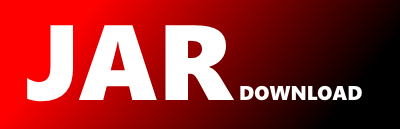
org.kie.pmml.commons.model.ProcessingDTO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-pmml-commons Show documentation
Show all versions of kie-pmml-commons Show documentation
Common code for PMML module
/*
* Copyright 2021 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kie.pmml.commons.model;
import java.util.ArrayList;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.kie.pmml.api.models.MiningField;
import org.kie.pmml.commons.model.tuples.KiePMMLNameValue;
import org.kie.pmml.commons.transformations.KiePMMLDefineFunction;
import org.kie.pmml.commons.transformations.KiePMMLDerivedField;
/**
* DTO class used to bring around data related to Pre/Post processing
* phases
*/
public class ProcessingDTO {
private final List defineFunctions;
private final List derivedFields;
private final List outputFields;
private final List kiePMMLTargets;
private final List kiePMMLNameValues;
private final List orderedReasonCodes;
private final List miningFields;
private Object predictedDisplayValue;
private Object entityId;
private Object affinity;
private Map probabilityMap;
/**
*
* @param model
* @param kiePMMLNameValues a mutable list of KiePMMLNameValue
*/
public ProcessingDTO(final KiePMMLModel model, final List kiePMMLNameValues) {
this.derivedFields = new ArrayList<>();
this.defineFunctions = new ArrayList<>();
if (model.getTransformationDictionary() != null) {
if (model.getTransformationDictionary().getDerivedFields() != null) {
this.derivedFields.addAll(model.getTransformationDictionary().getDerivedFields());
}
if (model.getTransformationDictionary().getDefineFunctions() != null) {
this.defineFunctions.addAll(model.getTransformationDictionary().getDefineFunctions());
}
}
if (model.getLocalTransformations() != null && model.getLocalTransformations().getDerivedFields() != null) {
this.derivedFields.addAll(model.getLocalTransformations().getDerivedFields());
}
this.outputFields = model.getKiePMMLOutputFields();
this.kiePMMLTargets = model.getKiePMMLTargets();
this.kiePMMLNameValues = kiePMMLNameValues;
this.orderedReasonCodes = new ArrayList<>();
this.miningFields = model.getMiningFields();
}
/**
* @param defineFunctions
* @param derivedFields
* @param outputFields
* @param kiePMMLTargets
* @param kiePMMLNameValues a mutable list of KiePMMLNameValue
* @param miningFields
* @param orderedReasonCodes a mutable list
*/
public ProcessingDTO(final List defineFunctions,
final List derivedFields,
final List outputFields,
final List kiePMMLTargets,
final List kiePMMLNameValues,
final List miningFields,
final List orderedReasonCodes) {
this.defineFunctions = defineFunctions;
this.derivedFields = derivedFields;
this.outputFields = outputFields;
this.kiePMMLTargets = kiePMMLTargets;
this.miningFields = miningFields;
this.kiePMMLNameValues = kiePMMLNameValues;
this.orderedReasonCodes = orderedReasonCodes;
this.predictedDisplayValue = null;
this.entityId = null;
this.affinity = null;
this.probabilityMap = new LinkedHashMap<>();
}
public List getDefineFunctions() {
return Collections.unmodifiableList(defineFunctions);
}
public List getDerivedFields() {
return Collections.unmodifiableList(derivedFields);
}
public List getOutputFields() {
return Collections.unmodifiableList(outputFields);
}
public List getKiePMMLTargets() {
return Collections.unmodifiableList(kiePMMLTargets);
}
public List getKiePMMLNameValues() {
return Collections.unmodifiableList(kiePMMLNameValues);
}
/**
* Add the given KiePMMLNameValue
to kiePMMLNameValues
* if there is not another with the same name; otherwise replace it.
*
* @param toAdd
* @return
*/
public boolean addKiePMMLNameValue(KiePMMLNameValue toAdd) {
kiePMMLNameValues.removeIf(kpm -> kpm.getName().equals(toAdd.getName()));
return kiePMMLNameValues.add(toAdd);
}
public List getOrderedReasonCodes() {
return Collections.unmodifiableList(orderedReasonCodes);
}
public boolean addOrderedReasonCodes(List toAdd) {
return orderedReasonCodes.addAll(toAdd);
}
public List getMiningFields() {
return Collections.unmodifiableList(miningFields);
}
public Object getPredictedDisplayValue() {
return predictedDisplayValue;
}
public void setPredictedDisplayValue(Object predictedDisplayValue) {
this.predictedDisplayValue = predictedDisplayValue;
}
public Object getEntityId() {
return entityId;
}
public void setEntityId(Object entityId) {
this.entityId = entityId;
}
public Object getAffinity() {
return affinity;
}
public void setAffinity(Object affinity) {
this.affinity = affinity;
}
public Map getProbabilityMap() {
return probabilityMap;
}
public void setProbabilityMap(Map probabilityMap) {
this.probabilityMap = probabilityMap;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy