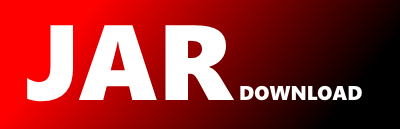
org.kie.pmml.commons.model.predicates.KiePMMLSimpleSetPredicate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kie-pmml-commons Show documentation
Show all versions of kie-pmml-commons Show documentation
Common code for PMML module
/*
* Copyright 2020 Red Hat, Inc. and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kie.pmml.commons.model.predicates;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.StringJoiner;
import org.kie.pmml.api.exceptions.KiePMMLException;
import org.kie.pmml.commons.model.KiePMMLExtension;
import org.kie.pmml.api.enums.ARRAY_TYPE;
import org.kie.pmml.api.enums.IN_NOTIN;
import org.kie.pmml.api.utils.ConverterTypeUtil;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* @see SimpleSetPredicate
*/
public class KiePMMLSimpleSetPredicate extends KiePMMLPredicate {
private static final Logger logger = LoggerFactory.getLogger(KiePMMLSimpleSetPredicate.class);
private final ARRAY_TYPE arrayType;
private final IN_NOTIN inNotIn;
protected List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy