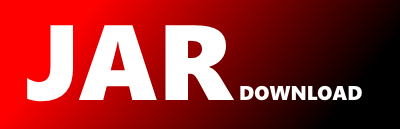
org.kie.internal.io.ResourceWithConfigurationImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-internal Show documentation
Show all versions of kie-internal Show documentation
The Drools and jBPM internal API which is NOT backwards compatible between releases.
package org.kie.internal.io;
import java.util.function.Consumer;
import org.kie.api.internal.assembler.KieAssemblerService;
import org.kie.api.io.Resource;
import org.kie.api.io.ResourceConfiguration;
import org.kie.api.io.ResourceType;
import org.kie.api.io.ResourceWithConfiguration;
public class ResourceWithConfigurationImpl implements ResourceWithConfiguration {
private final Resource resource;
private final ResourceConfiguration resourceConfiguration;
private final Consumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy