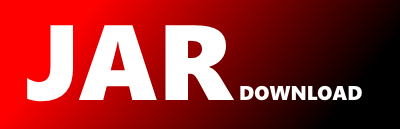
org.skife.jdbi.v2.ContainerFactoryRegistry Maven / Gradle / Ivy
/*
* Copyright (C) 2004 - 2014 Brian McCallister
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.skife.jdbi.v2;
import org.skife.jdbi.v2.tweak.ContainerFactory;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.SortedSet;
import java.util.TreeSet;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.CopyOnWriteArrayList;
class ContainerFactoryRegistry
{
private final Map, ContainerFactory>> cache = new ConcurrentHashMap, ContainerFactory>>();
private final List factories = new CopyOnWriteArrayList();
ContainerFactoryRegistry()
{
factories.add(new ListContainerFactory());
factories.add(new SetContainerFactory());
factories.add(new SortedSetContainerFactory());
factories.add(new UnwrappedSingleValueFactory());
}
ContainerFactoryRegistry(ContainerFactoryRegistry parent)
{
cache.putAll(parent.cache);
factories.addAll(parent.factories);
}
void register(ContainerFactory> factory)
{
factories.add(factory);
cache.clear();
}
public ContainerFactoryRegistry createChild()
{
return new ContainerFactoryRegistry(this);
}
public ContainerBuilder createBuilderFor(Class> type)
{
if (cache.containsKey(type)) {
return cache.get(type).newContainerBuilderFor(type);
}
for (int i = factories.size(); i > 0; i--) {
ContainerFactory factory = factories.get(i - 1);
if (factory.accepts(type)) {
cache.put(type, factory);
return factory.newContainerBuilderFor(type);
}
}
throw new IllegalStateException("No container builder available for " + type.getName());
}
static class SortedSetContainerFactory implements ContainerFactory> {
@Override
public boolean accepts(Class> type)
{
return type.equals(SortedSet.class);
}
@Override
public ContainerBuilder> newContainerBuilderFor(Class> type)
{
return new ContainerBuilder>()
{
private SortedSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy