript();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.DocumentRoot#getPackageName Package Name}'.
*
*
* @return the meta object for the attribute 'Package Name'.
* @see org.jboss.drools.DocumentRoot#getPackageName()
* @see #getDocumentRoot()
* @generated
*/
EAttribute getDocumentRoot_PackageName();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.DocumentRoot#getPriority Priority}'.
*
*
* @return the meta object for the attribute 'Priority'.
* @see org.jboss.drools.DocumentRoot#getPriority()
* @see #getDocumentRoot()
* @generated
*/
EAttribute getDocumentRoot_Priority();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.DocumentRoot#getRuleFlowGroup Rule Flow Group}'.
*
*
* @return the meta object for the attribute 'Rule Flow Group'.
* @see org.jboss.drools.DocumentRoot#getRuleFlowGroup()
* @see #getDocumentRoot()
* @generated
*/
EAttribute getDocumentRoot_RuleFlowGroup();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.DocumentRoot#getTaskName Task Name}'.
*
*
* @return the meta object for the attribute 'Task Name'.
* @see org.jboss.drools.DocumentRoot#getTaskName()
* @see #getDocumentRoot()
* @generated
*/
EAttribute getDocumentRoot_TaskName();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.DocumentRoot#getVersion Version}'.
*
*
* @return the meta object for the attribute 'Version'.
* @see org.jboss.drools.DocumentRoot#getVersion()
* @see #getDocumentRoot()
* @generated
*/
EAttribute getDocumentRoot_Version();
/**
* Returns the meta object for class '{@link org.jboss.drools.GlobalType Global Type}'.
*
*
* @return the meta object for class 'Global Type'.
* @see org.jboss.drools.GlobalType
* @generated
*/
EClass getGlobalType();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.GlobalType#getIdentifier Identifier}'.
*
*
* @return the meta object for the attribute 'Identifier'.
* @see org.jboss.drools.GlobalType#getIdentifier()
* @see #getGlobalType()
* @generated
*/
EAttribute getGlobalType_Identifier();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.GlobalType#getType Type}'.
*
*
* @return the meta object for the attribute 'Type'.
* @see org.jboss.drools.GlobalType#getType()
* @see #getGlobalType()
* @generated
*/
EAttribute getGlobalType_Type();
/**
* Returns the meta object for class '{@link org.jboss.drools.ImportType Import Type}'.
*
*
* @return the meta object for class 'Import Type'.
* @see org.jboss.drools.ImportType
* @generated
*/
EClass getImportType();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.ImportType#getName Name}'.
*
*
* @return the meta object for the attribute 'Name'.
* @see org.jboss.drools.ImportType#getName()
* @see #getImportType()
* @generated
*/
EAttribute getImportType_Name();
/**
* Returns the meta object for class '{@link org.jboss.drools.MetaDataType Meta Data Type}'.
*
*
* @return the meta object for class 'Meta Data Type'.
* @see org.jboss.drools.MetaDataType
* @generated
*/
EClass getMetaDataType();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.MetaDataType#getMetaValue Meta Value}'.
*
*
* @return the meta object for the attribute 'Meta Value'.
* @see org.jboss.drools.MetaDataType#getMetaValue()
* @see #getMetaDataType()
* @generated
*/
EAttribute getMetaDataType_MetaValue();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.MetaDataType#getName Name}'.
*
*
* @return the meta object for the attribute 'Name'.
* @see org.jboss.drools.MetaDataType#getName()
* @see #getMetaDataType()
* @generated
*/
EAttribute getMetaDataType_Name();
/**
* Returns the meta object for class '{@link org.jboss.drools.OnEntryScriptType On Entry Script Type}'.
*
*
* @return the meta object for class 'On Entry Script Type'.
* @see org.jboss.drools.OnEntryScriptType
* @generated
*/
EClass getOnEntryScriptType();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.OnEntryScriptType#getScript Script}'.
*
*
* @return the meta object for the attribute 'Script'.
* @see org.jboss.drools.OnEntryScriptType#getScript()
* @see #getOnEntryScriptType()
* @generated
*/
EAttribute getOnEntryScriptType_Script();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.OnEntryScriptType#getScriptFormat Script Format}'.
*
*
* @return the meta object for the attribute 'Script Format'.
* @see org.jboss.drools.OnEntryScriptType#getScriptFormat()
* @see #getOnEntryScriptType()
* @generated
*/
EAttribute getOnEntryScriptType_ScriptFormat();
/**
* Returns the meta object for class '{@link org.jboss.drools.OnExitScriptType On Exit Script Type}'.
*
*
* @return the meta object for class 'On Exit Script Type'.
* @see org.jboss.drools.OnExitScriptType
* @generated
*/
EClass getOnExitScriptType();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.OnExitScriptType#getScript Script}'.
*
*
* @return the meta object for the attribute 'Script'.
* @see org.jboss.drools.OnExitScriptType#getScript()
* @see #getOnExitScriptType()
* @generated
*/
EAttribute getOnExitScriptType_Script();
/**
* Returns the meta object for the attribute '{@link org.jboss.drools.OnExitScriptType#getScriptFormat Script Format}'.
*
*
* @return the meta object for the attribute 'Script Format'.
* @see org.jboss.drools.OnExitScriptType#getScriptFormat()
* @see #getOnExitScriptType()
* @generated
*/
EAttribute getOnExitScriptType_ScriptFormat();
/**
* Returns the meta object for data type '{@link java.lang.String Package Name Type}'.
*
*
* @return the meta object for data type 'Package Name Type'.
* @see java.lang.String
* @model instanceClass="java.lang.String"
* extendedMetaData="name='packageName_._type' baseType='http://www.eclipse.org/emf/2003/XMLType#string'"
* @generated
*/
EDataType getPackageNameType();
/**
* Returns the meta object for data type '{@link java.math.BigInteger Priority Type}'.
*
*
* @return the meta object for data type 'Priority Type'.
* @see java.math.BigInteger
* @model instanceClass="java.math.BigInteger"
* extendedMetaData="name='priority_._type' baseType='http://www.eclipse.org/emf/2003/XMLType#integer' minInclusive='1'"
* @generated
*/
EDataType getPriorityType();
/**
* Returns the meta object for data type '{@link java.lang.String Rule Flow Group Type}'.
*
*
* @return the meta object for data type 'Rule Flow Group Type'.
* @see java.lang.String
* @model instanceClass="java.lang.String"
* extendedMetaData="name='ruleFlowGroup_._type' baseType='http://www.eclipse.org/emf/2003/XMLType#string'"
* @generated
*/
EDataType getRuleFlowGroupType();
/**
* Returns the meta object for data type '{@link java.lang.String Task Name Type}'.
*
*
* @return the meta object for data type 'Task Name Type'.
* @see java.lang.String
* @model instanceClass="java.lang.String"
* extendedMetaData="name='taskName_._type' baseType='http://www.eclipse.org/emf/2003/XMLType#string'"
* @generated
*/
EDataType getTaskNameType();
/**
* Returns the meta object for data type '{@link java.lang.String Version Type}'.
*
*
* @return the meta object for data type 'Version Type'.
* @see java.lang.String
* @model instanceClass="java.lang.String"
* extendedMetaData="name='version_._type' baseType='http://www.eclipse.org/emf/2003/XMLType#string'"
* @generated
*/
EDataType getVersionType();
/**
* Returns the factory that creates the instances of the model.
*
*
* @return the factory that creates the instances of the model.
* @generated
*/
DroolsFactory getDroolsFactory();
/**
*
* Defines literals for the meta objects that represent
*
* - each class,
* - each feature of each class,
* - each enum,
* - and each data type
*
*
* @generated
*/
interface Literals {
/**
* The meta object literal for the '{@link org.jboss.drools.impl.DocumentRootImpl Document Root}' class.
*
*
* @see org.jboss.drools.impl.DocumentRootImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getDocumentRoot()
* @generated
*/
EClass DOCUMENT_ROOT = eINSTANCE.getDocumentRoot();
/**
* The meta object literal for the 'Mixed' attribute list feature.
*
*
* @generated
*/
EAttribute DOCUMENT_ROOT__MIXED = eINSTANCE.getDocumentRoot_Mixed();
/**
* The meta object literal for the 'XMLNS Prefix Map' map feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__XMLNS_PREFIX_MAP = eINSTANCE.getDocumentRoot_XMLNSPrefixMap();
/**
* The meta object literal for the 'XSI Schema Location' map feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__XSI_SCHEMA_LOCATION = eINSTANCE.getDocumentRoot_XSISchemaLocation();
/**
* The meta object literal for the 'Global' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__GLOBAL = eINSTANCE.getDocumentRoot_Global();
/**
* The meta object literal for the 'Import' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__IMPORT = eINSTANCE.getDocumentRoot_Import();
/**
* The meta object literal for the 'Meta Data' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__META_DATA = eINSTANCE.getDocumentRoot_MetaData();
/**
* The meta object literal for the 'On Entry Script' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__ON_ENTRY_SCRIPT = eINSTANCE.getDocumentRoot_OnEntryScript();
/**
* The meta object literal for the 'On Exit Script' containment reference feature.
*
*
* @generated
*/
EReference DOCUMENT_ROOT__ON_EXIT_SCRIPT = eINSTANCE.getDocumentRoot_OnExitScript();
/**
* The meta object literal for the 'Package Name' attribute feature.
*
*
* @generated
*/
EAttribute DOCUMENT_ROOT__PACKAGE_NAME = eINSTANCE.getDocumentRoot_PackageName();
/**
* The meta object literal for the 'Priority' attribute feature.
*
*
* @generated
*/
EAttribute DOCUMENT_ROOT__PRIORITY = eINSTANCE.getDocumentRoot_Priority();
/**
* The meta object literal for the 'Rule Flow Group' attribute feature.
*
*
* @generated
*/
EAttribute DOCUMENT_ROOT__RULE_FLOW_GROUP = eINSTANCE.getDocumentRoot_RuleFlowGroup();
/**
* The meta object literal for the 'Task Name' attribute feature.
*
*
* @generated
*/
EAttribute DOCUMENT_ROOT__TASK_NAME = eINSTANCE.getDocumentRoot_TaskName();
/**
* The meta object literal for the 'Version' attribute feature.
*
*
* @generated
*/
EAttribute DOCUMENT_ROOT__VERSION = eINSTANCE.getDocumentRoot_Version();
/**
* The meta object literal for the '{@link org.jboss.drools.impl.GlobalTypeImpl Global Type}' class.
*
*
* @see org.jboss.drools.impl.GlobalTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getGlobalType()
* @generated
*/
EClass GLOBAL_TYPE = eINSTANCE.getGlobalType();
/**
* The meta object literal for the 'Identifier' attribute feature.
*
*
* @generated
*/
EAttribute GLOBAL_TYPE__IDENTIFIER = eINSTANCE.getGlobalType_Identifier();
/**
* The meta object literal for the 'Type' attribute feature.
*
*
* @generated
*/
EAttribute GLOBAL_TYPE__TYPE = eINSTANCE.getGlobalType_Type();
/**
* The meta object literal for the '{@link org.jboss.drools.impl.ImportTypeImpl Import Type}' class.
*
*
* @see org.jboss.drools.impl.ImportTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getImportType()
* @generated
*/
EClass IMPORT_TYPE = eINSTANCE.getImportType();
/**
* The meta object literal for the 'Name' attribute feature.
*
*
* @generated
*/
EAttribute IMPORT_TYPE__NAME = eINSTANCE.getImportType_Name();
/**
* The meta object literal for the '{@link org.jboss.drools.impl.MetaDataTypeImpl Meta Data Type}' class.
*
*
* @see org.jboss.drools.impl.MetaDataTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getMetaDataType()
* @generated
*/
EClass META_DATA_TYPE = eINSTANCE.getMetaDataType();
/**
* The meta object literal for the 'Meta Value' attribute feature.
*
*
* @generated
*/
EAttribute META_DATA_TYPE__META_VALUE = eINSTANCE.getMetaDataType_MetaValue();
/**
* The meta object literal for the 'Name' attribute feature.
*
*
* @generated
*/
EAttribute META_DATA_TYPE__NAME = eINSTANCE.getMetaDataType_Name();
/**
* The meta object literal for the '{@link org.jboss.drools.impl.OnEntryScriptTypeImpl On Entry Script Type}' class.
*
*
* @see org.jboss.drools.impl.OnEntryScriptTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getOnEntryScriptType()
* @generated
*/
EClass ON_ENTRY_SCRIPT_TYPE = eINSTANCE.getOnEntryScriptType();
/**
* The meta object literal for the 'Script' attribute feature.
*
*
* @generated
*/
EAttribute ON_ENTRY_SCRIPT_TYPE__SCRIPT = eINSTANCE.getOnEntryScriptType_Script();
/**
* The meta object literal for the 'Script Format' attribute feature.
*
*
* @generated
*/
EAttribute ON_ENTRY_SCRIPT_TYPE__SCRIPT_FORMAT = eINSTANCE.getOnEntryScriptType_ScriptFormat();
/**
* The meta object literal for the '{@link org.jboss.drools.impl.OnExitScriptTypeImpl On Exit Script Type}' class.
*
*
* @see org.jboss.drools.impl.OnExitScriptTypeImpl
* @see org.jboss.drools.impl.DroolsPackageImpl#getOnExitScriptType()
* @generated
*/
EClass ON_EXIT_SCRIPT_TYPE = eINSTANCE.getOnExitScriptType();
/**
* The meta object literal for the 'Script' attribute feature.
*
*
* @generated
*/
EAttribute ON_EXIT_SCRIPT_TYPE__SCRIPT = eINSTANCE.getOnExitScriptType_Script();
/**
* The meta object literal for the 'Script Format' attribute feature.
*
*
* @generated
*/
EAttribute ON_EXIT_SCRIPT_TYPE__SCRIPT_FORMAT = eINSTANCE.getOnExitScriptType_ScriptFormat();
/**
* The meta object literal for the 'Package Name Type' data type.
*
*
* @see java.lang.String
* @see org.jboss.drools.impl.DroolsPackageImpl#getPackageNameType()
* @generated
*/
EDataType PACKAGE_NAME_TYPE = eINSTANCE.getPackageNameType();
/**
* The meta object literal for the 'Priority Type' data type.
*
*
* @see java.math.BigInteger
* @see org.jboss.drools.impl.DroolsPackageImpl#getPriorityType()
* @generated
*/
EDataType PRIORITY_TYPE = eINSTANCE.getPriorityType();
/**
* The meta object literal for the 'Rule Flow Group Type' data type.
*
*
* @see java.lang.String
* @see org.jboss.drools.impl.DroolsPackageImpl#getRuleFlowGroupType()
* @generated
*/
EDataType RULE_FLOW_GROUP_TYPE = eINSTANCE.getRuleFlowGroupType();
/**
* The meta object literal for the 'Task Name Type' data type.
*
*
* @see java.lang.String
* @see org.jboss.drools.impl.DroolsPackageImpl#getTaskNameType()
* @generated
*/
EDataType TASK_NAME_TYPE = eINSTANCE.getTaskNameType();
/**
* The meta object literal for the 'Version Type' data type.
*
*
* @see java.lang.String
* @see org.jboss.drools.impl.DroolsPackageImpl#getVersionType()
* @generated
*/
EDataType VERSION_TYPE = eINSTANCE.getVersionType();
}
} //DroolsPackage
© 2015 - 2025 Weber Informatics LLC | Privacy Policy