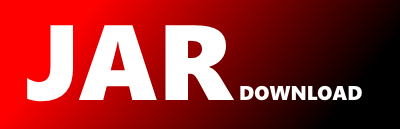
org.mariadb.jdbc.MySQLPooledConnection Maven / Gradle / Ivy
package org.mariadb.jdbc;
import javax.sql.*;
import java.sql.Statement;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.CopyOnWriteArrayList;
public class MySQLPooledConnection implements PooledConnection{
MySQLConnection connection;
List connectionEventListeners;
List statementEventListeners;
public MySQLPooledConnection(MySQLConnection connection)
{
this.connection = connection;
connection.pooledConnection = this;
statementEventListeners = new ArrayList();
connectionEventListeners = new ArrayList();
}
/**
* Creates and returns a Connection
object that is a handle
* for the physical connection that
* this PooledConnection
object represents.
* The connection pool manager calls this method when an application has
* called the method DataSource.getConnection
and there are
* no PooledConnection
objects available. See the
* {@link javax.sql.PooledConnection interface description} for more information.
*
* @return a Connection
object that is a handle to
* this PooledConnection
object
* @throws java.sql.SQLException if a database access error occurs
* if the JDBC driver does not support
* this method
* @since 1.4
*/
public Connection getConnection() throws SQLException {
return connection;
}
/**
* Closes the physical connection that this PooledConnection
* object represents. An application never calls this method directly;
* it is called by the connection pool module, or manager.
*
* See the {@link javax.sql.PooledConnection interface description} for more
* information.
*
* @throws java.sql.SQLException if a database access error occurs
* if the JDBC driver does not support
* this method
* @since 1.4
*/
public void close() throws SQLException {
connection.pooledConnection = null;
connection.close();
}
/**
* Registers the given event listener so that it will be notified
* when an event occurs on this PooledConnection
object.
*
* @param listener a component, usually the connection pool manager,
* that has implemented the
* ConnectionEventListener
interface and wants to be
* notified when the connection is closed or has an error
* @see #removeConnectionEventListener
*/
public void addConnectionEventListener(ConnectionEventListener listener) {
connectionEventListeners.add(listener);
}
/**
* Removes the given event listener from the list of components that
* will be notified when an event occurs on this
* PooledConnection
object.
*
* @param listener a component, usually the connection pool manager,
* that has implemented the
* ConnectionEventListener
interface and
* been registered with this PooledConnection
object as
* a listener
* @see #addConnectionEventListener
*/
public void removeConnectionEventListener(ConnectionEventListener listener) {
connectionEventListeners.remove(listener);
}
/**
* Registers a StatementEventListener
with this PooledConnection
object. Components that
* wish to be notified when PreparedStatement
s created by the
* connection are closed or are detected to be invalid may use this method
* to register a StatementEventListener
with this PooledConnection
object.
*
*
* @param listener an component which implements the StatementEventListener
* interface that is to be registered with this PooledConnection
object
*
* @since 1.6
*/
public void addStatementEventListener(StatementEventListener listener) {
statementEventListeners.add(listener);
}
/**
* Removes the specified StatementEventListener
from the list of
* components that will be notified when the driver detects that a
* PreparedStatement
has been closed or is invalid.
*
*
* @param listener the component which implements the
* StatementEventListener
interface that was previously
* registered with this PooledConnection
object
*
* @since 1.6
*/
public void removeStatementEventListener(StatementEventListener listener) {
statementEventListeners.remove(listener);
}
public void fireStatementClosed(Statement st) {
if (st instanceof PreparedStatement) {
StatementEvent event = new StatementEvent(this, (PreparedStatement)st);
for(StatementEventListener listener:statementEventListeners)
listener.statementClosed(event);
}
}
public void fireStatementErrorOccured(Statement st, SQLException e) {
if (st instanceof PreparedStatement) {
StatementEvent event = new StatementEvent(this,(PreparedStatement) st,e);
for(StatementEventListener listener:statementEventListeners)
listener.statementErrorOccurred(event);
}
}
public void fireConnectionClosed() {
ConnectionEvent event = new ConnectionEvent(this);
CopyOnWriteArrayList copyListeners = new CopyOnWriteArrayList(connectionEventListeners);
for(ConnectionEventListener listener: copyListeners)
listener.connectionClosed(event);
}
public void fireConnectionErrorOccured(SQLException e) {
ConnectionEvent event = new ConnectionEvent(this,e);
for(ConnectionEventListener listener: connectionEventListeners)
listener.connectionErrorOccurred(event);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy